Need Help with this code completion. class neural_network: def __init__(self,Nhidden,Ninput,Noutput,Nonlinearities): self.layer = [] # BUILD THE NETWORK # Add a linear layer with Ninput input features and Nhidden output features # YOUR CODE HERE # Add a non-linearity if(Nonlinearities[0]=='relu'): self.layer.append(relu()) else: self.layer.append(sigmoid()) # Add a linear layer # YOUR CODE HERE # Add a non-linearity def forward(self,X): # Forward propagation # YOUR CODE HERE return X def backward(self,dY): # Backward propagation # YOUR CODE HERE return def update(self, learning_rate): # Updating the parameters of the network according to algorithm # YOUR CODE HERE self.layer[0].W = self.layer[0].b = self.layer[2].W = self.layer[2].b =
Need Help with this code completion.
class neural_network:
def __init__(self,Nhidden,Ninput,Noutput,Nonlinearities):
self.layer = []
# BUILD THE NETWORK
# Add a linear layer with Ninput input features and Nhidden output features
# YOUR CODE HERE
# Add a non-linearity
if(Nonlinearities[0]=='relu'):
self.layer.append(relu())
else:
self.layer.append(sigmoid())
# Add a linear layer
# YOUR CODE HERE
# Add a non-linearity
def forward(self,X):
# Forward propagation
# YOUR CODE HERE
return X
def backward(self,dY):
# Backward propagation
# YOUR CODE HERE
return
def update(self, learning_rate):
# Updating the parameters of the network according to
# YOUR CODE HERE
self.layer[0].W =
self.layer[0].b =
self.layer[2].W =
self.layer[2].b =

Here's the completed code with comments explaining each step:
CODE in Python:
class neural_network:
def __init__(self, Nhidden, Ninput, Noutput, Nonlinearities):
self.layer = []
# BUILD THE NETWORK
# Add a linear layer with Ninput input features and Nhidden output features
# YOUR CODE HERE
self.layer.append(linear(Ninput, Nhidden))
# Add a non-linearity
if Nonlinearities[0] == 'relu':
self.layer.append(relu())
else:
self.layer.append(sigmoid())
# Add a linear layer with Nhidden input features and Noutput output features
# YOUR CODE HERE
self.layer.append(linear(Nhidden, Noutput))
# Add a non-linearity
if Nonlinearities[1] == 'relu':
self.layer.append(relu())
else:
self.layer.append(sigmoid())
def forward(self, X):
# Forward propagation
# YOUR CODE HERE
for layer in self.layer:
X = layer.forward(X)
return X
def backward(self, dY):
# Backward propagation
# YOUR CODE HERE
for layer in reversed(self.layer):
dY = layer.backward(dY)
return dY
def update(self, learning_rate):
# Updating the parameters of the network according to algorithm
# YOUR CODE HERE
self.layer[0].W = self.layer[0].W - learning_rate * self.layer[0].dW
self.layer[0].b = self.layer[0].b - learning_rate * self.layer[0].db
self.layer[2].W = self.layer[2].W - learning_rate * self.layer[2].dW
self.layer[2].b = self.layer[2].b - learning_rate * self.layer[2].db
Step by step
Solved in 2 steps

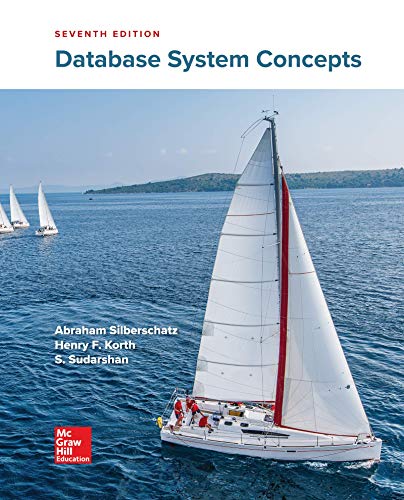
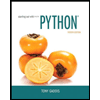
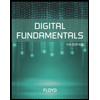
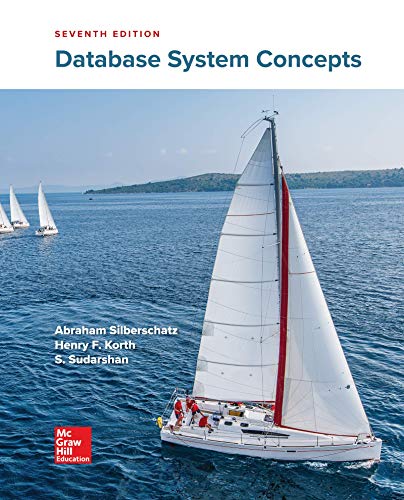
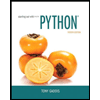
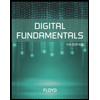
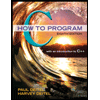
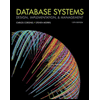
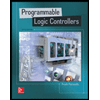