In java please Create a splice method for the implentation perspective. The 2nd queue shouuld not be altered when method is done. method header: public void splice(ArrayQueue secondQueue)
In java please Create a splice method for the implentation perspective. The 2nd queue shouuld not be altered when method is done. method header: public void splice(ArrayQueue secondQueue)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In java please
Create a splice method for the implentation perspective.
The 2nd queue shouuld not be altered when method is done.
method header:
public void splice(ArrayQueue<T> secondQueue)
![public void clear() {
if (!isEmpty(0) { // deallocates only the used portion
for (int index = frontIndex; index != backIndex; index =
55
56
57
(index +
1)
58
% queue.length) {
queue [index] = null;
}
queue [backIndex]
}
frontIndex = 0;
59
60
61
null;
62
63
64
backIndex
queue. length
1;
65
}
66
private boolean isArrayFull) {
return frontIndex
}
67
68
((backIndex + 2) % queue.length);
69
70
private void doubleArray() {
TI] oldQueue
int oldSize
71
72
queue;
oldQueue. length;
73
74
75
queue
(T[]) new Object[2 * oldSize];
76
1; index++) {
for (int index =
queue [index]
frontIndex =
}
0; index < oldSize
oldQueue [frontIndex];
(frontIndex + 1) % oldSize;
77
78
79
80
81
frontIndex = 0;
backIndex = oldSize
}
82
83
2;
84
85
86
public void display() {
87
for(int i=frontIndex; i!=(backIndex+1)%queue. length; i=(i+1)°%queue.length) {
I data = queue[i];
System.out.print(data +
88
89
90
" ");
91
System.out.println();
}
92
93
94
public void splice(ArrayQueue<T> anotherQueue) {
// YOUR CODE HERE!
}
95
96
97
98
public T getSecond() {
// YOUR EXTRA CREDIT CODE HERE!
return null; // placeholder: replace with your own code
}
99
100
101
102
103
104
105](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fff91ef73-4530-48ba-be88-fd15c629792d%2F8d25b61d-e986-4da5-9f95-bef83e4eeccc%2Fib7r8k_processed.png&w=3840&q=75)
Transcribed Image Text:public void clear() {
if (!isEmpty(0) { // deallocates only the used portion
for (int index = frontIndex; index != backIndex; index =
55
56
57
(index +
1)
58
% queue.length) {
queue [index] = null;
}
queue [backIndex]
}
frontIndex = 0;
59
60
61
null;
62
63
64
backIndex
queue. length
1;
65
}
66
private boolean isArrayFull) {
return frontIndex
}
67
68
((backIndex + 2) % queue.length);
69
70
private void doubleArray() {
TI] oldQueue
int oldSize
71
72
queue;
oldQueue. length;
73
74
75
queue
(T[]) new Object[2 * oldSize];
76
1; index++) {
for (int index =
queue [index]
frontIndex =
}
0; index < oldSize
oldQueue [frontIndex];
(frontIndex + 1) % oldSize;
77
78
79
80
81
frontIndex = 0;
backIndex = oldSize
}
82
83
2;
84
85
86
public void display() {
87
for(int i=frontIndex; i!=(backIndex+1)%queue. length; i=(i+1)°%queue.length) {
I data = queue[i];
System.out.print(data +
88
89
90
" ");
91
System.out.println();
}
92
93
94
public void splice(ArrayQueue<T> anotherQueue) {
// YOUR CODE HERE!
}
95
96
97
98
public T getSecond() {
// YOUR EXTRA CREDIT CODE HERE!
return null; // placeholder: replace with your own code
}
99
100
101
102
103
104
105
![1
public class ArrayQueue<T> implements QueueInterface<T> {
2
private T[] queue; // circular array of queue entries and one unused location
public int frontIndex;
public int backIndex;
private static final int DEFAULT_INITIAL_CAPACITY
3
4
50;
7
public ArrayQueue() {
this(DEFAULT_INITIAL_CAPACITY);
}
8.
