This assignment requires you to create a dictionary by reading the text file created for the Chapter 6 assignment. The dictionary should have player names for the keys. The value for each key must be a two-element list holding the player's goals and assists, respectively. See page 472! Start with an empty dictionary. Then, use a loop to cycle through the text file and add key-value pairs to the dictionary. Close the text file and process the dictionary to print the stats and determine the top scorer as before. In fact, much of the code used in program6_2.py can be copied and used for this program. Printing the stats for each player is the most challenging part of this program. To master this, refer to the examples in the zip file that can be downloaded from the "Dictionary values can be lists" link in the "Learn Here" part of this module. NOTE: you do not need to submit the text file. Submit just this program. The required output should be the same well-formatted table as was generated by program_62.py. PLAYER COLUMN: 10 characters wide and left-aligned. GOALS COLUMN: 6 characters wide and centered. ASSISTS COLUMN: 8 characters wide and centered. TOTAL COLUMN: 6 characters wide and centered. This is for Python, COP 1000. I have to use def main and I have program 6_2 listed below def main(): scores = open('text.txt','r') name = scores.readline() goals_assists = 0 print(f'{"Name":<10} {"Goals":^6} {"Assists":^8} {"Overall":^6}') while name != '': name = name.rstrip('\n') goals = int(scores.readline()) assists = int(scores.readline()) overall = goals + assists if overall > goals_assists: top_scorer = name goals_assists = overall print(f'{name:<10}{goals:^6}{assists:^8}{overall:^6}') name = scores.readline() scores.close() print(f'{top_scorer} is the top scorer with {goals_assists}') if __name__ == '__main__': main()
program9_1.py
This assignment requires you to create a dictionary by reading the text file created for the Chapter 6 assignment. The dictionary should have player names for the keys. The value for each key must be a two-element list holding the player's goals and assists, respectively. See page 472! Start with an empty dictionary. Then, use a loop to cycle through the text file and add key-value pairs to the dictionary. Close the text file and process the dictionary to print the stats and determine the top scorer as before. In fact, much of the code used in program6_2.py can be copied and used for this program. Printing the stats for each player is the most challenging part of this program. To master this, refer to the examples in the zip file that can be downloaded from the "Dictionary values can be lists" link in the "Learn Here" part of this module. NOTE: you do not need to submit the text file. Submit just this program. The required output should be the same well-formatted table as was generated by program_62.py.
- PLAYER COLUMN: 10 characters wide and left-aligned.
- GOALS COLUMN: 6 characters wide and centered.
- ASSISTS COLUMN: 8 characters wide and centered.
- TOTAL COLUMN: 6 characters wide and centered.
This is for Python, COP 1000. I have to use def main and I have program 6_2 listed below
def main():
scores = open('text.txt','r')
name = scores.readline()
goals_assists = 0
print(f'{"Name":<10} {"Goals":^6} {"Assists":^8} {"Overall":^6}')
while name != '':
name = name.rstrip('\n')
goals = int(scores.readline())
assists = int(scores.readline())
overall = goals + assists
if overall > goals_assists:
top_scorer = name
goals_assists = overall
print(f'{name:<10}{goals:^6}{assists:^8}{overall:^6}')
name = scores.readline()
scores.close()
print(f'{top_scorer} is the top scorer with {goals_assists}')
if __name__ == '__main__':
main()

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

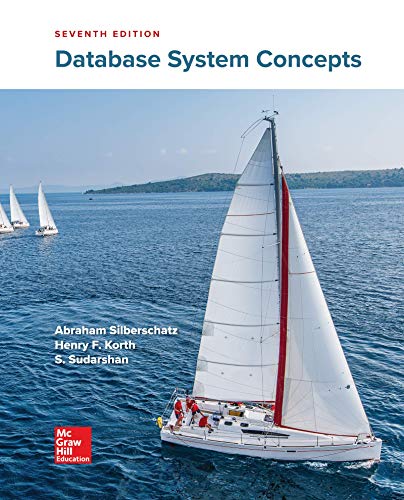
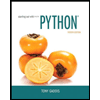
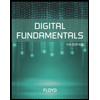
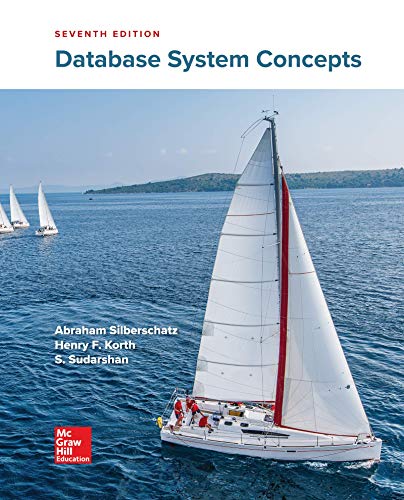
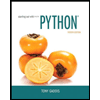
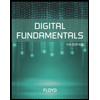
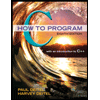
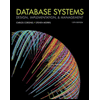
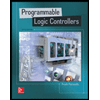