C++ Coding Assignment: Redo the 8 queens 1-dimensional array program with backtracking by REMOVING ALL GOTO's, but implementing the same algorithm. GIVEN C++ CODE - WITH GOTO's: #include using namespace std; int main() { int q[8], c=0; q[0] = 0; // place a queen in row 0 of column 0 int counter = 0; // to count solutions // c: column as q[c]: row in column c next_col: ++c; // or c++; // next column if(c==8) goto print; q[c]=-1; // 0 next_row: ++q[c]; // or q[c]++; // next row if(q[c]==8) goto backtrack; for(int i=0; i
C++ Coding Assignment: Redo the 8 queens 1-dimensional array
GIVEN C++ CODE - WITH GOTO's:
#include <iostream>
using namespace std;
int main() {
int q[8], c=0;
q[0] = 0; // place a queen in row 0 of column 0
int counter = 0; // to count solutions
// c: column as q[c]: row in column c
next_col:
++c; // or c++;
// next column
if(c==8) goto print;
q[c]=-1; // 0
next_row:
++q[c]; // or q[c]++;
// next row
if(q[c]==8) goto backtrack;
for(int i=0; i<c; ++i)
if(q[i]==q[c] || (c-i)== abs(q[c]-q[i])) // || = row test OR diagonal tests
goto next_row;
goto next_col;
backtrack:
--c; // or c--
if(c==-1) // once we backtrack to column -1, we have all the solutions.
return 0; // ends the program
goto next_row;
print:
counter++; // new solutions
cout << counter << ":" << endl;
for(int i=0; i<8; i++)
cout << q[i];
cout << "\n" << endl;
goto backtrack;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

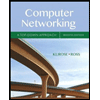
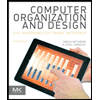
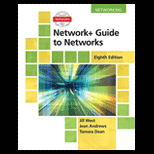
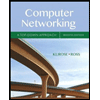
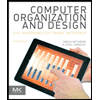
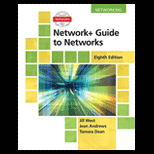
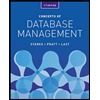
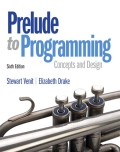
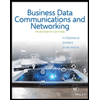