The following program rotates a cube with color interpolation , where colors are assigned to the vertices and mouse buttons control the direction of the rotation. Create the code /* cube.cpp */ #include #include static GLfloat theta[] = {0.0,0.0,0.0}; static GLint axis = 2; GLfloat vertices[][3] = {{-1.0,-1.0,-1.0},{1.0,-1.0,-1.0}, {1.0,1.0,-1.0}, {-1.0,1.0,-1.0}, {-1.0,-1.0,1.0}, {1.0,-1.0,1.0}, {1.0,1.0,1.0}, {-1.0,1.0,1.0}}; GLfloat colors[][3] = {{0.0,0.0,0.0}, {0.0,0.0,1.0}, {0.0,1.0,0.0}, {0.0,1.0,1.0}, {1.0,0.0,0.0}, {1.0,0.0,1.0}, {1.0,1.0,0.0}, {1.0,1.0,1.0}}; // draw a polygon via list of vertices void polygon(int a, int b, int c , int d) { glBegin(GL_POLYGON); glColor3fv(colors[a]); glVertex3fv(vertices[a]); glColor3fv(colors[b]); glVertex3fv(vertices[b]); glColor3fv(colors[c]); glVertex3fv(vertices[c]); glColor3fv(colors[d]); glVertex3fv(vertices[d]); glEnd(); } // map vertices to faces void colorcube(void) { polygon(0,3,2,1); polygon(2,3,7,6); polygon(0,4,7,3); polygon(1,2,6,5); polygon(4,5,6,7); polygon(0,1,5,4); } // display callback void display(void) { // clear frame buffer and z buffer, // rotate cube and draw, swap buffers glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT); glLoadIdentity(); glRotatef(theta[0], 1.0, 0.0, 0.0); glRotatef(theta[1], 0.0, 1.0, 0.0); glRotatef(theta[2], 0.0, 0.0, 1.0); colorcube(); glFlush(); glutSwapBuffers(); } // idle callback, spin cube 2 degrees about selected axis void spinCube() { theta[axis] += 2.0; if( theta[axis] > 360.0 ) theta[axis] -= 360.0; glutPostRedisplay(); } // mouse callback void mouse(int btn, int state, int x, int y) { // selects an axis about which to rotate if(btn==GLUT_LEFT_BUTTON && state == GLUT_DOWN) axis = 0; if(btn==GLUT_MIDDLE_BUTTON && state == GLUT_DOWN) axis = 1; if(btn==GLUT_RIGHT_BUTTON && state == GLUT_DOWN) axis = 2; } // reshape callback void myReshape(int w, int h) { glViewport(0, 0, w, h); glMatrixMode(GL_PROJECTION); glLoadIdentity(); if (w <= h) glOrtho(-2.0, 2.0, -2.0 * (GLfloat) h / (GLfloat) w, 2.0 * (GLfloat) h / (GLfloat) w, -10.0, 10.0); else glOrtho(-2.0 * (GLfloat) w / (GLfloat) h, 2.0 * (GLfloat) w / (GLfloat) h, -2.0, 2.0, -10.0, 10.0); glMatrixMode(GL_MODELVIEW); } // main function int main(int argc, char **argv) { glutInit(&argc, argv); // need both double buffering and z buffer glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH); glutInitWindowSize(500, 500); glutCreateWindow("Color Cube"); glutReshapeFunc(myReshape); glutDisplayFunc(display); glutIdleFunc(spinCube); glutMouseFunc(mouse); glEnable(GL_DEPTH_TEST); // Enable hidden--surface--removal glutMainLoop(); return 0; }
The following
/* cube.cpp */
#include <stdlib.h>
#include <GL/glut.h>
static GLfloat theta[] = {0.0,0.0,0.0};
static GLint axis = 2;
