Please help me with this code becuase I dont think my output is correct. QUENEMAIN.CPP #include #include #include #include #include #include #include "LinkedQueue.h" using namespace std; /** * Name of input file containing officer names */ const char INPUT_FILE[] = "Alamo.csv"; /** * Inputs data from a text file and places each officer's name into the * queue passed as a parameter. * @param officers - dynamic queue of Confederate officers in the Civil War */ void loadQueue(LinkedQueue &officers); /** * Displays a list of officers removed from the queue and also displays * the name of the officer going for help. Retrieves officers from the * officers queue, one at a time, counting as each is retrieved. If the * count is not equal to hatNumber, the officer is put back into the * queue. When the count reaches hatNumber, that officer is removed from * the queue permanently (i.e., not put back into the queue), and the * officer's name is output to the screen. The last officer remaining in * the queue is the one shown as going for help. * @param officers - dynamic queue of Confederate officers in the Civil War * @param hatNumber - the number drawn from the hat, used to count off */ void countOff(LinkedQueue officers, int hatNumber); int main(void) { ifstream inFile(INPUT_FILE); // declare and open the input file int count; // number used for counting off LinkedQueue officers; // queue containing officer names string officerName; // name of officer in front of queue string firstName; // name of first officer in original queue loadQueue(officers); // Display list of officers in original queue cout << "_______________________________" << endl; cout << "OFFICERS IN THE ORIGINAL QUEUE: " << endl; cout << "-------------------------------" << endl; firstName = officers.peekFront(); cout << firstName << endl; officers.dequeue(); officers.enqueue(firstName); officerName = officers.peekFront(); while (firstName != officerName) { cout << officerName << endl; officers.dequeue(); officers.enqueue(officerName); officerName = officers.peekFront(); } // Test countOff() method using various numbers drawn from the hat count = 4; cout << endl << endl << "COUNT IS: " << count << endl; countOff(officers, count); // count = 8; // cout << endl << endl << "COUNT IS: " << count << endl; // countOff(officers, count); return EXIT_SUCCESS; } void loadQueue(LinkedQueue &officers) { ifstream inFile(INPUT_FILE); // declare and open the input file string officerName; // name of officer input from file if (!inFile) cout << "Error opening file for input: " << INPUT_FILE << endl; else { getline(inFile, officerName); //read https address getline(inFile, officerName); //read headers getline(inFile, officerName); //reads first name while (!inFile.eof()) { officers.enqueue(officerName); getline(inFile, officerName); } inFile.close(); } } void countOff(LinkedQueue officers, int count) { //write your code here }
Please help me with this code becuase I dont think my output is correct.
QUENEMAIN.CPP
#include <iostream>
#include <fstream>
#include <cstdlib>
#include <string>
#include <stdexcept>
#include <cassert>
#include "LinkedQueue.h"
using namespace std;
/**
* Name of input file containing officer names
*/
const char INPUT_FILE[] = "Alamo.csv";
/**
* Inputs data from a text file and places each officer's name into the
* queue passed as a parameter.
* @param officers - dynamic queue of Confederate officers in the Civil War
*/
void loadQueue(LinkedQueue<string> &officers);
/**
* Displays a list of officers removed from the queue and also displays
* the name of the officer going for help. Retrieves officers from the
* officers queue, one at a time, counting as each is retrieved. If the
* count is not equal to hatNumber, the officer is put back into the
* queue. When the count reaches hatNumber, that officer is removed from
* the queue permanently (i.e., not put back into the queue), and the
* officer's name is output to the screen. The last officer remaining in
* the queue is the one shown as going for help.
* @param officers - dynamic queue of Confederate officers in the Civil War
* @param hatNumber - the number drawn from the hat, used to count off
*/
void countOff(LinkedQueue<string> officers, int hatNumber);
int main(void) {
ifstream inFile(INPUT_FILE); // declare and open the input file
int count; // number used for counting off
LinkedQueue<string> officers; // queue containing officer names
string officerName; // name of officer in front of queue
string firstName; // name of first officer in original queue
loadQueue(officers);
// Display list of officers in original queue
cout << "_______________________________" << endl;
cout << "OFFICERS IN THE ORIGINAL QUEUE: " << endl;
cout << "-------------------------------" << endl;
firstName = officers.peekFront();
cout << firstName << endl;
officers.dequeue();
officers.enqueue(firstName);
officerName = officers.peekFront();
while (firstName != officerName) {
cout << officerName << endl;
officers.dequeue();
officers.enqueue(officerName);
officerName = officers.peekFront();
}
// Test countOff() method using various numbers drawn from the hat
count = 4;
cout << endl << endl << "COUNT IS: " << count << endl;
countOff(officers, count);
// count = 8;
// cout << endl << endl << "COUNT IS: " << count << endl;
// countOff(officers, count);
return EXIT_SUCCESS;
}
void loadQueue(LinkedQueue<string> &officers) {
ifstream inFile(INPUT_FILE); // declare and open the input file
string officerName; // name of officer input from file
if (!inFile)
cout << "Error opening file for input: " << INPUT_FILE << endl;
else {
getline(inFile, officerName); //read https address
getline(inFile, officerName); //read headers
getline(inFile, officerName); //reads first name
while (!inFile.eof()) {
officers.enqueue(officerName);
getline(inFile, officerName);
}
inFile.close();
}
}
void countOff(LinkedQueue<string> officers, int count)
{
//write your code here
}



Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

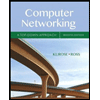
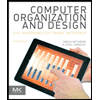
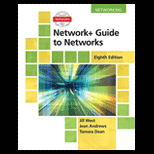
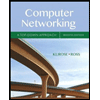
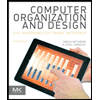
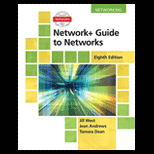
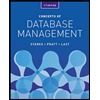
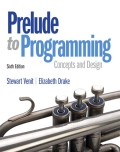
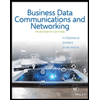