Instruction: Explain the function of the program line by line thoroughly. Program: #include using namespace std; void insertionSort(int* arr, int size); int main() { cout << "Enter the number of integers: "; int size; cin >> size; // Allocate memory for the input array int* arr = new int[size]; for (int i = 0; i < size; i++) { cout << " Enter Integer No. " << (i+1) << ": "; cin >> arr[i]; } insertionSort(arr, size); cout << endl; cout << "Sorted Integers: "; for (int i = 0; i < size; i++) { cout << arr[i] << " "; } cout << endl; // Deallocate memory for the input array delete[] arr; return 0; } void insertionSort(int* arr, int size) { for (int i = 1; i < size; i++) { int key = arr[i]; int j = i - 1; while (j >= 0 && arr[j] > key) { arr[j + 1] = arr[j]; j--; } arr[j + 1] = key; } }
Instruction: Explain the function of the program line by line thoroughly.
Program:
#include <iostream>
using namespace std;
void insertionSort(int* arr, int size);
int main() {
cout << "Enter the number of integers: ";
int size;
cin >> size;
// Allocate memory for the input array
int* arr = new int[size];
for (int i = 0; i < size; i++)
{
cout << " Enter Integer No. " << (i+1) << ": ";
cin >> arr[i];
}
insertionSort(arr, size);
cout << endl;
cout << "Sorted Integers: ";
for (int i = 0; i < size; i++) {
cout << arr[i] << " ";
}
cout << endl;
// Deallocate memory for the input array
delete[] arr;
return 0;
}
void insertionSort(int* arr, int size)
{
for (int i = 1; i < size; i++) {
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}

Step by step
Solved in 5 steps with 3 images

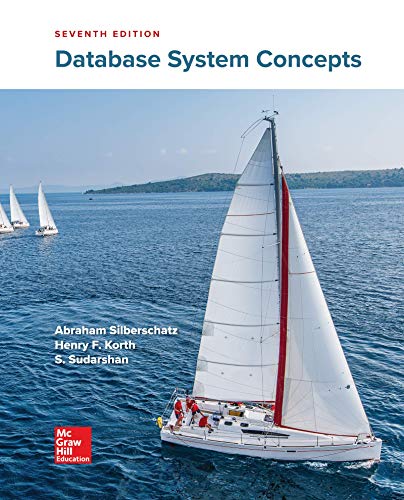
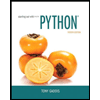
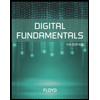
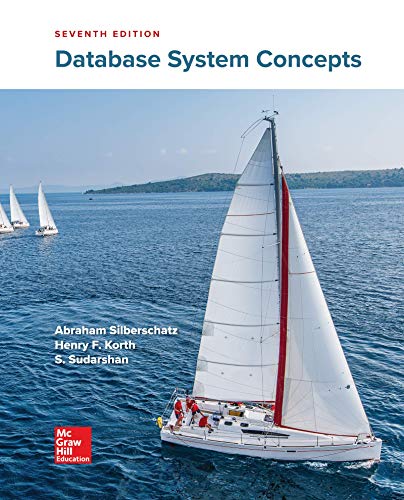
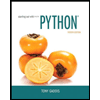
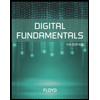
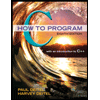
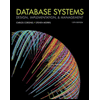
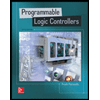