++ Please add the Decryption class. Read, using your FileReader, the PunchCard.txt file. Each Punch Card represents a matrix of 12 rows and 80 columns: -------------------------------------------------------------------------------- Y 00000000000000000000000000000000000000000000000000000000000000000000000000000000 X 00000000000000000000000000000000000000000000000000000000000000000000000000000000 0 10000000000000000000000000000000000000000000000000000000000000000000000000000000 1 01000000000000000000000000000000000000000000000000000000000000000000000000000000 2 00100000000000000000000000000000000000000000000000000000000000000000000000000000 3 00010000000000000000000000000000000000000000000000000000000000000000000000000000 4 00001000000000000000000000000000000000000000000000000000000000000000000000000000 5 00000100000000000000000000000000000000000000000000000000000000000000000000000000 6 00000010000000000000000000000000000000000000000000000000000000000000000000000000 7 00000001000000000000000000000000000000000000000000000000000000000000000000000000 8 00000000100000000000000000000000000000000000000000000000000000000000000000000000 9 00000000010000000000000000000000000000000000000000000000000000000000000000000000 -------------------------------------------------------------------------------- Each card in the data file is seperated by dashed lines. The matrix is 12x80. To read the file, read a line, store the 80 characters in a string and store the string in a Translation vector: vector translate. While the line-count is less than 13, read and translate the next line. Process the in-memory matrix and then read the next card. To Process the matrix, read the rows by the 80 columns. Each complete column is a EBDIC character. Lookup the EBDIC character in the tupleVector and find the ASCII character in the found tuple. Repeat for each character in the file. The end result is English readable text. Design a Decryption class to model the decryption process as outlined in step 3. Your Decryption class needs a Process function CallBack, as each encrypted file is encrypted differently. The class should also contain a vector for the translated strings. Determine which member functions are appropriate for decryption behavior. To answer that question, ask yourself: "What methods would you expect from a Decryption class?" #include #include #include #include #include #include #include #include using namespace std; // Define a struct to represent a tuple template struct MyTuple { T1 field1; T2 field2; T3 field3; }; // Define a regex callback function for parsing binary files string RemoveSpace(string s) { regex nonSpace(" "); return regex_replace(s, nonSpace, ""); } int main() { // Read the tuple file ifstream tupleFile("Tuple.csv"); string line; vector> tupleVector; while (getline(tupleFile, line)) { // Split the line into three parts separated by commas size_t comma1 = line.find(","); size_t comma2 = line.find(",", comma1+1); string field1 = line.substr(0, comma1); string field2 = line.substr(comma1+1, comma2-comma1-1); char field3 = line[comma2+1]; // Create a tuple from the three fields and add it to the vector tupleVector.push_back(MyTuple{field1, field2, field3}); } // Read the punch card file ifstream punchCardFile("PunchCards.txt"); vector translate; while (getline(punchCardFile, line)) { // Skip any lines that start with dashes if (line.substr(0, 1) == "-") { continue; } // Read the next 12 lines and store them in a matrix vector> matrix(12, vector(80)); for (int i = 0; i < 12; i++) { getline(punchCardFile, line); for (int j = 0; j < 80; j++) { matrix[i][j] = line[j]; } } // Process the matrix by looking up each EBDIC character in the tuple vector string translated; for (int j = 0; j < 80; j++) { string ebdic; for (int i = 0; i < 12; i++) { ebdic += matrix[i][j]; } auto it = find_if(tupleVector.begin(), tupleVector.end(), [&ebdic](const auto& t){ return get<1>(t) == ebdic; }); if (it != tupleVector.end()) { translated += get<2>(*it); / It has an error here when I compile the code. } } translate.push_back(translated); } }
C++
Please add the Decryption class.
Read, using your FileReader, the PunchCard.txt file. Each Punch Card represents a matrix of 12 rows and 80 columns:
--------------------------------------------------------------------------------
Y 00000000000000000000000000000000000000000000000000000000000000000000000000000000
X 00000000000000000000000000000000000000000000000000000000000000000000000000000000
0 10000000000000000000000000000000000000000000000000000000000000000000000000000000
1 01000000000000000000000000000000000000000000000000000000000000000000000000000000
2 00100000000000000000000000000000000000000000000000000000000000000000000000000000
3 00010000000000000000000000000000000000000000000000000000000000000000000000000000
4 00001000000000000000000000000000000000000000000000000000000000000000000000000000
5 00000100000000000000000000000000000000000000000000000000000000000000000000000000
6 00000010000000000000000000000000000000000000000000000000000000000000000000000000
7 00000001000000000000000000000000000000000000000000000000000000000000000000000000
8 00000000100000000000000000000000000000000000000000000000000000000000000000000000
9 00000000010000000000000000000000000000000000000000000000000000000000000000000000
--------------------------------------------------------------------------------
Each card in the data file is seperated by dashed lines. The matrix is 12x80. To read the file, read a line, store the 80 characters in a string and store the string in a Translation
To Process the matrix, read the rows by the 80 columns. Each complete column is a EBDIC character. Lookup the EBDIC character in the tupleVector and find the ASCII character in the found tuple. Repeat for each character in the file. The end result is English readable text.
