public class ForgetfulCritter extends Critter XxX private String event; public void addHistory (String event) { event = "?"; super.addHistory(); }
public class ForgetfulCritter extends Critter XxX private String event; public void addHistory (String event) { event = "?"; super.addHistory(); }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
JAVA
What can I change to make it work?

Transcribed Image Text:### ForgetfulCritter Class Code Explanation
The displayed code is a Java class named `ForgetfulCritter`, which extends another class named `Critter`. This means that `ForgetfulCritter` is a subclass and inherits properties and methods from the `Critter` class.
#### Key Elements of the Code:
1. **Class Declaration**:
```java
public class ForgetfulCritter extends Critter
```
- `public class ForgetfulCritter`: Declares the class `ForgetfulCritter`.
- `extends Critter`: Indicates inheritance from the `Critter` class.
2. **Private String Field**:
```java
private String event;
```
- Declares a private field `event` of type `String`. Being private means it can only be accessed within this class.
3. **Method: addHistory**:
```java
public void addHistory(String event){
event = "?";
super.addHistory();
}
```
- **Method Declaration**:
- `public void addHistory(String event)`: A public method with no return value (`void`) that takes a `String` parameter named `event`.
- **Parameter Shadowing**:
- `event = "?";`: Assigns the string `"?"` to the local parameter `event`, which shadows the class field `event`. The class field `event` remains unaffected due to shadowing.
- **Superclass Method Call**:
- `super.addHistory();`: Calls the `addHistory` method from the superclass `Critter`. This suggests that `Critter` also has an `addHistory` method.
### Educational Insights:
- **Inheritance in Java**: Demonstrates basic inheritance with the `extends` keyword.
- **Method Overriding and Use of `super`**: Shows how to override a method and use `super` to call the superclass's version of the method.
- **Variable Shadowing**: Illustrates how parameter shadowing occurs when a method's parameter has the same name as a class's field.
![```java
public class ForgetfulCritterTester
{
public static void main(String[] args)
{
Critter dopey = new ForgetfulCritter();
dopey.addHistory("eat");
System.out.println(dopey.getHistory());
System.out.println("Expected: [?]");
dopey.move(10);
System.out.println(dopey.getHistory());
System.out.println("Expected: [?, ?]");
}
}
```
### Explanation:
- **Class Definition**: The code defines a class called `ForgetfulCritterTester`.
- **Main Method**: The `main` method is the entry point of the application.
- **Object Instantiation**: A `Critter` object named `dopey` is created as an instance of `ForgetfulCritter`.
- **addHistory Method**: The method `addHistory("eat")` is called on `dopey`, presumably to log or record an activity.
- **Output**: The program prints the current history of `dopey` using `System.out.println(dopey.getHistory())`, followed by an expected output statement `Expected: [?]`.
- **move Method**: The `move(10)` method is called on `dopey`, likely indicating a movement action.
- **Final Output**: The history is printed again, expected to show two entries, indicated by `Expected: [?, ?]`.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1580620b-ec3d-48da-8d5f-e0e1d6dd1757%2Fc39c4d5b-8c82-43a9-99d2-8dc5e403eb5c%2F0vxmusp_processed.png&w=3840&q=75)
Transcribed Image Text:```java
public class ForgetfulCritterTester
{
public static void main(String[] args)
{
Critter dopey = new ForgetfulCritter();
dopey.addHistory("eat");
System.out.println(dopey.getHistory());
System.out.println("Expected: [?]");
dopey.move(10);
System.out.println(dopey.getHistory());
System.out.println("Expected: [?, ?]");
}
}
```
### Explanation:
- **Class Definition**: The code defines a class called `ForgetfulCritterTester`.
- **Main Method**: The `main` method is the entry point of the application.
- **Object Instantiation**: A `Critter` object named `dopey` is created as an instance of `ForgetfulCritter`.
- **addHistory Method**: The method `addHistory("eat")` is called on `dopey`, presumably to log or record an activity.
- **Output**: The program prints the current history of `dopey` using `System.out.println(dopey.getHistory())`, followed by an expected output statement `Expected: [?]`.
- **move Method**: The `move(10)` method is called on `dopey`, likely indicating a movement action.
- **Final Output**: The history is printed again, expected to show two entries, indicated by `Expected: [?, ?]`.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
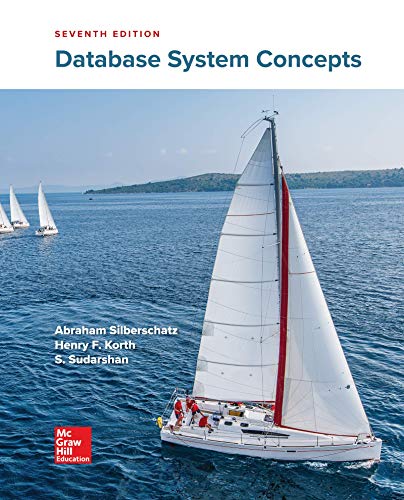
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
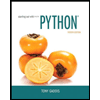
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
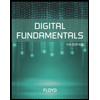
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
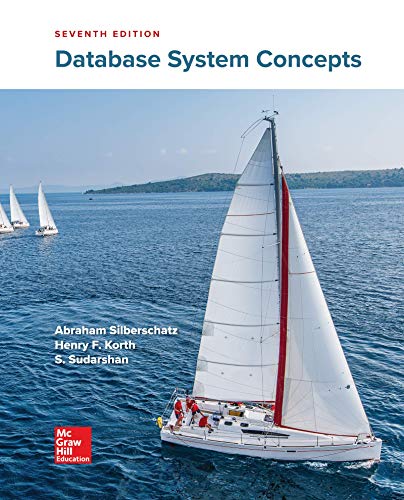
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
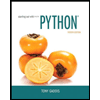
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
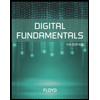
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
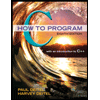
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
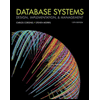
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
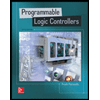
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education