How to input the following code # Open the input file and read the dictionary with open("books.txt", "r") as file: favorite_books = {} for line in file: title, author, year = line.strip().split(", ") favorite_books[title] = [author, int(year)] # Invert the dictionary using the function from the previous program def invert_dict(d): inverse = dict() for key in d: vals = d[key] for val in vals: if val not in inverse: inverse[val] = [key] else: inverse[val].append(key) return inverse inverted_dict = invert_dict(favorite_books) # Write the inverted dictionary to a file with open("inverted_books.txt", "w") as file: for key, value in inverted_dict.items(): file.write(str(key) + ": " + str(value) + "\n")
How to input the following code
# Open the input file and read the dictionary
with open("books.txt", "r") as file:
favorite_books = {}
for line in file:
title, author, year = line.strip().split(", ")
favorite_books[title] = [author, int(year)]
# Invert the dictionary using the function from the previous program
def invert_dict(d):
inverse = dict()
for key in d:
vals = d[key]
for val in vals:
if val not in inverse:
inverse[val] = [key]
else:
inverse[val].append(key)
return inverse
inverted_dict = invert_dict(favorite_books)
# Write the inverted dictionary to a file
with open("inverted_books.txt", "w") as file:
for key, value in inverted_dict.items():
file.write(str(key) + ": " + str(value) + "\n")

Step by step
Solved in 4 steps with 3 images

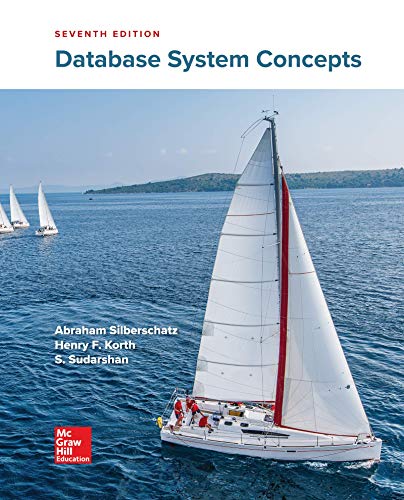
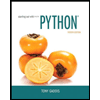
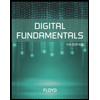
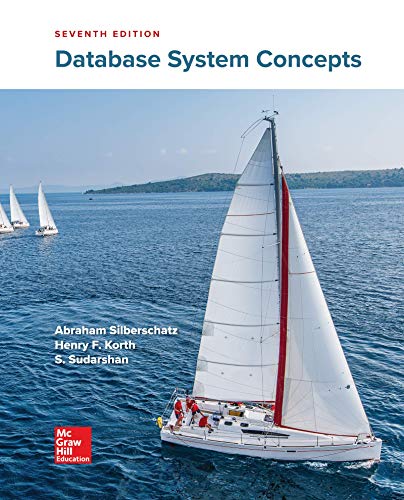
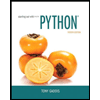
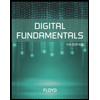
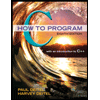
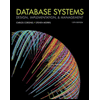
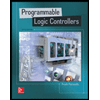