Design a Decryption class to model the decryption process as outlined in step 3. Your Decryption class needs a Process function CallBack, as each encrypted file is encrypted differently. The class should also contain a vector<string> for the translated strings. Determine which member functions are appropriate for decryption behavior. To answer that question, ask yourself: "What methods would you expect from a Decryption class?"
#include <iostream>
#include <fstream>
#include <string>
#include <functional>
#include <vector>
#include <tuple>
#include <regex>
#include <algorithm>
using namespace std;
// Define a struct to represent a tuple
template <typename T1, typename T2, typename T3>
struct MyTuple {
T1 field1;
T2 field2;
T3 field3;
};
// Define a regex callback function for parsing binary files
string RemoveSpace(string s) {
regex nonSpace(" ");
return regex_replace(s, nonSpace, "");
}
int main() {
// Read the tuple file
ifstream tupleFile("Tuple.csv");
string line;
vector<MyTuple<string, string, char>> tupleVector;
while (getline(tupleFile, line)) {
// Split the line into three parts separated by commas
size_t comma1 = line.find(",");
size_t comma2 = line.find(",", comma1+1);
string field1 = line.substr(0, comma1);
string field2 = line.substr(comma1+1, comma2-comma1-1);
char field3 = line[comma2+1];
// Create a tuple from the three fields and add it to the vector
tupleVector.push_back(MyTuple<string, string, char>{field1, field2, field3});
}
// Read the punch card file
ifstream punchCardFile("PunchCards.txt");
vector<string> translate;
while (getline(punchCardFile, line)) {
// Skip any lines that start with dashes
if (line.substr(0, 1) == "-") {
continue;
}
// Read the next 12 lines and store them in a matrix
vector<vector<char>> matrix(12, vector<char>(80));
for (int i = 0; i < 12; i++) {
getline(punchCardFile, line);
for (int j = 0; j < 80; j++) {
matrix[i][j] = line[j];
}
}
// Process the matrix by looking up each EBDIC character in the tuple vector
string translated;
for (int j = 0; j < 80; j++) {
string ebdic;
for (int i = 0; i < 12; i++) {
ebdic += matrix[i][j];
}
auto it = find_if(tupleVector.begin(), tupleVector.end(), [&ebdic](const auto& t){ return get<1>(t) == ebdic; });
if (it != tupleVector.end()) {
translated += get<2>(*it); / It has an error here when I compile the code.
}
}
translate.push_back(translated);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

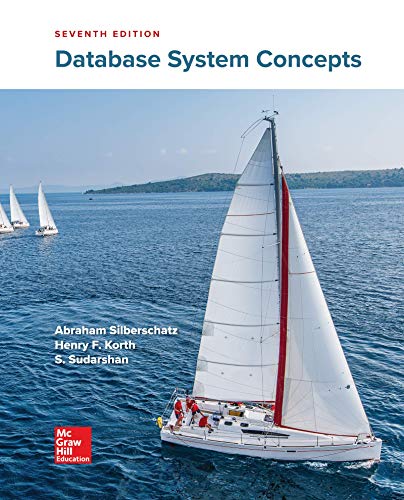
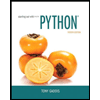
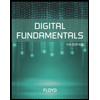
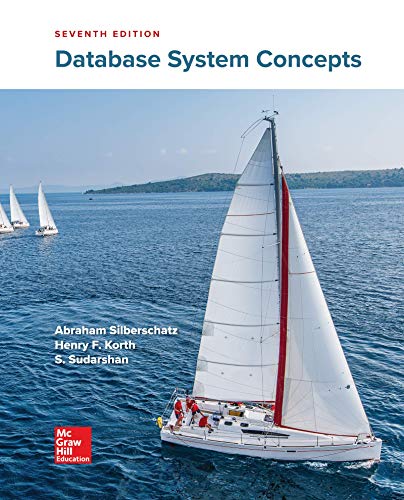
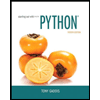
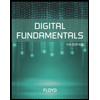
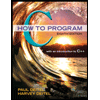
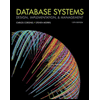
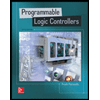