Hello I'm having some trouble with my python code in making a Caesar's cipher. First, I'm only supposed to use one global constant , ALPHABET, but I don't think I can import string. So should I just include every alphabet letter in a bracket ( for ex: [A, B, C....].lower() ) or is there another method? In lines 18 and 19, I have i = ALPHABET.index(char) and encodeMessage += cipher[i] but should I replace the variable i with another variable name, since in line 17, I don't have it listed as , for char in message(i)? Instructions: The main() function will prompt the user for two values: a text message string consisting of only alphabetic characters and spaces, and a key value between 1 and 26. You will have one global constant list called ALPHABET, which will have lowercase letters and the space (in the order shown above) as elements In addition to main, your program will have 3 functions: The generateCipher() function will accept the parameter key and will generate and return the cipher alphabet list. Hint: create the cipher list by slicing the plaintext alphabet list (using the key), and return it.
Hello I'm having some trouble with my python code in making a Caesar's cipher. First, I'm only supposed to use one global constant , ALPHABET, but I don't think I can import string. So should I just include every alphabet letter in a bracket ( for ex: [A, B, C....].lower() ) or is there another method? In lines 18 and 19, I have i = ALPHABET.index(char) and encodeMessage += cipher[i] but should I replace the variable i with another variable name, since in line 17, I don't have it listed as , for char in message(i)? Instructions: The main() function will prompt the user for two values: a text message string consisting of only alphabetic characters and spaces, and a key value between 1 and 26. You will have one global constant list called ALPHABET, which will have lowercase letters and the space (in the order shown above) as elements In addition to main, your program will have 3 functions: The generateCipher() function will accept the parameter key and will generate and return the cipher alphabet list. Hint: create the cipher list by slicing the plaintext alphabet list (using the key), and return it.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Hello I'm having some trouble with my python code in making a Caesar's cipher. First, I'm only supposed to use one global constant , ALPHABET, but I don't think I can import string. So should I just include every alphabet letter in a bracket ( for ex: [A, B, C....].lower() ) or is there another method?
In lines 18 and 19, I have i = ALPHABET.index(char) and encodeMessage += cipher[i] but should I replace the variable i with another variable name, since in line 17, I don't have it listed as , for char in message(i)?
Instructions:
- The main() function will prompt the user for two values: a text message string consisting of only alphabetic characters and spaces, and a key value between 1 and 26.
- You will have one global constant list called ALPHABET, which will have lowercase letters and the space (in the order shown above) as elements
- In addition to main, your program will have 3 functions:
- The generateCipher() function will accept the parameter key and will generate and return the cipher alphabet list. Hint: create the cipher list by slicing the plaintext alphabet list (using the key), and return it.
- The encodeMessage () function will accept as parameters a string containing the plaintext message and the cipher alphabet list, and will return a string with the encoded message
- The decodeMessage () function will accept as parameters a string containing an encoded message and the cipher alphabet list, and will return a string with the plaintext message. If the original message contained uppercase letters, those will NOT be restored (they will be shown in lowercase).
- You may not use any global variables other than the constant(s) mentioned above

Transcribed Image Text:Your program will take as input a plaintext message string consisting only of letters and spaces, and a numeric key value between 1 and 26. The program will display these input values, encode and output the ciphered message, and decode the encoded message back to plain text. A sample execution is shown below:
```
Enter the message you want to encode: my secret
Enter the key: 6
Encoding message 'my secret' with key 6
Encoded: gsuzxlzn
Decoded: my secret
```
![```python
# The purpose of the program is to depict messages using a Caesar's cipher
import string
ALPHABET = string.ascii_lowercase
def generateCipher(key_value):
cipher = ALPHABET[-key_value:] + ALPHABET[:-key_value]
return cipher
def encodeMessage(message, cipher):
encodeMessage = ""
for char in message:
i = ALPHABET.index(char)
encodeMessage += cipher[i]
return encodeMessage
def decodeMessage(encodeMessage, cipher):
decodeMessage = ""
for char in encodeMessage:
i = cipher.index(char)
decodeMessage += ALPHABET[i]
return decodeMessage
def main():
message = input("Enter the message you want to encode: ").lower()
key_value = int(input("Enter the key: "))
if key_value < 1 or key_value > 26:
key_value = int(input("Invalid key value. Try again: "))
cipher = generateCipher(key_value)
encodeMessage = encodeMessage(message, cipher)
print("Encoded: " + encode)
decodeMessage = decodeMessage(encodeMessage, cipher)
print("Decoded: " + decode)
main()
```
### Explanation
This Python script is designed to encode and decode messages using a Caesar cipher. The Caesar cipher is a type of substitution cipher where each letter in the plaintext is shifted a certain number of places down the alphabet.
