We have an interface called discount strategy, that calculates discounts for a simple parking management software. It has two sub-classes that implement different discount strategies. 1. DUAffilatedFriday discounts - make discounts for DUAffilated car type on Fridays 2. SUVFriday discounts - make discounts for SUV car type on Fridays These two classes check the car type and day of the week and implement a certain discount strategy. 1. Use a factory pattern to reimplement the entire code as-is. 2. Use a main method to test your code
We have an interface called discount strategy, that calculates discounts for a simple parking management software. It has two sub-classes that implement different discount strategies.
1. DUAffilatedFriday discounts - make discounts for DUAffilated car type on Fridays
2. SUVFriday discounts - make discounts for SUV car type on Fridays
These two classes check the car type and day of the week and implement a certain discount strategy.
1. Use a factory pattern to reimplement the entire code as-is.
2. Use a main method to test your code
NOte - Use java to write your code
Code
DiscountStrategy.java
import java.time.*;
interface DiscountStrategy{
public String getStrategyName();
public double getDiscount(String CarType, LocalDateTime date);
}
DUAffilatedFridayDiscounts.java
import java.time.*;
public class DUAffilatedFridayDiscounts implements DiscountStrategy{
// setting default value of discount to 0.0
private double discountPercentage = 0.0;
private boolean checkDate(LocalDateTime date){
return "Friday".equals(date.getDayOfWeek().toString());
}
private boolean checkCarType(String CarType){
return CarType.compareTo("DuAffilated") == 0;
}
@Override
public String getStrategyName(){
return "DUAffilatedFridayDiscounts";
}
@Override
public double getDiscount(String CarType, LocalDateTime date){
if(checkDate(date) && checkCarType(CarType))
discountPercentage= 5.6;
return discountPercentage;
}
}
SUVFridayDiscounts.java
import java.time.*;
public class SUVFridayDiscounts implements DiscountStrategy{
// setting default value of discount to 0.0
private double discountPercentage = 0.0;
private boolean checkDate(LocalDateTime date){
return "Friday".equals(date.getDayOfWeek().toString());
}
private boolean checkCarType(String CarType){
return CarType.compareTo("SUV") == 0;
}
@Override
public String getStrategyName(){
return "DUAffilatedFridayDiscounts";
}
@Override
public double getDiscount(String CarType, LocalDateTime date){
if(checkDate(date) && checkCarType(CarType))
discountPercentage= 10.5;
return discountPercentage;
}
}

Step by step
Solved in 2 steps

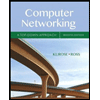
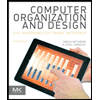
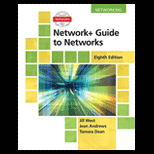
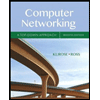
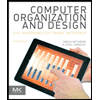
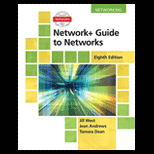
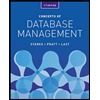
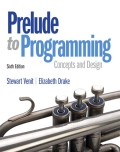
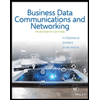