GLfloat vertices[][3] = {{-1.0,-1.0,-1.0},{1.0,-1.0,-1.0},
{1.0,1.0,-1.0}, {-1.0,1.0,-1.0}, {-1.0,-1.0,1.0},
{1.0,-1.0,1.0}, {1.0,1.0,1.0}, {-1.0,1.0,1.0}};
GLfloat colors[][3] = {{0.0,0.0,0.0}, {0.0,0.0,1.0},
{0.0,1.0,0.0}, {0.0,1.0,1.0}, {1.0,0.0,0.0},
{1.0,0.0,1.0}, {1.0,1.0,0.0}, {1.0,1.0,1.0}};
// draw a polygon via list of vertices
void polygon(int a, int b, int c , int d)
{
glBegin(GL_POLYGON);
glColor3fv(colors[a]);
glVertex3fv(vertices[a]);
glColor3fv(colors[b]);
glVertex3fv(vertices[b]);
glColor3fv(colors[c]);
glVertex3fv(vertices[c]);
glColor3fv(colors[d]);
glVertex3fv(vertices[d]);
glEnd();
}
// map vertices to faces
void colorcube(void)
{
polygon(0,3,2,1);
polygon(2,3,7,6);
polygon(0,4,7,3);
polygon(1,2,6,5);
polygon(4,5,6,7);
polygon(0,1,5,4);
}
// display callback
void display(void)
{
// clear frame buffer and z buffer,
// rotate cube and draw, swap buffers
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glLoadIdentity();
glRotatef(theta[0], 1.0, 0.0, 0.0);
glRotatef(theta[1], 0.0, 1.0, 0.0);
glRotatef(theta[2], 0.0, 0.0, 1.0);
colorcube();
glFlush();
glutSwapBuffers();
}
// idle callback, spin cube 2 degrees about selected axis
void spinCube()
{
theta[axis] += 2.0;
if( theta[axis] > 360.0 ) theta[axis] -= 360.0;
glutPostRedisplay();
}
// mouse callback
void mouse(int btn, int state, int x, int y)
{
// selects an axis about which to rotate
if(btn==GLUT_LEFT_BUTTON && state == GLUT_DOWN) axis = 0;
if(btn==GLUT_MIDDLE_BUTTON && state == GLUT_DOWN) axis = 1;
if(btn==GLUT_RIGHT_BUTTON && state == GLUT_DOWN) axis = 2;
}
// reshape callback
void myReshape(int w, int h)
{
glViewport(0, 0, w, h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
if (w <= h)
glOrtho(-2.0, 2.0, -2.0 * (GLfloat) h / (GLfloat) w,
2.0 * (GLfloat) h / (GLfloat) w, -10.0, 10.0);
else
glOrtho(-2.0 * (GLfloat) w / (GLfloat) h,
2.0 * (GLfloat) w / (GLfloat) h, -2.0, 2.0, -10.0, 10.0);
glMatrixMode(GL_MODELVIEW);
}
// main function
int main(int argc, char **argv)
{
glutInit(&argc, argv);
// need both double buffering and z buffer
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH);
glutInitWindowSize(500, 500);
glutCreateWindow("Color Cube");
glutReshapeFunc(myReshape);
glutDisplayFunc(display);
glutIdleFunc(spinCube);
glutMouseFunc(mouse);
glEnable(GL_DEPTH_TEST); // Enable hidden--surface--removal
glutMainLoop();
return 0;
}
![```cpp
/* cube.cpp */
#include <stdlib.h>
#include <GL/glut.h>
static GLfloat theta[] = {0.0, 0.0, 0.0};
static GLint axis = 2;
GLfloat vertices[][3] = {
{-1.0, -1.0, -1.0}, {1.0, -1.0, -1.0},
{1.0, 1.0, -1.0}, {-1.0, 1.0, -1.0},
{-1.0, -1.0, 1.0}, {1.0, -1.0, 1.0},
{1.0, 1.0, 1.0}, {-1.0, 1.0, 1.0}
};
GLfloat colors[][3] = {
{0.0, 0.0, 0.0}, {0.0, 0.0, 1.0},
{0.0, 1.0, 0.0}, {0.0, 1.0, 1.0},
