Please fill in the blanks for C. #include #include<__A__> // this library is needed to use exit(0) to terminal the program #include //Fill in the values of the original array __B__ scanArray(__C__ size, __D__ array[size]) { for(int i = 0;i <__E__; i++) { printf("Input value for element %d: ",i+1); scanf("__F__",&__G__); } } /* Printing a subset of the array based on the input range [start, stop] */ __H__ printArray(__I__ start,__J__ stop, __K__ size, __L__ array[size]) { for(int i = __M__;i <= __N__; i++) { printf("__O__",__P__); } } /* Return False (invalid) if either of these is true: begin index is negative, end index is size or bigger, begin index is bigger than end index. and True otherwise */ __Q__ checkValid(__R__ size, __S__ begin, __T__ end) { //condition checks for invalid and returns false or true accordingly //check function declaration above for invalid cases __U__ ((begin < __V__) __W__ (end __X__ size) __Y__ (begin __Z__ end)) ? false : true; } int main() { int size, start, stop; printf("Need 3 values. Enter array size, followed by the range(start, then stop): "); scanf("%d %d %d",__AA_, __BB__, __CC__); //create the array with appropriate size __DD__ arr[__EE__]; //check range's validity, look at checkValid function header to know parameters order __FF__ isValid = checkValid(__GG__,__HH__,__II__); if(!isValid)// check for invalid input { printf("The range is invalid. Exiting...\n"); __JJ__; //terminate the program } //Getting to this point means the range is valid because // if it is not valid, line 48 would terminate the program printf("I got the range. Now, let's fill in the array.\n"); scanArray(__KK__, __MM__); //fill in the array values printf("Printing array within range [%d, %d]\n", start, stop); printArray(__NN__,__OO__,__PP__,__QQ__); //printing back the subset array return 0; }
Please fill in the blanks for C.
#include<stdio.h>
#include<__A__> // this library is needed to use exit(0) to terminal the
#include<stdbool.h>
//Fill in the values of the original array
__B__ scanArray(__C__ size, __D__ array[size])
{
for(int i = 0;i <__E__; i++)
{
printf("Input value for element %d: ",i+1);
scanf("__F__",&__G__);
}
}
/*
Printing a subset of the array based on the input range [start, stop]
*/
__H__ printArray(__I__ start,__J__ stop, __K__ size, __L__ array[size])
{
for(int i = __M__;i <= __N__; i++)
{
printf("__O__",__P__);
}
}
/*
Return False (invalid) if either of these is true:
begin index is negative,
end index is size or bigger,
begin index is bigger than end index.
and True otherwise
*/
__Q__ checkValid(__R__ size, __S__ begin, __T__ end)
{
//condition checks for invalid and returns false or true accordingly
//check function declaration above for invalid cases
__U__ ((begin < __V__) __W__ (end __X__ size) __Y__ (begin __Z__ end)) ? false : true;
}
int main()
{
int size, start, stop;
printf("Need 3 values. Enter array size, followed by the range(start, then stop): ");
scanf("%d %d %d",__AA_, __BB__, __CC__);
//create the array with appropriate size
__DD__ arr[__EE__];
//check range's validity, look at checkValid function header to know parameters order
__FF__ isValid = checkValid(__GG__,__HH__,__II__);
if(!isValid)// check for invalid input
{
printf("The range is invalid. Exiting...\n");
__JJ__; //terminate the program
}
//Getting to this point means the range is valid because
// if it is not valid, line 48 would terminate the program
printf("I got the range. Now, let's fill in the array.\n");
scanArray(__KK__, __MM__); //fill in the array values
printf("Printing array within range [%d, %d]\n", start, stop);
printArray(__NN__,__OO__,__PP__,__QQ__); //printing back the subset array
return 0;
}

Step by step
Solved in 2 steps with 1 images

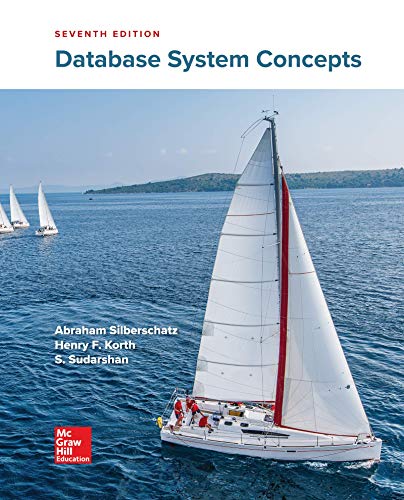
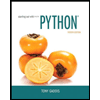
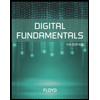
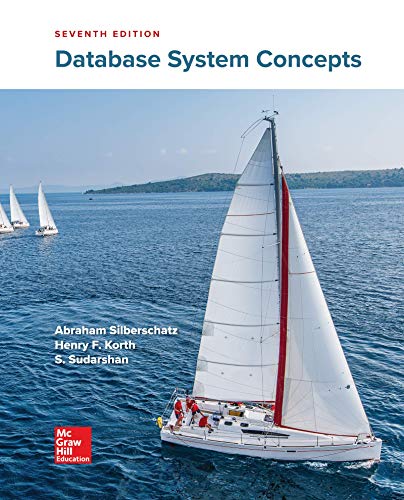
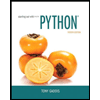
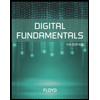
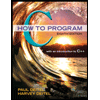
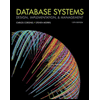
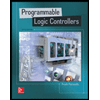