this code: #include #include using namespace std; // Node struct to store data for each song in the playlist struct Node { string data; Node * next; }; // Function to create a new node and return its address Node * getNewNode(string song) { Node * newNode = new Node();
In this code:
#include <iostream>
#include <string>
using namespace std;
// Node struct to store data for each song in the playlist
struct Node
{
string data;
Node * next;
};
// Function to create a new node and return its address
Node * getNewNode(string song)
{
Node * newNode = new Node();
newNode -> data = song;
newNode -> next = NULL;
return newNode;
}
// Function to insert a new node at the head of the linked list
void insertAtHead(Node ** head, string song)
{
Node * newNode = getNewNode(song);
if ( * head == NULL) {
* head = newNode;
return;
}
newNode -> next = * head;
* head = newNode;
}
// Function to insert a new node at the tail of the linked list
void insertAtTail(Node ** head, string song)
{
Node * newNode = getNewNode(song);
if ( * head == NULL) {
* head = newNode;
return;
}
Node * temp = * head;
while (temp -> next != NULL)
{
temp = temp -> next;
}
temp -> next = newNode;
}
// Function to remove a node from the linked list
void removeNode(Node ** head, string song)
{
if ( * head == NULL) {
return;
}
Node * temp = * head;
// If the song to be removed is at the head
if (temp != NULL && temp -> data == song) {
* head = temp -> next;
free(temp);
return;
}
// If the song to be removed is not at the head
while (temp -> next != NULL) {
if (temp -> next -> data == song) {
break;
}
temp = temp -> next;
}
// If the song was not present in the linked list
if (temp -> next == NULL) {
return;
}
// Unlink the node from the linked list
Node * next = temp -> next -> next;
free(temp -> next);
temp -> next = next;
}
// Function to print the contents of the linked list
void printList(Node * head) {
while (head != NULL) {
cout << head -> data << " ";
head = head -> next;
}
cout << endl;
}
// Function to play the songs in the playlist in a loop
void playSongs(Node * head) {
if (head == NULL) {
cout << "Playlist is empty!" << endl;
return;
}
cout << "Playing songs in the playlist:" << endl;
Node * temp = head;
while (temp != NULL) {
cout << temp -> data << endl;
temp = temp -> next;
}
}
int main() {
Node * head = NULL;
insertAtHead( & head, "Song1");
insertAtHead( & head, "Song2");
insertAtTail( & head, "Song3");
insertAtTail( & head, "Song4");
removeNode( & head, "Song2");
printList(head);
playSongs(head);
return 0;
}
how to put a traversing the playlist and allow for common operations on a playlist such as: insert, remove, next, previous, play all songs

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

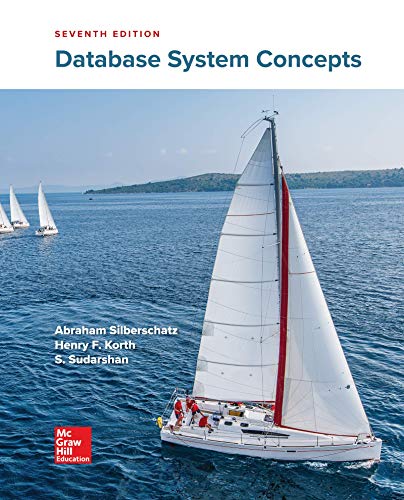
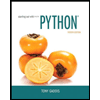
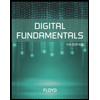
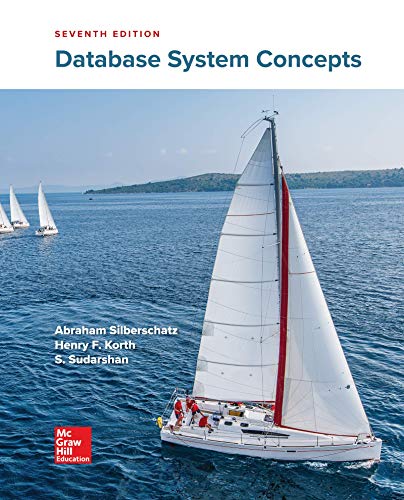
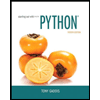
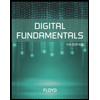
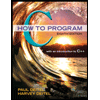
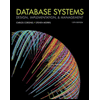
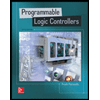