get Write a test for the get] method. Make sure to test the cases where [get receives an invalid argument, e.g. [get (28723) when the Deque] only has 1 item, or a negative index. In these cases get should return null. You should disregard the skeleton code comments for Deque.java] and take spec as your primary point. get must use iteration. Task: After you've written tests and verified that they fail, implement [get]
Write tests and implement
![get
Write a test for the [get method. Make sure to test the cases where [get] receives an invalid argument,
e.g.get(28723) when the Deque only has 1 item, or a negative index. In these cases get should return
null. You should disregard the skeleton code comments for Deque.java and take spec as your primary
point.
get must use iteration.
Task: After you've written tests and verified that they fail, implement [get
getRecursive #
Since we're working with a linked list, it is interesting to write a recursive get method, [getRecursive
Copy and paste your tests for the [get method so that they are the same except they call getRecursive
(While there is a way to avoid having copy and pasted tests, though the syntax is not worth introducing-
passing around functions in love is
in](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffabd8e49-6e36-4259-b8d2-259afe4bcbb1%2Fbf03d9a4-0e33-426b-b8af-4e5e043313de%2Fy54nwtb_processed.jpeg&w=3840&q=75)

Here is a sample test case for the get method using iteration:
public void testGetWithIteration() {
// Create a new Deque object
Deque deque = new Deque();
// Add three items to the Deque object
deque.addFirst(1);
deque.addLast(2);
deque.addLast(3);
// Assert that the get method returns the expected values for valid indices
assertEquals(1, deque.get(0));
assertEquals(2, deque.get(1));
assertEquals(3, deque.get(2));
// Test the case where the get method receives an invalid index (larger than the size of the Deque)
try {
deque.get(3);
fail("Should have thrown an exception.");
} catch (IndexOutOfBoundsException e) {
// expected
}
// Test the case where the get method receives a negative index
try {
deque.get(-1);
fail("Should have thrown an exception.");
} catch (IndexOutOfBoundsException e) {
// expected
}
}
Step by step
Solved in 2 steps

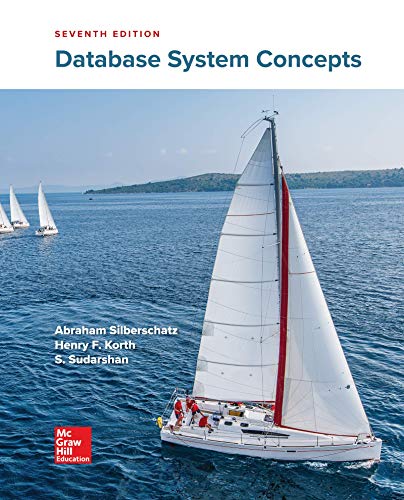
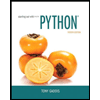
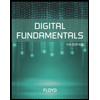
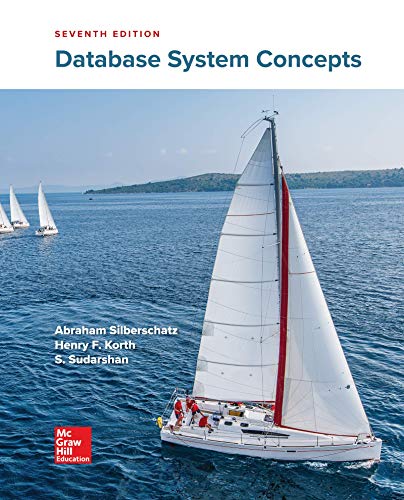
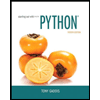
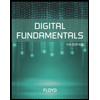
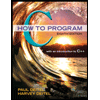
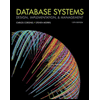
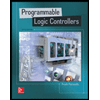