The following is code for a disc golf program written in C++: player.h: #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H player.cpp: #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum score } return; // Exit after finding the course } } // If course not found, add a new course and score if (gameCount < 20) { courses[gameCount] = courseName; scores[gameCount] = gameScore; ++gameCount; } } void Player::ReportPlayer(int playerId) const { if (gameCount == 0) { std::cout << "Player P" << playerId << " has no games\n"; } else { std::cout << "Player P" << playerId << "\n"; for (int i = 0; i < gameCount; ++i) { std::cout << "\t" << courses[i] << " " << scores[i] << "\n"; } } } main.cpp: #include <iostream> #include <string> #include <algorithm> #include <limits> #include "player.h" int main() { Player players[10]; // Array to hold players std::string playerCode; int inId, inScore; std::string inCourse; int maxPlayerIndex = -1; // Track the highest player ID while (true) { std::cout << "Enter player code or Q to quit: "; std::cin >> playerCode; // Check for quit input if (playerCode == "q" || playerCode == "Q") break; // Validate player code if (playerCode.length() != 2 || playerCode[0] != 'P' || playerCode[1] < '0' || playerCode[1] > '9') { std::cout << "Invalid player code. Please enter P0-P9.\n"; continue; } inId = playerCode[1] - '0'; // Extract player ID if (inId < 0 || inId > 9) { std::cerr << "Invalid player ID detected.\n"; continue; } maxPlayerIndex = std::max(maxPlayerIndex, inId); // Update the highest player ID std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // Input course name std::cout << "Enter course name: "; std::getline(std::cin, inCourse); // Input game score std::cout << "Enter game score: "; std::cin >> inScore; std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); std::cout << std::endl; // Register the game for the player players[inId].CheckGame(inCourse, inScore); } // Generate a report for all players std::cout << "\nReport\n"; for (int i = 0; i <= maxPlayerIndex; ++i) { players[i].ReportPlayer(i); } return 0; } Edit the program to allow both uppercase (as in P2) and lowercase (as in p2) inputs
The following is code for a disc golf program written in C++:
player.h:
#ifndef PLAYER_H
#define PLAYER_H
#include <string>
#include <iostream>
class Player {
private:
std::string courses[20]; // Array of course names
int scores[20]; // Array of scores
int gameCount; // Number of games played
public:
Player(); // Constructor
void CheckGame(const std::string& courseName, int gameScore);
void ReportPlayer(int playerId) const;
};
#endif // PLAYER_H
player.cpp:
#include "player.h"
#include <iomanip>
Player::Player() : gameCount(0) {}
void Player::CheckGame(const std::string& courseName, int gameScore) {
for (int i = 0; i < gameCount; ++i) {
if (courses[i] == courseName) {
// If course has been played, check for minimum score
if (gameScore < scores[i]) {
scores[i] = gameScore; // Update to new minimum score
}
return; // Exit after finding the course
}
}
// If course not found, add a new course and score
if (gameCount < 20) {
courses[gameCount] = courseName;
scores[gameCount] = gameScore;
++gameCount;
}
}
void Player::ReportPlayer(int playerId) const {
if (gameCount == 0) {
std::cout << "Player P" << playerId << " has no games\n";
} else {
std::cout << "Player P" << playerId << "\n";
for (int i = 0; i < gameCount; ++i) {
std::cout << "\t" << courses[i] << " " << scores[i] << "\n";
}
}
}
main.cpp:
#include <iostream>
#include <string>
#include <algorithm>
#include <limits>
#include "player.h"
int main() {
Player players[10]; // Array to hold players
std::string playerCode;
int inId, inScore;
std::string inCourse;
int maxPlayerIndex = -1; // Track the highest player ID
while (true) {
std::cout << "Enter player code or Q to quit: ";
std::cin >> playerCode;
// Check for quit input
if (playerCode == "q" || playerCode == "Q") break;
// Validate player code
if (playerCode.length() != 2 || playerCode[0] != 'P' || playerCode[1] < '0' || playerCode[1] > '9') {
std::cout << "Invalid player code. Please enter P0-P9.\n";
continue;
}
inId = playerCode[1] - '0'; // Extract player ID
if (inId < 0 || inId > 9) {
std::cerr << "Invalid player ID detected.\n";
continue;
}
maxPlayerIndex = std::max(maxPlayerIndex, inId); // Update the highest player ID
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
// Input course name
std::cout << "Enter course name: ";
std::getline(std::cin, inCourse);
// Input game score
std::cout << "Enter game score: ";
std::cin >> inScore;
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
std::cout << std::endl;
// Register the game for the player
players[inId].CheckGame(inCourse, inScore);
}
// Generate a report for all players
std::cout << "\nReport\n";
for (int i = 0; i <= maxPlayerIndex; ++i) {
players[i].ReportPlayer(i);
}
return 0;
}
Edit the program to allow both uppercase (as in P2) and lowercase (as in p2) inputs

Step by step
Solved in 2 steps

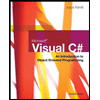
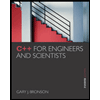
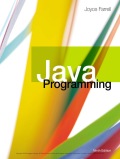
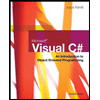
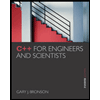
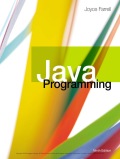
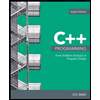
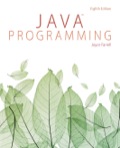