In Java Design and implement a program that implements the following 3 methods: - Method isValid(...) returns true if the sum of the width and height is greater than 30 boolean isValid(double width, double height){} - Method area(...) returns the area of the rectangle if it is a valid rectangle double area(double width, double height){} - Method perimeter(...) returns the perimeter of the rectangle if it is a valid rectangle double perimeter(double width, double height){} Always remember that in Java and C#, the methods must be public and static The main method should prompt the user to enter the width and height of a rectangle ( double values ) and uses the methods to print out a message followed by the area and perimeter if the rectangle is valid. Otherwise, it prints out only the message “ This is an invalid rectangle .” Note: that method isValid(...)is used to validate the input before attempting to compute the area and perimeter. Design the main method in the test program such that it allows the user to re-run the program with different inputs (using a sentinel loop structure). In Java Remember, the class name should be Lab10B. The user input is indicated in bold. Sample output: Enter width: 4.0 Enter height: 5.0 This is an invalid rectangle Do you want to enter another width and height (Y/N)? : Y Enter width: 20.0 Enter height: 15.0 This is a valid rectangle The area is: 300.0 The perimeter is: 70.0 Do you want to enter another width and height (Y/N)? : N Program Ends
In Java
Design and implement a program that implements the following 3 methods:
- Method isValid(...) returns true if the sum of the width and height is greater than 30
boolean isValid(double width, double height){}
- Method area(...) returns the area of the rectangle if it is a valid rectangle
double area(double width, double height){}
- Method perimeter(...) returns the perimeter of the rectangle if it is a valid rectangle
double perimeter(double width, double height){}
Always remember that in Java and C#, the methods must be public and static
The main method should prompt the user to enter the width and height of a rectangle ( double values )
and uses the methods to print out a message followed by the area and perimeter if the rectangle is
valid. Otherwise, it prints out only the message “ This is an invalid rectangle .”
Note: that method isValid(...)is used to validate the input before attempting to compute the area
and perimeter.
Design the main method in the test program such that it allows the user to re-run the program
with different inputs (using a sentinel loop structure).
In Java
Remember, the class name should be Lab10B.
The user input is indicated in bold.
Sample output:
Enter width: 4.0
Enter height: 5.0
This is an invalid rectangle
Do you want to enter another width and height (Y/N)? : Y
Enter width: 20.0
Enter height: 15.0
This is a valid rectangle
The area is: 300.0
The perimeter is: 70.0
Do you want to enter another width and height (Y/N)? : N
Program Ends

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

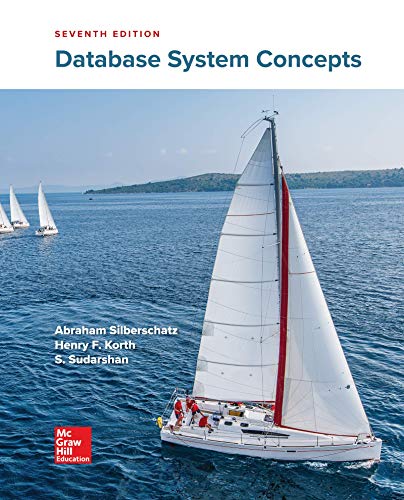
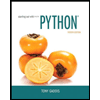
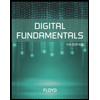
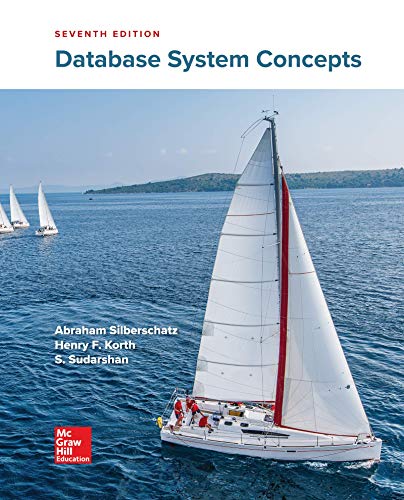
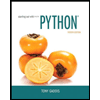
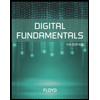
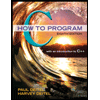
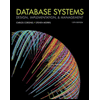
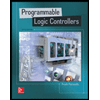