Using polymorphism and object-oriented programming Design an Elevator simulation. The simulation have 4 different types of elevators and passengers. There are 4 types of passengers in the system: Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements. VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators. Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators. Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. There are 4 types of elevators in the system: StandardElevator: This is the most common type of elevator and has a request percentage of 70%. Standard elevators have a maximum capacity of 10 passengers. ExpressElevator: This type of elevator has a request percentage of 20%. Express elevators have a maximum capacity of 8 passengers and are faster than standard elevators. FreightElevator: This type of elevator has a request percentage of 5%. Freight elevators have a maximum capacity of 5 passengers and are designed to transport heavy items. GlassElevator: This type of elevator has a request percentage of 5%. Glass elevators have a maximum capacity of 6 passengers and are designed to transport fragile item The input should look like this: # Building parameters floors=30 # Passengers to add to floors add_passenger 1 6 25 Standard 30 add_passenger 2 2 28 VIP 10 add_passenger 3 7 15 Freight 20 add_passenger 4 4 20 Glass 15 # Elevator types elevator_type StandardElevator 10 40 elevator_type ExpressElevator 8 25 elevator_type FreightElevator 5 20 elevator_type GlassElevator 6 15 # Percentage of passenger requests for each elevator type request_percentage StandardElevator 70 request_percentage ExpressElevator 20 request_percentage FreightElevator 5 request_percentage GlassElevator 5 # Percentage of passenger requests for each passenger type passenger_request_percentage Standard 70 passenger_request_percentage VIP 10 passenger_request_percentage Freight 15 passenger_request_percentage Glass 5 # Number of elevators in the system number_of_elevators 8 # Run simulation for 60 iterations run_simulation 60 Use these classes to make the elevator simulation: Main class: public class SimulatorSettings { SimulatorSettings(String fileName){ } private int nofloors; public int getNofloors() { return nofloors; } public void setNofloors(int nofloors) { this.nofloors = nofloors; } } Other Classes: public class Simulations { while(scanner.hasNextLine()){ String line = scanner.nextLine(); if(line.startsWith("floor=")) { line= line.replace("floor=", ""); // Convert the value to the wanted type System.out.println(line); } } settings.setNofloors(55); Passenger pass1 = new StandardPassenger(); pass1.requestElevator(settings); ArrayList passengers = null; for(int i = 0; i < 100; i++){ //Use the percentage from the file passengers.add(new StandardPassenger()); } } } public abstract class Passenger { public static int passangerCounter = 0; private String passengerID; protected int startFloor; protected int endFloor; Passenger(){ this.passengerID = ""+passangerCounter; passangerCounter++; } public abstract boolean requestElevator(SimulatorSettings settings); } public class StandardPassenger extends Passenger{ private String type; public StandardPassenger() { } public boolean requestElevator(SimulatorSettings settings){ Random rand = new Random(); this.startFloor = rand.nextInt()% settings.getNofloors(); this.endFloor = rand.nextInt() % settings.getNofloors(); while(this.startFloor == this.endFloor){ this.endFloor = rand.nextInt() % settings.getNofloors(); } return true; } } And make Other 3 passengers classes that use polymorphism. public class Elevator { private int currentFloor = 0; private int maxFloor; public int getCurrentFloor() { return currentFloor; } public int getMaxFloor() { return maxFloor; } public void setCurrentFloor(int currentFloor) { this.currentFloor = currentFloor; } public void setMaxFloor(int maxFloor) { this.maxFloor = maxFloor; } } And make Other 4 elevator classes that use polymorphism.
Using polymorphism and object-oriented programming Design an Elevator simulation. The simulation have 4 different types of elevators and passengers.
There are 4 types of passengers in the system:
Standard: This is the most common type of passenger and has a request percentage of 70%.
Standard passengers have no special requirements.
VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority
and are more likely to be picked up by express elevators.
Freight: This type of passenger has a request percentage of 15%. Freight passengers have large
items that need to be transported and are more likely to be picked up by freight elevators.
Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items
that need to be transported and are more likely to be picked up by glass elevators.
There are 4 types of elevators in the system:
StandardElevator: This is the most common type of elevator and has a request percentage of 70%.
Standard elevators have a maximum capacity of 10 passengers.
ExpressElevator: This type of elevator has a request percentage of 20%. Express elevators have a
maximum capacity of 8 passengers and are faster than standard elevators.
FreightElevator: This type of elevator has a request percentage of 5%. Freight elevators have a
maximum capacity of 5 passengers and are designed to transport heavy items.
