I need help in improving the Simulator setting class and Simulation class. I posted the requirement for both classes down below. This is an elevator simulator that uses polymorphism and object-oriented programming to simulate the movement of elevators in a building with multiple types of passengers and elevators. The elevator simulator that uses polymorphism and object-oriented programming to simulate the movement of elevators in a building with multiple types of passengers and elevators. The system has 8 elevators, each of which can be one of 4 types of elevators, with a certain percentage of requests for each type. Similarly, each passenger can be one of 4 types, with a different percentage of requests for each type. Classes requirements: Simulator Settings{ Houses setters/getters Holds variables used for the program and information taken from settings.txt to run the program } Simulation{ Initializes the simulation Creates Arraylist of Passengers, Elevator, Floors Holds the process of Elevators } My code: import java.io.File; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Random; import java.util.Scanner; public class Simulation { SimulatorSettings settings; public void initSimulation(SimulatorSettings settings) throws FileNotFoundException { Passenger.passengerCounter = 0; this.settings = settings; File file = new File("settings.txt"); Scanner scanner = new Scanner(file); while (scanner.hasNextLine()) { String line = scanner.nextLine(); if (line.startsWith("floor=")) { line = line.replace("floor=", ""); settings.setNofloors(Integer.parseInt(line)); } } ArrayList passengers = new ArrayList<>(); for (int i = 0; i < 100; i++) { // Use the percentage from the file passengers.add(new StandardPassenger()); } } } public class SimulatorSettings { SimulatorSettings(String fileName){ } private int nofloors; public int getNofloors() { return nofloors; } public void setNofloors(int nofloors) { this.nofloors = nofloors; } } import java.util.ArrayList; public class Building { private int totalFloors; private int totalElevators; private ArrayList elevators; private ArrayList floors; public Building(int totalFloors, int totalElevators) { this.totalFloors = totalFloors; this.totalElevators = totalElevators; elevators = new ArrayList(); floors = new ArrayList(); for (int i = 0; i < totalFloors; i++) { floors.add(new Floor()); } for (int i = 0; i < totalElevators; i++) { // Use a method to randomly generate the type of elevator // based on the given percentages Elevator elevator = generateRandomElevator(); elevators.add(elevator); elevator.setId(i); } } public int getTotalFloors() { return totalFloors; } public int getTotalElevators() { return totalElevators; } public ArrayList getElevators() { return elevators; } public ArrayList getFloors() { return floors; } private Elevator generateRandomElevator() { // Use a method to randomly generate the type of elevator // based on the given percentages // This method returns an instance of one of the four types of Elevator classes return new ElevatorType1(); // Example: Always return ElevatorType1 for now } } public class Floor { private ArrayList floorPass; public Floor() { floorPass = new ArrayList(); } public void addPassenger(Passenger passenger) { floorPass.add(passenger); } public ArrayList getPassengers() { return floorPass; } } abstract class Passenger { private static int passengerCounter = 0; private String passengerID; protected int startFloor; protected int endFloor; protected static Random rand = new Random(); public Passenger() { this.passengerID = String.valueOf(passengerCounter); passengerCounter++; } public abstract boolean requestElevator(SimulatorSettings settings); } public abstract class Elevator{ private int currentFloor; private int maxCapacity; private int passengersCount; public Elevator(int maxCapacity) { this.currentFloor = 0; // assuming initial floor is 0 this.maxCapacity = maxCapacity; this.passengersCount = 0; } public abstract void moveUp(); public abstract void moveDown(); public abstract void addPassenger(Passenger passenger); public abstract void removePassenger(Passenger passenger); }
I need help in improving the Simulator setting class and Simulation class. I posted the requirement for both classes down below. This is an elevator simulator that uses polymorphism and object-oriented programming to simulate the movement of elevators in a building with multiple types of passengers and elevators.
The elevator simulator that uses polymorphism and object-oriented programming to
simulate the movement of elevators in a building with multiple types of passengers and elevators.
The system has 8 elevators, each of which can be one of 4 types of elevators, with a certain
percentage of requests for each type. Similarly, each passenger can be one of 4 types, with a
different percentage of requests for each type.
