Use the following code to allow an output to the screen to play a word descrambling game in C++
Use the following code to allow an output to the screen to play a word descrambling game in C++
#include <bits/stdc++.h>
using namespace std;
// Function that sorts the given string
// and transform a sorted string to uppercase
string sortString(string word)
{
// Transformed to uppercase
transform(word.begin(), word.end(),
word.begin(), ::toupper);
// Sort the words
sort(word.begin(), word.end());
return word;
}
// Function that finds the anagram of
// given string in the given text file
void jumbledString(string jumble)
{
// Initialize strings
string checkPerWord = "";
string userEnteredAfterSorting;
// Sort the string
userEnteredAfterSorting
= sortString(jumble);
// Using filehandling ifstream
// to read the file
ifstream words("words.txt");
// If file exist
if (words) {
// Check each and every word
// of words.txt(dictionary)
while (getline(words,
checkPerWord)) {
string Ch
= sortString(checkPerWord);
// If words matches
if (Ch
== userEnteredAfterSorting) {
// Print the word
cout << checkPerWord
<< endl;
}
}
// Close the file
words.close();
}
}
// Driver Code
int main()
{
// Given string str
string string = "tac";
// Function Call
jumbledString(string);
return 0;
}

There are some issues with the code. Also without the contents of the text file, it is difficult to tell the error.
- There is an issue with a copy elision.
- In the code "words" is the text file and "word" is a variable used.
- Use fstream in the header to read and write files easily
- The file "words.txt" should be in the working directory. Usually, the working directory is the directory containing the executable
------------------------------------------------------ Updated C++ Code ------------------------------------------------
#include <iostream>
#include <fstream>
using namespace std;
string sortString(string word)
{
transform(word.begin(), word.end(), word.begin(), ::toupper);
sort(word.begin(), word.end());
return word;
}
void jumbledString(string jumble)
{
string checkPerWord = "";
string userEnteredAfterSorting;
userEnteredAfterSorting = sortString(jumble);
ifstream word("words.txt");
if (word) {
while (getline(word, checkPerWord)) {
string Ch = sortString(checkPerWord);
if (Ch == userEnteredAfterSorting) {
cout << checkPerWord << endl;
}
}
word.close();
}
}
int main()
{
string string = "tac";
jumbledString(string);
return 0;
}
Step by step
Solved in 2 steps

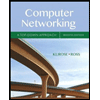
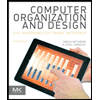
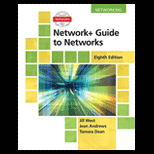
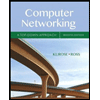
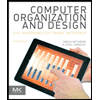
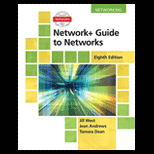
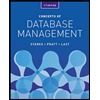
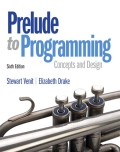
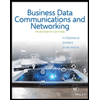