10
11
12
public ArrayQueue(int initialCapacity) {
(T[]) new Object[initialCapacity + 1];
0;
initialCapacity;
13
queue
frontIndex
14
15
backIndex
16
17
18
public void enqueue(T newEntry) {
if (isArrayFull())
doubleArray();
19
20
21
22
backIndex = (backIndex + 1) % queue. length;
queue [backIndex]
}
23
24
newEntry;
25
ir
26
public T getFront() {
I front
27
28
null;
29
if (!isEmpty()) {
front
30
31
queue [frontIndex];
32
}
33
return front;
}
34
35
36
public T dequeue() {
I front = null;
37
38
39
if (!isEmpty()) {
front =
queue [frontIndex] = null;
frontIndex =
}
40
41
queue [frontIndex];
42
43
(frontIndex + 1) % queue.length;
44
45
return front;
}
46
47
48
49
public boolean isEmpty() {
return frontIndex
}
50
51
((backIndex + 1) % queue.length);
52
53
54
public void clear() {
if (!isEmpty(O) { // deallocates only the used portion
for (int index = frontIndex; index != backIndex; index
55
56
57
(index
1)
% queue. length) {
queue [index] = null;
}
queue [backIndex]
58
59
60
61
null;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fff91ef73-4530-48ba-be88-fd15c629792d%2F8d25b61d-e986-4da5-9f95-bef83e4eeccc%2Fr5ey6sj_processed.png&w=3840&q=75)
Transcribed Image Text:1
public class ArrayQueue<T> implements QueueInterface<T> {
2
private T[] queue; // circular array of queue entries and one unused location
public int frontIndex;
public int backIndex;
private static final int DEFAULT_INITIAL_CAPACITY
3
4
50;
7
public ArrayQueue() {
this(DEFAULT_INITIAL_CAPACITY);
}
8.
10
11
12
public ArrayQueue(int initialCapacity) {
(T[]) new Object[initialCapacity + 1];
0;
initialCapacity;
13
queue
frontIndex
14
15
backIndex
16
17
18
public void enqueue(T newEntry) {
if (isArrayFull())
doubleArray();
19
20
21
22
backIndex = (backIndex + 1) % queue. length;
queue [backIndex]
}
23
24
newEntry;
25
ir
26
public T getFront() {
I front
27
28
null;
29
if (!isEmpty()) {
front
30
31
queue [frontIndex];
32
}
33
return front;
}
34
35
36
public T dequeue() {
I front = null;
37
38
39
if (!isEmpty()) {
front =
queue [frontIndex] = null;
frontIndex =
}
40
41
queue [frontIndex];
42
43
(frontIndex + 1) % queue.length;
44
45
return front;
}
46
47
48
49
public boolean isEmpty() {
return frontIndex
}
50
51
((backIndex + 1) % queue.length);
52
53
54
public void clear() {
if (!isEmpty(O) { // deallocates only the used portion
for (int index = frontIndex; index != backIndex; index
55
56
57
(index
1)
% queue. length) {
queue [index] = null;
}
queue [backIndex]
58
59
60
61
null;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
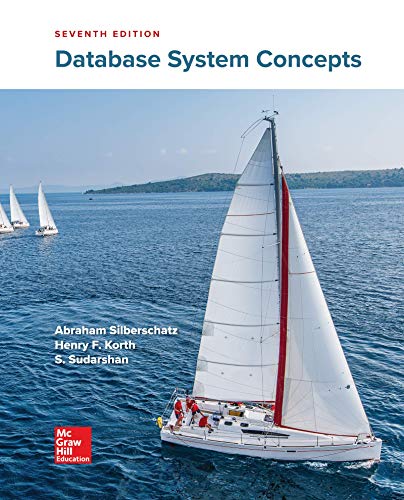
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
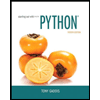
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
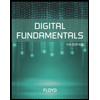
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
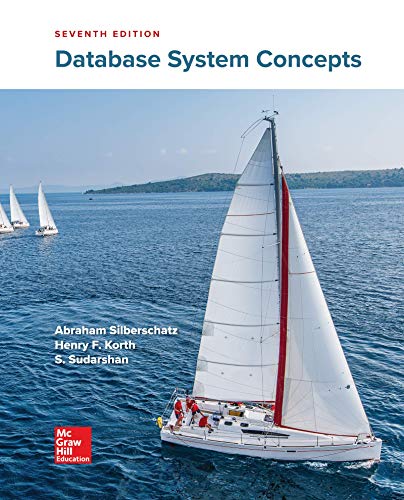
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
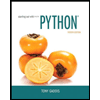
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
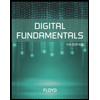
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
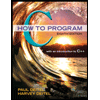
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
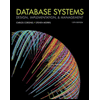
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
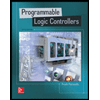
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education