Classes requirements:
Simulator Settings{
Houses setters/getters
Holds variables used for the program
and information taken from settings.txt to run the program
}
Simulation{
Initializes the simulation
Creates Arraylist of Passengers, Elevator, Floors
Holds the process of Elevators
}
My code:
import java.io.File;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Random;
import java.util.Scanner;
public class Simulation {
SimulatorSettings settings;
public void initSimulation(SimulatorSettings settings) throws FileNotFoundException {
Passenger.passengerCounter = 0;
this.settings = settings;
File file = new File("settings.txt");
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
if (line.startsWith("floor=")) {
line = line.replace("floor=", "");
settings.setNofloors(Integer.parseInt(line));
}
}
ArrayList<Passenger> passengers = new ArrayList<>();
for (int i = 0; i < 100; i++) {
// Use the percentage from the file
passengers.add(new StandardPassenger());
}
}
}
public class SimulatorSettings {
SimulatorSettings(String fileName){
}
private int nofloors;
public int getNofloors() {
return nofloors;
}
public void setNofloors(int nofloors) {
this.nofloors = nofloors;
}
}
import java.util.ArrayList;
public class Building {
private int totalFloors;
private int totalElevators;
private ArrayList<Elevator> elevators;
private ArrayList<Floor> floors;
public Building(int totalFloors, int totalElevators) {
this.totalFloors = totalFloors;
this.totalElevators = totalElevators;
elevators = new ArrayList<Elevator>();
floors = new ArrayList<Floor>();
for (int i = 0; i < totalFloors; i++) {
floors.add(new Floor());
}
for (int i = 0; i < totalElevators; i++) {
// Use a method to randomly generate the type of elevator
// based on the given percentages
Elevator elevator = generateRandomElevator();
elevators.add(elevator);
elevator.setId(i);
}
}
public int getTotalFloors() {
return totalFloors;
}
public int getTotalElevators() {
return totalElevators;
}
public ArrayList<Elevator> getElevators() {
return elevators;
}
public ArrayList<Floor> getFloors() {
return floors;
}
private Elevator generateRandomElevator() {
// Use a method to randomly generate the type of elevator
// based on the given percentages
// This method returns an instance of one of the four types of Elevator classes
return new ElevatorType1(); // Example: Always return ElevatorType1 for now
}
}
public class Floor {
private ArrayList<Passenger> floorPass;
public Floor() {
floorPass = new ArrayList<Passenger>();
}
public void addPassenger(Passenger passenger) {
floorPass.add(passenger);
}
public ArrayList<Passenger> getPassengers() {
return floorPass;
}
}
abstract class Passenger {
private static int passengerCounter = 0;
private String passengerID;
protected int startFloor;
protected int endFloor;
protected static Random rand = new Random();
public Passenger() {
this.passengerID = String.valueOf(passengerCounter);
passengerCounter++;
}
public abstract boolean requestElevator(SimulatorSettings settings);
}
public abstract class Elevator{
private int currentFloor;
private int maxCapacity;
private int passengersCount;
public Elevator(int maxCapacity) {
this.currentFloor = 0; // assuming initial floor is 0
this.maxCapacity = maxCapacity;
this.passengersCount = 0;
}
public abstract void moveUp();
public abstract void moveDown();
public abstract void addPassenger(Passenger passenger);
public abstract void removePassenger(Passenger passenger);
}

Step by step
Solved in 3 steps

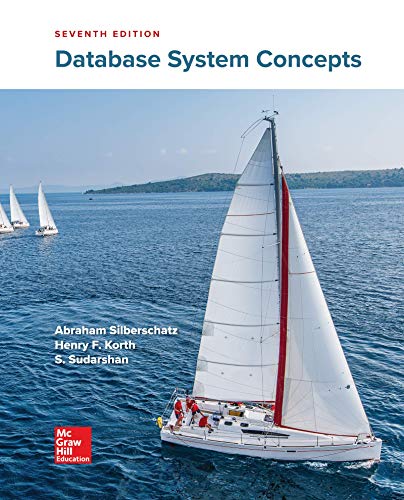
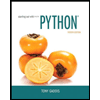
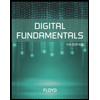
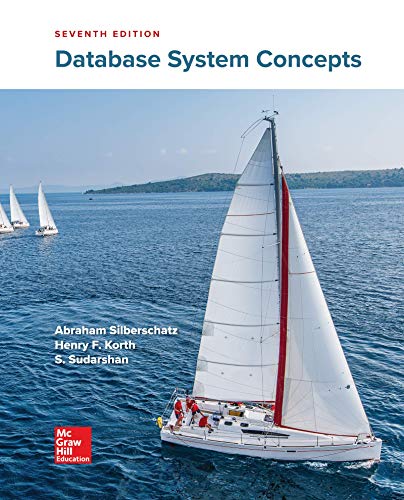
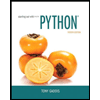
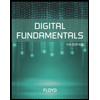
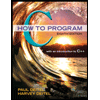
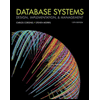
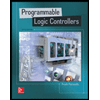