{1.0, 0.0, 0.0}, {1.0, 0.0, 1.0},
{1.0, 1.0, 0.0}, {1.0, 1.0, 1.0}
};
// draw a polygon via list of vertices
void polygon(int a, int b, int c, int d)
{
glBegin(GL_POLYGON);
glColor3fv(colors[a]);
glVertex3fv(vertices[a]);
glColor3fv(colors[b]);
glVertex3fv(vertices[b]);
glColor3fv(colors[c]);
glVertex3fv(vertices[c]);
glColor3fv(colors[d]);
glVertex3fv(vertices[d]);
glEnd();
}
// map vertices to faces
void colorcube(void)
{
polygon(0, 3, 2, 1);
polygon(2, 3, 7, 6);
polygon(0, 4, 7, 3);
polygon(1, 2, 6, 5);
polygon(4, 5, 6, 7);
polygon(0, 1,](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fca46498c-9039-4635-914d-0b10c3becf43%2F1caf9b70-7375-4d11-9339-d6356f963ec9%2F89wpjak_processed.jpeg&w=3840&q=75)
![```c
glutSwapBuffers();
}
// idle callback, spin cube 2 degrees about selected axis
void spinCube()
{
theta[axis] += 2.0;
if( theta[axis] > 360.0 ) theta[axis] -= 360.0;
glutPostRedisplay();
}
// mouse callback
void mouse(int btn, int state, int x, int y)
{
// selects an axis about which to rotate
if(btn==GLUT_LEFT_BUTTON && state == GLUT_DOWN) axis = 0;
if(btn==GLUT_MIDDLE_BUTTON && state == GLUT_DOWN) axis = 1;
if(btn==GLUT_RIGHT_BUTTON && state == GLUT_DOWN) axis = 2;
}
// reshape callback
void myReshape(int w, int h)
{
glViewport(0, 0, w, h);
glMatrixMode(GL_PROJECTION);
if (w <= h)
glOrtho(-2.0, 2.0, -2.0 * (GLfloat) h / (GLfloat) w,
2.0 * (GLfloat) h / (GLfloat) w, -10.0, 10.0);
else
glOrtho(-2.0 * (GLfloat) w / (GLfloat) h,
2.0 * (GLfloat) w / (GLfloat) h, -2.0, 2.0, -10.0, 10.0);
glMatrixMode(GL_MODELVIEW);
}
// main function
int main(int argc, char **argv)
{
glutInit(&argc, argv);
// need both double buffering and z buffer
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH);
glutInitWindowSize(500, 500);
glutCreateWindow("Color Cube");
glutReshapeFunc(myReshape);
glutDisplayFunc(display);
glutIdleFunc(spinCube);
glutMouseFunc(mouse);
glEnable(GL_DEPTH_TEST); // Enable hidden-surface-removal
glutMainLoop();
return 0;
}
```
### Explanation
This code is for a 3D graphics application using the OpenGL Utility Toolkit (GLUT). It implements the following key functionalities:
- **Idle Callback (`spinCube`)**: This function rotates a cube by 2 degrees around a selected](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fca46498c-9039-4635-914d-0b10c3becf43%2F1caf9b70-7375-4d11-9339-d6356f963ec9%2Fjdyyjj2_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

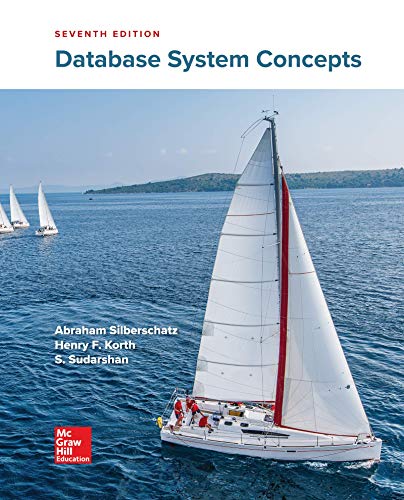
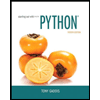
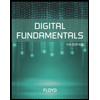
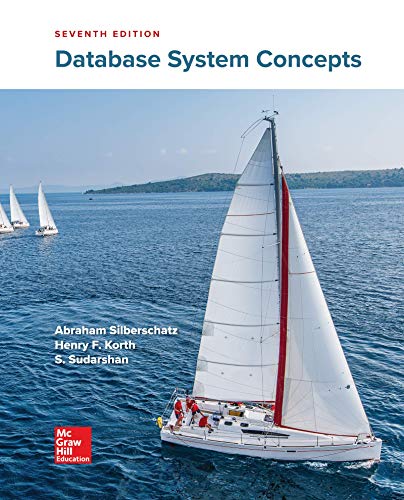
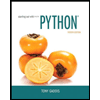
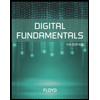
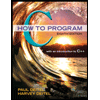
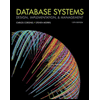
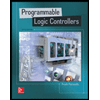