Add a prompt to ask the user to input their last name (no spaces ; please use underscores if needed), age and a balance of their choice. Create a new entry in the patient_list array with this information [Important note: you can statically modify the code to have the patient_list array hold an extra entry ; no need to do any dynamic memory allocation to accommodate the new entry].
Add a prompt to ask the user to input their last name (no spaces ; please use underscores if needed), age and a balance of their choice. Create a new entry in the patient_list array with this information [Important note: you can statically modify the code to have the patient_list array hold an extra entry ; no need to do any dynamic memory allocation to accommodate the new entry].
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
- Add a prompt to ask the user to input their last name (no spaces ; please use underscores if needed), age and a balance of their choice. Create a new entry in the patient_list array with this information [Important note: you can statically modify the code to have the patient_list array hold an extra entry ; no need to do any dynamic memory allocation to accommodate the new entry].
#include <iostream>
#include <cstdlib>
#include <cstring>
using namespace std;
// Declaring a new struct to store patient data
struct patient {
int age;
char name[20];
float balance;
};
// TODO:
// IMPLEMENT A FUNCTION THAT COMPARES TWO PATIENTS BY AGE
// THE FUNCTION RETURNS AN INTEGER AS FOLLOWS:
// -1 IF THE AGE OF THE FIRST PATIENT IS LESS
// THAN THE SECOND PATIENT'S AGE
// 0 IF THE AGES ARE EQUAL
// 1 OTHERWISE
// TODO:
// IMPLEMENT A FUNCTION THAT COMPARES TWO PATIENTS BY BALANCE DUE
// THE FUNCTION RETURNS AN INTEGER AS FOLLOWS:
// -1 IF THE BALANCE FOR THE FIRST PATIENT IS LESS
// THAN THE SECOND PATIENT'S BALANCE
// 0 IF THE BALANCES ARE EQUAL
// 1 OTHERWISE
// TODO:
// IMPLEMENT A FUNCTION THAT COMPARES TWO PATIENTS BY NAME
// THE FUNCTION RETURNS AN INTEGER AS FOLLOWS:
// -1 IF THE NAME OF THE FIRST PATIENT GOES BEFORE
// THE SECOND PATIENT'S NAME
// 0 IF THE AGES ARE EQUAL
// 1 OTHERWISE
//
// HINT: USE THE strncmp FUNCTION
// (SEE http://www.cplusplus.com/reference/cstring/strncmp/)
// The main program
int main()
{
int total_patients = 10;
// Storing some test data
struct patient patient_list[total_patients] = {
{25, "Juan Valdez ", 1250},
{15, "James Morris ", 2100},
{32, "Tyra Banks ", 750},
{62, "Mario O'Donell", 375},
{53, "Pablo Picasso ", 615}
};
patient p1;
for (int i = 0; i < total_patients; i++) {
cout << "Enter last name of your choice: " << endl;
cin >> p1.name;
cout << "Enter your age of your choice: " <<endl;
cin >> p1.age;
cout << "Enter balance of your choice: " <<endl;
cin >> p1.balance;
}
cout << "Patient List: " << endl;
// TODO:
// IMPLEMENT THE CODE TO DISPLAY THE CONTENTS
// OF THE ARRAY BEFORE SORTING
cout << patient_list << endl;
cout << endl;
cout << "Sorting..." << endl;
// TODO:
// CALL THE qsort FUNCTION TO SORT THE ARRAY BY PATIENT AGE
cout << "Patient List - Sorted by Age: " << endl;
// TODO:
// DISPLAY THE CONTENTS OF THE ARRAY
// AFTER SORTING BY AGE
cout << endl;
cout << "Sorting..." << endl;
// TODO:
// CALL THE qsort FUNCTION TO SORT THE ARRAY BY PATIENT BALANCE
cout << "Patient List - Sorted by Balance Due: " << endl;
// TODO:
// DISPLAY THE CONTENTS OF THE ARRAY
// AFTER SORTING BY BALANCE
cout << endl;
cout << "Sorting..." << endl;
// TODO:
// CALL THE qsort FUNCTION TO SORT THE ARRAY BY PATIENT NAME
cout << "Patient List - Sorted by Name: " << endl;
// TODO:
// DISPLAY THE CONTENTS OF THE ARRAY
// AFTER SORTING BY NAME
cout << endl;
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
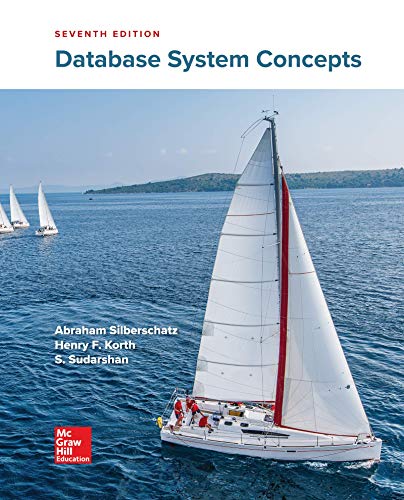
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
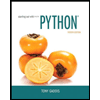
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
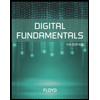
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
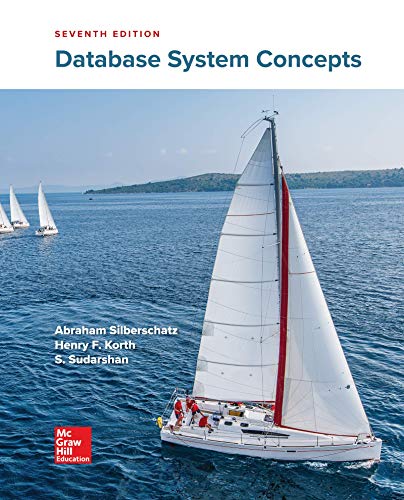
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
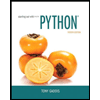
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
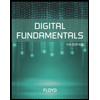
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
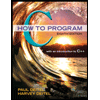
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
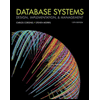
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
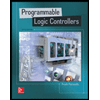
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education