Part 1.2. Write a main() method inside of the HybridCourse class, having the following: 1. Create an instance for HybridCourse using the constructor with all data fields. 2. Create an instance for OnlineCourse using the constructor with all data fields. 3. Print the two instances using toString method and match the example output: @Javadoc Declaration Console X Properties Course [Java Application] /Users/kuan/.p2/pool/plugins/org.eclipse.justj.openjdk.hotspot.jre.full.macosx.x86_64_17.0.4.v20220805-1047/jre/bin/java (Nov 13, HybridCourse [courseName=CPS1000, section Number=1, instructorName=Tom, percentOfRemoteLearning=50] OnlineCourse [courseName=CPS1001, section Number=2, instructorName=Jack, platform-Zoom, meeting Link-www.xxx.com] Task 1: Abstract Class (15 Points) HybridCourse percentOfRemoteLearning: int +HybridCourse() +HybridCourse(courseName: String, sectiomNumber: int, instructorName: String, percentOfRemoteLearning: int) +getCapacity() int +getPercentOfRemoteLearning(): int +setPercentOfRemoteLearning (percentOfRemoteLearning: int): void +toString(): String Course -courseName: String - sectionNumber: int - instructorName: String - numberOfStudentsEnrolled: int #Course() #Course(courseName: String) #Course(courseName: String, sectionNumber: int, instructorName: String) +getCapacity(): int +get CourseName(): String +set CourseName(courseName: String): void +getSection Number(): int +setSection Number(section Number: int): void +getInstructorName(): String +setInstructorName(instructorName: String): void +getNumberOfStudentsEnrolled(): int +toString(): String - platform: String Online Course - meetingLink: String sectionNumber: int, + OnlineCourse() + OnlineCourse(courseName: String, instructorName: String, platform: String, meetingLink: String) #getMeetingLink(): String #setMeetingLink (meetingLink: String): void +getCapacity(): int +toString(): String Task 1 Abstract Class, Abstract Method and Inheritance Part 1.1. Implement the Course, HybridCourse, OnlineCourse inheritance structure strictly according to its UML diagram in three different .java files. Add comments stating where data fields, constructors, toString(), and other methods are (if any). Neither method should have an empty body unless abstract methods. Note: You should implement the abstract class Course, regular class HybridCourse, and regular class OnlineCourse. Note: the getCapacity() in the HybridCourse and OnlineCourse should return an integer value representing the maximum student number in the class. toString() Methods should be implemented properly in order to match the output requirements in Part 1.2.
Part 1.2. Write a main() method inside of the HybridCourse class, having the following: 1. Create an instance for HybridCourse using the constructor with all data fields. 2. Create an instance for OnlineCourse using the constructor with all data fields. 3. Print the two instances using toString method and match the example output: @Javadoc Declaration Console X Properties Course [Java Application] /Users/kuan/.p2/pool/plugins/org.eclipse.justj.openjdk.hotspot.jre.full.macosx.x86_64_17.0.4.v20220805-1047/jre/bin/java (Nov 13, HybridCourse [courseName=CPS1000, section Number=1, instructorName=Tom, percentOfRemoteLearning=50] OnlineCourse [courseName=CPS1001, section Number=2, instructorName=Jack, platform-Zoom, meeting Link-www.xxx.com] Task 1: Abstract Class (15 Points) HybridCourse percentOfRemoteLearning: int +HybridCourse() +HybridCourse(courseName: String, sectiomNumber: int, instructorName: String, percentOfRemoteLearning: int) +getCapacity() int +getPercentOfRemoteLearning(): int +setPercentOfRemoteLearning (percentOfRemoteLearning: int): void +toString(): String Course -courseName: String - sectionNumber: int - instructorName: String - numberOfStudentsEnrolled: int #Course() #Course(courseName: String) #Course(courseName: String, sectionNumber: int, instructorName: String) +getCapacity(): int +get CourseName(): String +set CourseName(courseName: String): void +getSection Number(): int +setSection Number(section Number: int): void +getInstructorName(): String +setInstructorName(instructorName: String): void +getNumberOfStudentsEnrolled(): int +toString(): String - platform: String Online Course - meetingLink: String sectionNumber: int, + OnlineCourse() + OnlineCourse(courseName: String, instructorName: String, platform: String, meetingLink: String) #getMeetingLink(): String #setMeetingLink (meetingLink: String): void +getCapacity(): int +toString(): String Task 1 Abstract Class, Abstract Method and Inheritance Part 1.1. Implement the Course, HybridCourse, OnlineCourse inheritance structure strictly according to its UML diagram in three different .java files. Add comments stating where data fields, constructors, toString(), and other methods are (if any). Neither method should have an empty body unless abstract methods. Note: You should implement the abstract class Course, regular class HybridCourse, and regular class OnlineCourse. Note: the getCapacity() in the HybridCourse and OnlineCourse should return an integer value representing the maximum student number in the class. toString() Methods should be implemented properly in order to match the output requirements in Part 1.2.
