Please visit htt ps://en.wikipedia.org/wiki/Caesar cipher. You will be writing code that encrpyts and decrypts using this method. Specifically, you'll be writing functions: E„(x) (x+ n) mod 27 D„(1) (1 – n) mod 27 On the Wiki page, the modulus (%) is 26, but we're using 27-why? We are adding an extra symbol { for space. Please visit https://en.wikipedia.org/wiki/ASCII If you look at the printable ASCII characters, you'll notice that { (hex value 7A) follows z. Thus we can easily extend our cypher to include this symbol for space. Let's see how. 1 sentence "4his is a secret message about the class" 2 sentence.replace (" ", "(") sentence 3 print (sentence) 4 es - for i in sentence: es - encrypt(i, 5) 7 print (es) 6 8 9 ds - 10 for i in es : ds - decrypt (i, 5) 11 12 13 o_sentence - ds. replace ("(", " ") 14 print (o sentence) has output 1 this {is {a{seeret {message{about {the {class 2 ymnxenxefexjhwjycrjxxfljefgtzycy mjchqfxx 3 this is a seeret message about the class In this cypher we are shifting by five. Look at the first letter t. Here is the shift in Python: 1 >>> ord ('L') 2 116 3 >>> chr (ord ('t') + 5) 4 'y' 5 > chr (ord ('h') + 5) 6 m' Line one is our original sentence with { replacing space. Line two is the encrypted sentence. Line 3 the decrypted sentence. The shift is five, so we replace t' with 'y' and 'h' with 'm'. What
Please visit htt ps://en.wikipedia.org/wiki/Caesar cipher. You will be writing code that encrpyts and decrypts using this method. Specifically, you'll be writing functions: E„(x) (x+ n) mod 27 D„(1) (1 – n) mod 27 On the Wiki page, the modulus (%) is 26, but we're using 27-why? We are adding an extra symbol { for space. Please visit https://en.wikipedia.org/wiki/ASCII If you look at the printable ASCII characters, you'll notice that { (hex value 7A) follows z. Thus we can easily extend our cypher to include this symbol for space. Let's see how. 1 sentence "4his is a secret message about the class" 2 sentence.replace (" ", "(") sentence 3 print (sentence) 4 es - for i in sentence: es - encrypt(i, 5) 7 print (es) 6 8 9 ds - 10 for i in es : ds - decrypt (i, 5) 11 12 13 o_sentence - ds. replace ("(", " ") 14 print (o sentence) has output 1 this {is {a{seeret {message{about {the {class 2 ymnxenxefexjhwjycrjxxfljefgtzycy mjchqfxx 3 this is a seeret message about the class In this cypher we are shifting by five. Look at the first letter t. Here is the shift in Python: 1 >>> ord ('L') 2 116 3 >>> chr (ord ('t') + 5) 4 'y' 5 > chr (ord ('h') + 5) 6 m' Line one is our original sentence with { replacing space. Line two is the encrypted sentence. Line 3 the decrypted sentence. The shift is five, so we replace t' with 'y' and 'h' with 'm'. What
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
. You will be writing code that encrypts and decrypts using this method. Specifically, you'll be writing functions:
\[
E_n(x) = (x + n) \mod 27 \\
D_n(x) = (x - n) \mod 27
\]
On the Wiki page, the modulus (%) is 26, but we're using 27—why? We are adding an extra symbol `{` for space. Please visit [https://en.wikipedia.org/wiki/ASCII](https://en.wikipedia.org/wiki/ASCII). If you look at the printable ASCII characters, you’ll notice that `{` (hex value 7A) follows `z`. Thus we can easily extend our cypher to include this symbol for space. Let’s see how.