GlassElevator: This type of elevator has a request percentage of 5%. Glass elevators have a
maximum capacity of 6 passengers and are designed to transport fragile item
The input should look like this:
# Building parameters
floors=30
# Passengers to add to floors
add_passenger 1 6 25 Standard 30
add_passenger 2 2 28 VIP 10
add_passenger 3 7 15 Freight 20
add_passenger 4 4 20 Glass 15
# Elevator types
elevator_type StandardElevator 10 40
elevator_type ExpressElevator 8 25
elevator_type FreightElevator 5 20
elevator_type GlassElevator 6 15
# Percentage of passenger requests for each elevator type
request_percentage StandardElevator 70
request_percentage ExpressElevator 20
request_percentage FreightElevator 5
request_percentage GlassElevator 5
# Percentage of passenger requests for each passenger type
passenger_request_percentage Standard 70
passenger_request_percentage VIP 10
passenger_request_percentage Freight 15
passenger_request_percentage Glass 5
# Number of elevators in the system
number_of_elevators 8
# Run simulation for 60 iterations
run_simulation 60
Use these classes to make the elevator simulation:
Main class:
public class SimulatorSettings {
SimulatorSettings(String fileName){
}
private int nofloors;
public int getNofloors() {
return nofloors;
}
public void setNofloors(int nofloors) {
this.nofloors = nofloors;
}
}
Other Classes:
public class Simulations {
while(scanner.hasNextLine()){
String line = scanner.nextLine();
if(line.startsWith("floor="))
{
line= line.replace("floor=", "");
// Convert the value to the wanted type
System.out.println(line);
}
}
settings.setNofloors(55);
Passenger pass1 = new StandardPassenger();
pass1.requestElevator(settings);
ArrayList<Passenger> passengers = null;
for(int i = 0; i < 100; i++){
//Use the percentage from the file
passengers.add(new StandardPassenger());
}
}
}
public abstract class Passenger {
public static int passangerCounter = 0;
private String passengerID;
protected int startFloor;
protected int endFloor;
Passenger(){
this.passengerID = ""+passangerCounter;
passangerCounter++;
}
public abstract boolean requestElevator(SimulatorSettings settings);
}
public class StandardPassenger extends Passenger{
private String type;
public StandardPassenger() {
}
public boolean requestElevator(SimulatorSettings settings){
Random rand = new Random();
this.startFloor = rand.nextInt()% settings.getNofloors();
this.endFloor = rand.nextInt() % settings.getNofloors();
while(this.startFloor == this.endFloor){
this.endFloor = rand.nextInt() % settings.getNofloors();
}
return true;
}
}
And make Other 3 passengers classes that use polymorphism.
public class Elevator {
private int currentFloor = 0;
private int maxFloor;
public int getCurrentFloor() {
return currentFloor;
}
public int getMaxFloor() {
return maxFloor;
}
public void setCurrentFloor(int currentFloor) {
this.currentFloor = currentFloor;
}
public void setMaxFloor(int maxFloor) {
this.maxFloor = maxFloor;
}
}
And make Other 4 elevator classes that use polymorphism.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

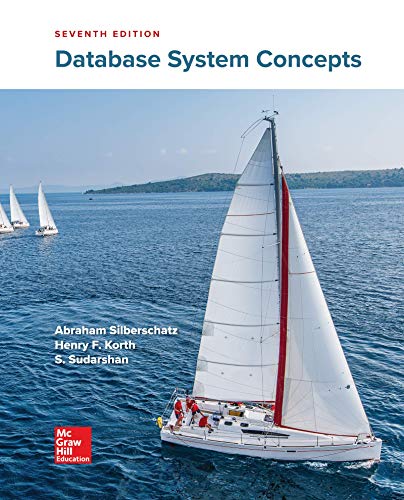
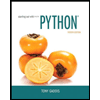
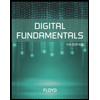
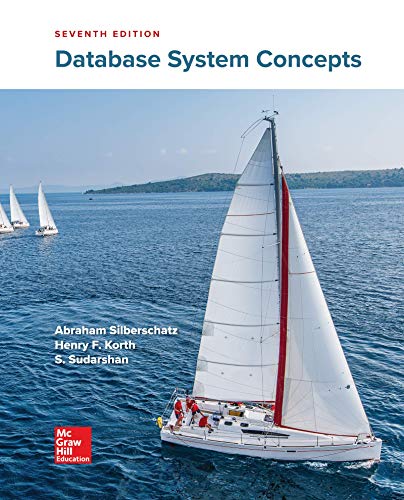
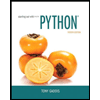
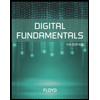
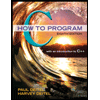
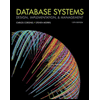
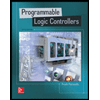