Chapter9: Using Classes And Objects
Section: Chapter Questions
Problem 19RQ
Related questions
Question
![Part 1.2. Write a main() method inside of the HybridCourse class, having the following:
1. Create an instance for HybridCourse using the constructor with all data fields.
2. Create an instance for OnlineCourse using the constructor with all data fields.
3. Print the two instances using toString method and match the example output:
@Javadoc Declaration Console X Properties
<terminated> Course [Java Application] /Users/kuan/.p2/pool/plugins/org.eclipse.justj.openjdk.hotspot.jre.full.macosx.x86_64_17.0.4.v20220805-1047/jre/bin/java (Nov 13,
HybridCourse [courseName=CPS1000, section Number=1, instructorName=Tom, percentOfRemoteLearning=50]
OnlineCourse [courseName=CPS1001, section Number=2, instructorName=Jack, platform-Zoom, meeting Link-www.xxx.com]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1f1cff89-d0c3-401a-ba77-d9b5f81fcda4%2F1cf22a9a-d44b-4345-a620-e086a6f24ab8%2Flfbraa6_processed.png&w=3840&q=75)
Transcribed Image Text:Part 1.2. Write a main() method inside of the HybridCourse class, having the following:
1. Create an instance for HybridCourse using the constructor with all data fields.
2. Create an instance for OnlineCourse using the constructor with all data fields.
3. Print the two instances using toString method and match the example output:
@Javadoc Declaration Console X Properties
<terminated> Course [Java Application] /Users/kuan/.p2/pool/plugins/org.eclipse.justj.openjdk.hotspot.jre.full.macosx.x86_64_17.0.4.v20220805-1047/jre/bin/java (Nov 13,
HybridCourse [courseName=CPS1000, section Number=1, instructorName=Tom, percentOfRemoteLearning=50]
OnlineCourse [courseName=CPS1001, section Number=2, instructorName=Jack, platform-Zoom, meeting Link-www.xxx.com]

Transcribed Image Text:Task 1: Abstract Class (15 Points)
HybridCourse
percentOfRemoteLearning: int
+HybridCourse()
+HybridCourse(courseName: String, sectiomNumber: int,
instructorName: String, percentOfRemoteLearning: int)
+getCapacity() int
+getPercentOfRemoteLearning(): int
+setPercentOfRemoteLearning (percentOfRemoteLearning:
int): void
+toString(): String
Course
-courseName: String
- sectionNumber: int
- instructorName: String
- numberOfStudentsEnrolled: int
#Course()
#Course(courseName: String)
#Course(courseName: String, sectionNumber: int,
instructorName: String)
+getCapacity(): int
+get CourseName(): String
+set CourseName(courseName: String): void
+getSection Number(): int
+setSection Number(section Number: int): void
+getInstructorName(): String
+setInstructorName(instructorName: String): void
+getNumberOfStudentsEnrolled(): int
+toString(): String
- platform: String
Online Course
- meetingLink: String
sectionNumber: int,
+ OnlineCourse()
+ OnlineCourse(courseName: String,
instructorName: String,
platform: String,
meetingLink: String)
#getMeetingLink(): String
#setMeetingLink (meetingLink: String): void
+getCapacity(): int
+toString(): String
Task 1 Abstract Class, Abstract Method and Inheritance
Part 1.1. Implement the Course, HybridCourse, OnlineCourse inheritance structure strictly
according to its UML diagram in three different .java files. Add comments stating where data
fields, constructors, toString(), and other methods are (if any).
Neither method should have an empty body unless abstract methods.
Note: You should implement the abstract class Course, regular class HybridCourse, and
regular class OnlineCourse.
Note: the getCapacity() in the HybridCourse and OnlineCourse should return an integer
value representing the maximum student number in the class.
toString() Methods should be implemented properly in order to match the output requirements in
Part 1.2.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
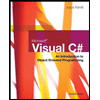
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
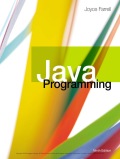
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
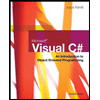
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
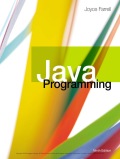
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
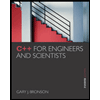
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
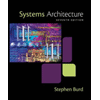
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
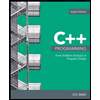
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning