The following code is for the code file namedpipe_p1.c. // This process writes first, then reads #include #include #include #include #include #include int main() { int fd; // FIFO file path char * myfifo = "/tmp/myfifo"; // Creating the named file(FIFO) // mkfifo(, ) mkfifo(myfifo, 0666); char arr1[80], arr2[80]; // Open FIFO for write only fd = open(myfifo, O_WRONLY); // Take an input arr2ing from user. // 80 is maximum length printf("your message: "); fgets(arr2, 80, stdin); // Write the input arr2ing on FIFO // and close it write(fd, arr2, strlen(arr2)+1); close(fd); // Open FIFO for Read only fd = open(myfifo, O_RDONLY); // Read from FIFO read(fd, arr1, sizeof(arr1)); // Print the read message printf("Received: %s\n", arr1); close(fd); return 0; } The following code is for another code file namedpipe_p2.c. // This program reads first, then writes #include #include #include #include #include #include int main() { int fd1; // FIFO file path char * myfifo = "/tmp/myfifo"; // Creating the named file(FIFO) // mkfifo(,) mkfifo(myfifo, 0666); char str1[80], str2[80]; // First open in read only and read fd1 = open(myfifo,O_RDONLY); read(fd1, str1, 80); // Print the read string and close printf("Received: %s\n", str1); close(fd1); // Now open in write mode and write // string taken from user. fd1 = open(myfifo,O_WRONLY); printf("your message: "); fgets(str2, 80, stdin); write(fd1, str2, strlen(str2)+1); close(fd1); return 0; } Compile the two files and run the files in two SSH terminals respectively. Hint: gcc sourcecode.c -o outputfile.out What is the output you have observed? Please paste the screenshots. Add a piece of code to each file so that (1) each process shows its pid using printf and (2) then sends its pid to another side using the named pipe. Hint: use getpid() as what you did in previous labs. What is your modified source code for namedpipe_p1.c only? What are the results on the two SSH terminal? Please paste the screenshots?
The following code is for the code file namedpipe_p1.c.
// This process writes first, then reads
#include <stdio.h>
#include <string.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
int main()
{
int fd;
// FIFO file path
char * myfifo = "/tmp/myfifo";
// Creating the named file(FIFO)
// mkfifo(<pathname>, <permission>)
mkfifo(myfifo, 0666);
char arr1[80], arr2[80];
// Open FIFO for write only
fd = open(myfifo, O_WRONLY);
// Take an input arr2ing from user.
// 80 is maximum length
printf("your message: ");
fgets(arr2, 80, stdin);
// Write the input arr2ing on FIFO
// and close it
write(fd, arr2, strlen(arr2)+1);
close(fd);
// Open FIFO for Read only
fd = open(myfifo, O_RDONLY);
// Read from FIFO
read(fd, arr1, sizeof(arr1));
// Print the read message
printf("Received: %s\n", arr1);
close(fd);
return 0;
}
The following code is for another code file namedpipe_p2.c.
// This program reads first, then writes
#include <stdio.h>
#include <string.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
int main()
{
int fd1;
// FIFO file path
char * myfifo = "/tmp/myfifo";
// Creating the named file(FIFO)
// mkfifo(<pathname>,<permission>)
mkfifo(myfifo, 0666);
char str1[80], str2[80];
// First open in read only and read
fd1 = open(myfifo,O_RDONLY);
read(fd1, str1, 80);
// Print the read string and close
printf("Received: %s\n", str1);
close(fd1);
// Now open in write mode and write
// string taken from user.
fd1 = open(myfifo,O_WRONLY);
printf("your message: ");
fgets(str2, 80, stdin);
write(fd1, str2, strlen(str2)+1);
close(fd1);
return 0;
}
Compile the two files and run the files in two SSH terminals respectively.
Hint: gcc sourcecode.c -o outputfile.out
What is the output you have observed? Please paste the screenshots.
Add a piece of code to each file so that (1) each process shows its pid using printf and (2) then sends its pid to another side using the named pipe. Hint: use getpid() as what you did in previous labs.
What is your modified source code for namedpipe_p1.c only?
What are the results on the two SSH terminal? Please paste the screenshots?

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 3 images

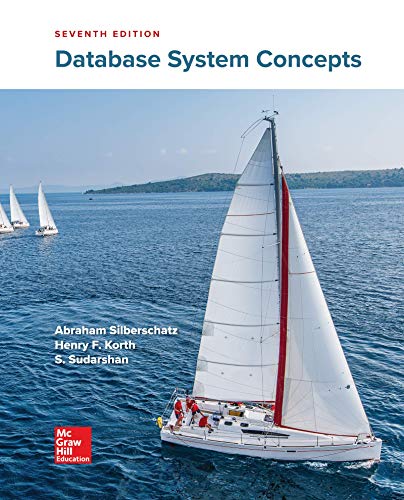
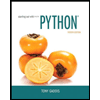
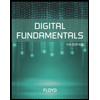
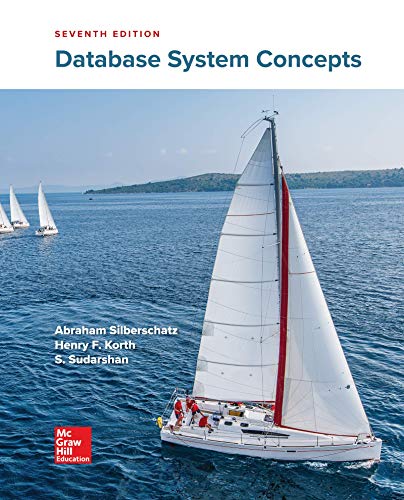
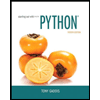
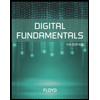
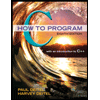
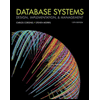
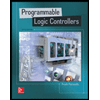