**Functions:**
1. **`generateCipher(key_value)`:**
- Generates a cipher alphabet by shifting the original alphabet by the `key_value`.
- `key_value` determines how many positions each letter in the alphabet is shifted.
2. **`encodeMessage(message, cipher)`:**
- Encodes a message using the generated cipher.
- Converts each character in the message into its corresponding character in the cipher.
3. **`decodeMessage(encodeMessage, cipher)`:**
- Decodes an encoded message back into plaintext.
- Converts each character in the encoded message to the original alphabet character using the cipher.
4. **`main()`:**
- Takes input from the user for the message and the key value.
- Checks the validity of the key (should be between 1 and 26).
- Generates the cipher and encodes the message.
- Prints the encoded and decoded message.
The script uses Python's `string` library to](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3eb03a80-7cae-4d33-b228-e2e0361c370e%2F62952648-cf20-4b86-919b-4556a0fa02b7%2Fvir8g5b_processed.png&w=3840&q=75)
Transcribed Image Text:```python
# The purpose of the program is to depict messages using a Caesar's cipher
import string
ALPHABET = string.ascii_lowercase
def generateCipher(key_value):
cipher = ALPHABET[-key_value:] + ALPHABET[:-key_value]
return cipher
def encodeMessage(message, cipher):
encodeMessage = ""
for char in message:
i = ALPHABET.index(char)
encodeMessage += cipher[i]
return encodeMessage
def decodeMessage(encodeMessage, cipher):
decodeMessage = ""
for char in encodeMessage:
i = cipher.index(char)
decodeMessage += ALPHABET[i]
return decodeMessage
def main():
message = input("Enter the message you want to encode: ").lower()
key_value = int(input("Enter the key: "))
if key_value < 1 or key_value > 26:
key_value = int(input("Invalid key value. Try again: "))
cipher = generateCipher(key_value)
encodeMessage = encodeMessage(message, cipher)
print("Encoded: " + encode)
decodeMessage = decodeMessage(encodeMessage, cipher)
print("Decoded: " + decode)
main()
```
### Explanation
This Python script is designed to encode and decode messages using a Caesar cipher. The Caesar cipher is a type of substitution cipher where each letter in the plaintext is shifted a certain number of places down the alphabet.
**Functions:**
1. **`generateCipher(key_value)`:**
- Generates a cipher alphabet by shifting the original alphabet by the `key_value`.
- `key_value` determines how many positions each letter in the alphabet is shifted.
2. **`encodeMessage(message, cipher)`:**
- Encodes a message using the generated cipher.
- Converts each character in the message into its corresponding character in the cipher.
3. **`decodeMessage(encodeMessage, cipher)`:**
- Decodes an encoded message back into plaintext.
- Converts each character in the encoded message to the original alphabet character using the cipher.
4. **`main()`:**
- Takes input from the user for the message and the key value.
- Checks the validity of the key (should be between 1 and 26).
- Generates the cipher and encodes the message.
- Prints the encoded and decoded message.
The script uses Python's `string` library to
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
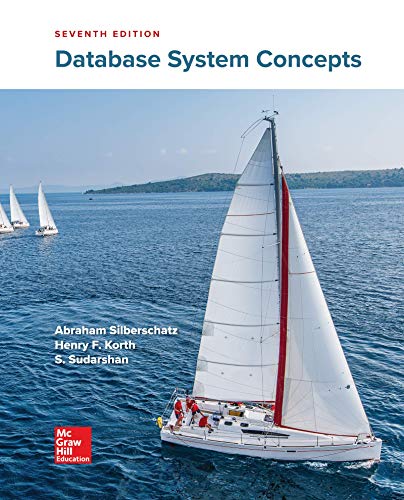
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
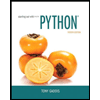
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
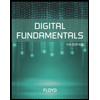
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
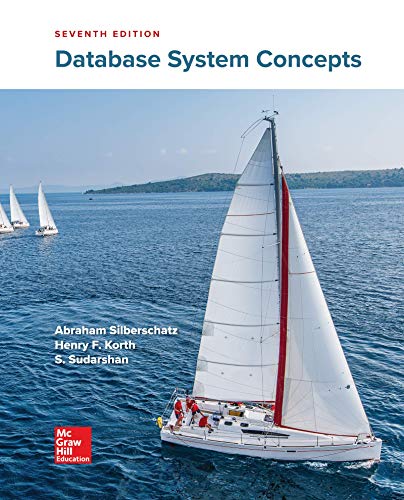
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
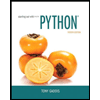
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
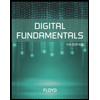
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
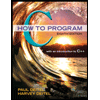
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
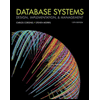
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
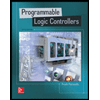
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education