```python
sentence = "this is a secret message about the class"
_sentence = sentence.replace(" ", "{")
print(_sentence)
cs = ""
for i in _sentence:
es += encrypt(i, 5)
print(es)
ds = ""
for i in es:
ds += decrypt(i, 5)
o_sentence = ds.replace("{", " ")
print(o_sentence)
```
**Output:**
```
1 this{is{a{secret{message{about{the{class
2 ymnxnxefsijhwjertjxfljcfyqjtymjhfqjxx
3 this is a secret message about the class
```
In this cypher we are shifting by five. Look at the first letter 't'. Here is the shift in Python:
```python
>>> ord('t')
116
>>> chr(ord('t') + 5)
'y'
>>> chr(ord('h') + 5)
'm'
```
Line one is our original sentence with `{` replacing space. Line two is the encrypted sentence. Line three is the decrypted sentence. The shift is five, so we replace 't' with 'y' and 'h' with 'm'. What do you notice?](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8e2a772e-1ea3-4d63-a454-a2f39f414658%2Fbffef055-82d6-46e3-9cf7-7c196be5857d%2F8q6q53u_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Please visit [https://en.wikipedia.org/wiki/Caesar_cipher](https://en.wikipedia.org/wiki/Caesar_cipher). You will be writing code that encrypts and decrypts using this method. Specifically, you'll be writing functions:
\[
E_n(x) = (x + n) \mod 27 \\
D_n(x) = (x - n) \mod 27
\]
On the Wiki page, the modulus (%) is 26, but we're using 27—why? We are adding an extra symbol `{` for space. Please visit [https://en.wikipedia.org/wiki/ASCII](https://en.wikipedia.org/wiki/ASCII). If you look at the printable ASCII characters, you’ll notice that `{` (hex value 7A) follows `z`. Thus we can easily extend our cypher to include this symbol for space. Let’s see how.
```python
sentence = "this is a secret message about the class"
_sentence = sentence.replace(" ", "{")
print(_sentence)
cs = ""
for i in _sentence:
es += encrypt(i, 5)
print(es)
ds = ""
for i in es:
ds += decrypt(i, 5)
o_sentence = ds.replace("{", " ")
print(o_sentence)
```
**Output:**
```
1 this{is{a{secret{message{about{the{class
2 ymnxnxefsijhwjertjxfljcfyqjtymjhfqjxx
3 this is a secret message about the class
```
In this cypher we are shifting by five. Look at the first letter 't'. Here is the shift in Python:
```python
>>> ord('t')
116
>>> chr(ord('t') + 5)
'y'
>>> chr(ord('h') + 5)
'm'
```
Line one is our original sentence with `{` replacing space. Line two is the encrypted sentence. Line three is the decrypted sentence. The shift is five, so we replace 't' with 'y' and 'h' with 'm'. What do you notice?

Transcribed Image Text:The text discusses encryption and decryption methods using a shift cipher approach. It starts by explaining the use of characters, mentioning that the letter 'a' can shift to 'e' by moving five spaces. This technique can be implemented in Python using the `chr` and `ord` functions or by creating a dictionary.
The arguments for the encrypt and decrypt functions are described as \( E_n(x) \) and \( D_n(x) \), each taking two parameters: the letter and the amount of shift. The same shift value is used for both encryption and decryption processes.
A note is made that using a dictionary may simplify the implementation of these functions, though it is left to the reader's discretion.
**Deliverables for Problem 4:**
- Complete the `encrypt` and `decrypt` functions, using `{` to encode spaces.
- The `replace()` function may be utilized.
- A dictionary might make implementing the functions easier.
- Provide docstrings for the functions.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Recommended textbooks for you
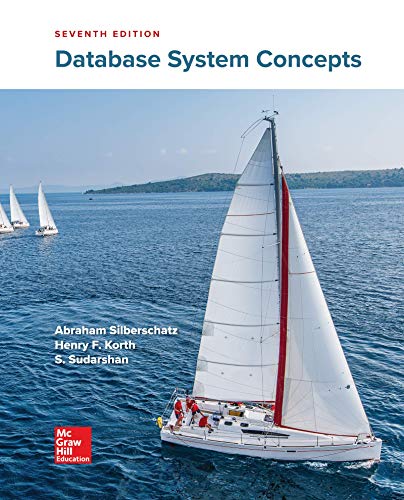
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
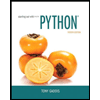
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
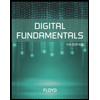
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
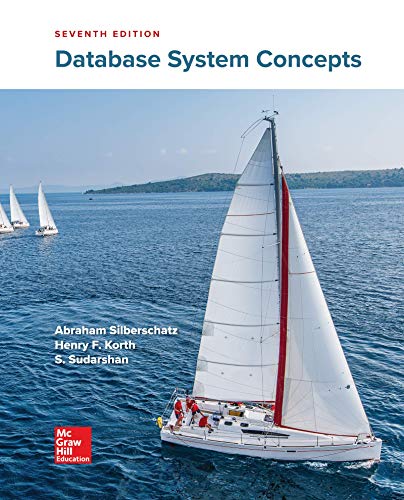
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
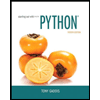
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
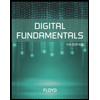
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
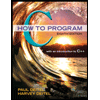
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
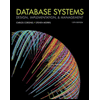
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
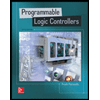
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education