import image def rotate(pic): '''Returns an image rotated 90 degrees''' pass #You can leave this or remove it #Your code here to rotate the image #Hint: you'll have to create an empty image that swaps the #width and height of pic - it will have the same number of #columns as pic has rows and the same number of rows as #pic has columns. Then copy the pixels from pic into the #rotated positions in the empty image. def main():
import image def rotate(pic): '''Returns an image rotated 90 degrees''' pass #You can leave this or remove it #Your code here to rotate the image #Hint: you'll have to create an empty image that swaps the #width and height of pic - it will have the same number of #columns as pic has rows and the same number of rows as #pic has columns. Then copy the pixels from pic into the #rotated positions in the empty image. def main():
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:```python
import image
def rotate(pic):
'''Returns an image rotated 90 degrees'''
pass # You can leave this or remove it
# Your code here to rotate the image
# Hint: you'll have to create an empty image that swaps the
# width and height of pic - it will have the same number of
# columns as pic has rows and the same number of rows as
# pic has columns. Then copy the pixels from pic into the
# rotated positions in the empty image.
def main():
```
**Explanation:**
The code snippet is designed to rotate an image by 90 degrees. It includes a function `rotate()` that accepts a parameter `pic`, representing the image to be rotated.
- The `rotate()` function contains a docstring that describes its purpose: to return an image rotated by 90 degrees.
- Inside the function, the `pass` statement is a placeholder indicating where the rotation logic should be implemented.
**Steps to Implement the Rotation:**
1. **Create an Empty Image**:
- To rotate the image, a new image needs to be created with swapped dimensions. This new image will have the width equal to the height of the original image and the height equal to the width of the original image.
2. **Copy Pixels**:
- Iterate over each pixel in the original image.
- Map and copy each pixel to its new location in the empty image.
The `main()` function is defined to possibly execute the code but currently lacks implementation details.

Transcribed Image Text:### Python Program for Image Processing
Below is a Python code snippet for a simple image processing program. It utilizes the graphics library to load, manipulate, and display an image.
```python
def main():
'''Controls the program'''
fname = "https://runestone.academy/runestone/static/instructorguide/_static/arch.jpg"
img = image.Image(fname)
width = img.getWidth()
height = img.getHeight()
win = image.ImageWin(height, width)
rotate(img)
img.draw(win)
main() # Run the program
```
#### Explanation
- **Function `main()`:** This controls the program execution. It is responsible for loading and displaying an image.
- **fname:** A string containing the URL of the image (`arch.jpg`), which will be processed.
- **img:** The image object is created using the specified file name.
- **width & height:** The dimensions of the image are retrieved through `getWidth()` and `getHeight()`.
- **win:** An `ImageWin` object is created with the dimensions of the image, which serves as a window to display the image.
- **rotate(img):** A function call to rotate the image (implementation not shown here).
- **img.draw(win):** Draws the image onto the window provided by `ImageWin`.
#### Image Display
- The image displayed is of an archway covered with greenery and flowers, giving a sense of architectural beauty and natural aesthetics. This image serves as an example for the image processing task executed by the Python program.
This educational website section intends to guide students through basic image manipulation using Python, demonstrating reading, modifying, and displaying an image with simple code.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
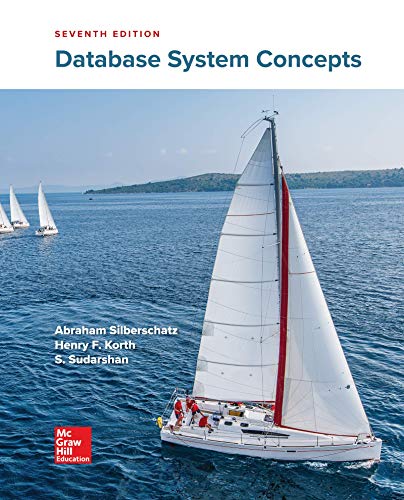
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
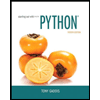
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
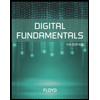
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
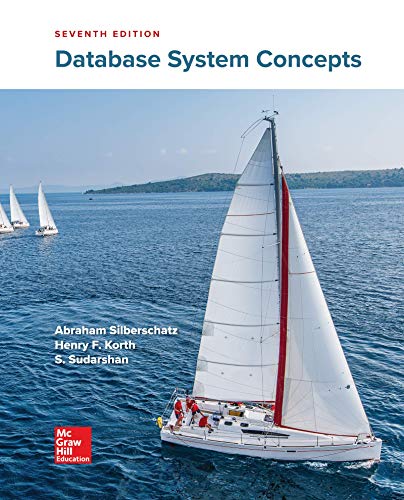
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
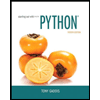
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
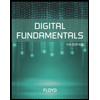
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
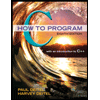
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
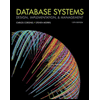
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
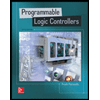
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education