Write a user defined function named as “length_list” function that finds the length of a list of list_node_t nodes. This code should fit with the following code. Partial Code: #include #include /* gives access to malloc */ #define SENT -1 typedef struct list_node_s { int digit; struct list_node_s *restp; } list_node_t; list_node_t *get_list(void); int main(void) { list_node_t *x; int y; x=get_list(); printf("%d\n",x->digit); printf("%d\n",x->restp->digit); printf("%d\n",x->restp->restp->digit); // your length_list function should be called from here printf("Length is: %d",y); } /* * Forms a linked list of an input list of integers * terminated by SENT */ list_node_t * get_list(void) { int data; list_node_t *ansp; [20] scanf("%d", &data); if (data == SENT) { ansp = NULL; } else { ansp = (list_node_t *)malloc(sizeof (list_node_t)); ansp->digit = data; ansp->restp = get_list(); } return (ansp);
Write a user defined function named as “length_list” function that finds the length of a list
of list_node_t nodes. This code should fit with the following code.
Partial Code:
#include <stdio.h>
#include <stdlib.h> /* gives access to malloc */
#define SENT -1
typedef struct list_node_s {
int digit;
struct list_node_s *restp;
} list_node_t;
list_node_t *get_list(void);
int
main(void)
{
list_node_t *x;
int y;
x=get_list();
printf("%d\n",x->digit);
printf("%d\n",x->restp->digit);
printf("%d\n",x->restp->restp->digit);
// your length_list function should be called from here
printf("Length is: %d",y);
}
/*
* Forms a linked list of an input list of integers
* terminated by SENT
*/
list_node_t *
get_list(void)
{
int data;
list_node_t *ansp;
[20]
scanf("%d", &data);
if (data == SENT) {
ansp = NULL;
} else {
ansp = (list_node_t *)malloc(sizeof (list_node_t));
ansp->digit = data;
ansp->restp = get_list();
}
return (ansp);

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

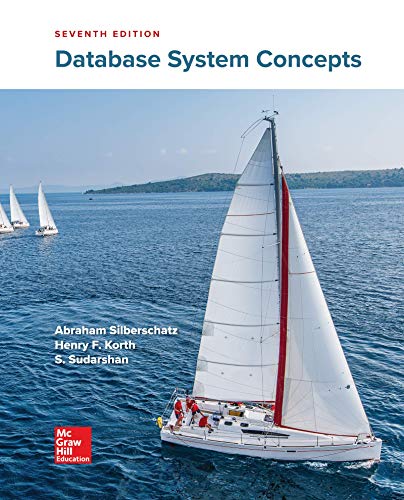
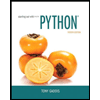
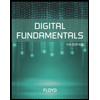
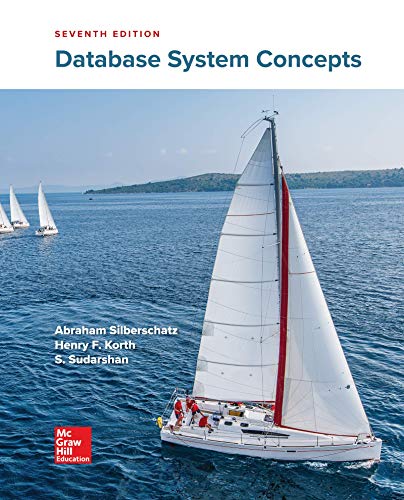
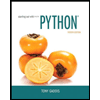
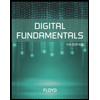
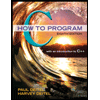
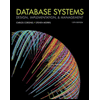
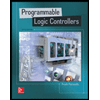