Question 1. What is the time complexity for the following code/program? 1.1 for (int i = 1; i <= n; i++){ for (int j = 1; j <= n; j++){ 1.2 int a = 0; for (int k = n; k >= 1; k--){ sum = i + j+k; cout << sum << endl;}}} for (i = 0; i < N; i++) { for (j=N; j>i; j--) { a = a +i+j;}} 1.3 for (int i = 1; i <= n; i++){ for (int j = 1; j <= 100; j++){ sum = i + j + k;}}
Question 1. What is the time complexity for the following code/program? 1.1 for (int i = 1; i <= n; i++){ for (int j = 1; j <= n; j++){ 1.2 int a = 0; for (int k = n; k >= 1; k--){ sum = i + j+k; cout << sum << endl;}}} for (i = 0; i < N; i++) { for (j=N; j>i; j--) { a = a +i+j;}} 1.3 for (int i = 1; i <= n; i++){ for (int j = 1; j <= 100; j++){ sum = i + j + k;}}
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:## Question 1: What is the time complexity for the following code/program?
### 1.1
```cpp
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= n; j++) {
for (int k = n; k >= 1; k--) {
sum = i + j + k;
cout << sum << endl;
}
}
}
```
- **Explanation:** This code contains three nested loops, each dependent on `n`. The outer loop runs `n` times, the middle loop also runs `n` times for each iteration of the outer loop, and the innermost loop runs `n` times for each iteration of the middle loop.
- **Time Complexity:** \(O(n^3)\)
### 1.2
```cpp
int a = 0;
for (i = 0; i < N; i++) {
for (j = N; j > i; j--) {
a = a + i + j;
}
}
```
- **Explanation:** There are two nested loops. The outer loop runs `N` times. The inner loop runs `N - i` times for each iteration of the outer loop, decreasing as `i` increases.
- **Time Complexity:** \(O(N^2)\)
### 1.3
```cpp
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= 100; j++) {
sum = i + j;
}
}
```
- **Explanation:** The outer loop runs `n` times, and the inner loop always runs 100 times, regardless of `n`.
- **Time Complexity:** \(O(n)\)
### 1.4
```cpp
for (int i = 0; i < n; i++) {
for (int j = i; j > 0; j /= 2) {
cout << j << endl;
}
}
```
- **Explanation:** The outer loop runs `n` times. The inner loop performs a logarithmic division, reducing `j` by half each time, leading to `O(log i)` iterations.
- **Time Complexity:** \(O(n \log n)\)
### 1.5
**Description:** A binary search works by comparing the target value
Expert Solution

Step 1
As per Bartleby's rules, we can only answer first 3 subparts of a question
I request you to post other questions seperately
Solution for question 1.1, 1.2 and 1.3 are given below with proper explanation
Happy to help you ?
Trending now
This is a popular solution!
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
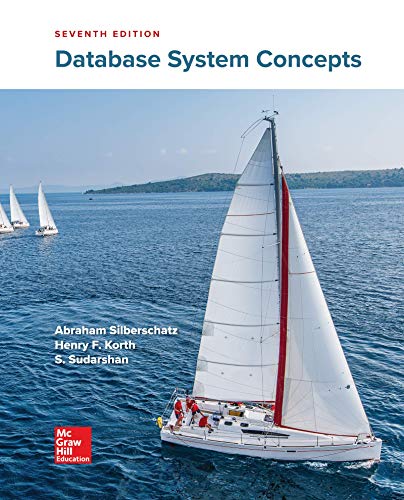
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
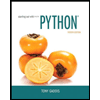
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
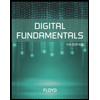
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
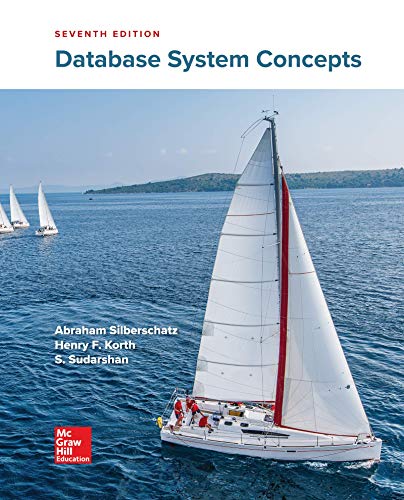
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
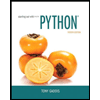
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
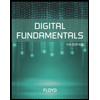
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
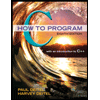
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
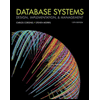
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
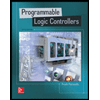
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education