ifndef queueLL_H #define queueLL_H #include class queueLL { private: //defining an inner node class class node { public: //has integer data int data; //reference to next node node* next; //constructor taking value for data field node(int dat) { data = dat; next = NULL; } }; //references to front and rear nodes of the queue node* front, * rear; public: //constructor to initialize empty queue queueLL() { front = NULL; rear = NULL; } ~queueLL() // Deconstructor of queueLL to free space { //continuously dequeueing the queue until empty while (!empty()) { dequeue(); } } // Checks if the received queue is empty bool empty() const { return front == NULL; } //Adds the enqueued item to back of queue void enqueue(int x) { //if queue is empty, creating a new node and saving it as both front //and rear if (empty()) { front = new node(x); rear = front; } //otherwise appending new node as next of rear and updating rear else { rear->next = new node(x); rear = rear->next; } } //Removes and return first item from queue int dequeue() { //taking a reference to front node node* f = front; //advancing front front = front->next; //if front is now NULL, setting rear to NULL too if (front == NULL) { rear = NULL; } //fetching data of f int data = f->data; //deleting f delete f; //returning removed data return data; } }; #endif
How do I make my queueLL.h into a template class and a decimation method?
////////////////////////////
#ifndef queueLL_H
#define queueLL_H
#include<iostream>
class queueLL
{
private:
//defining an inner node class
class node
{
public:
//has integer data
int data;
//reference to next node
node* next;
//constructor taking value for data field
node(int dat) {
data = dat;
next = NULL;
}
};
//references to front and rear nodes of the queue
node* front, * rear;
public:
//constructor to initialize empty queue
queueLL()
{
front = NULL;
rear = NULL;
}
~queueLL() // Deconstructor of queueLL to free space
{
//continuously dequeueing the queue until empty
while (!empty()) {
dequeue();
}
}
// Checks if the received queue is empty
bool empty() const
{
return front == NULL;
}
//Adds the enqueued item to back of queue
void enqueue(int x)
{
//if queue is empty, creating a new node and saving it as both front
//and rear
if (empty()) {
front = new node(x);
rear = front;
}
//otherwise appending new node as next of rear and updating rear
else {
rear->next = new node(x);
rear = rear->next;
}
}
//Removes and return first item from queue
int dequeue()
{
//taking a reference to front node
node* f = front;
//advancing front
front = front->next;
//if front is now NULL, setting rear to NULL too
if (front == NULL) {
rear = NULL;
}
//fetching data of f
int data = f->data;
//deleting f
delete f;
//returning removed data
return data;
}
};
#endif

Code:
#include <iostream>
using namespace std;
typedef int entrytype;
class node
{
public:
entrytype nodedata;
node *next;
node()
{
}
node(entrytype nodedata, node *next = nullptr)
{
this->nodedata = nodedata;
this->next = next;
}
};
class queue
{
private:
node *first;
node *last;
int Size;
public:
// constructor
queue()
{
first = last = nullptr;
Size = 0;
}
// destructor
~queue()
{
node *curr = first;
// iterate over the list and delete the nodes
while (curr != nullptr)
{
node *toDel = curr;
curr = curr->next;
delete toDel;
}
first = last = nullptr; // set the first AND LAST as null
Size = 0; // set the size to zero
}
// method to append a node to the end of the queue
bool append(entrytype value)
{
node *newNode = new node(value);
// return false in case of overflow
if (newNode == nullptr)
{
return false;
}
// if there is no node in the list
if (first == nullptr)
{
first = newNode; // make this the head node
last = first; // this is also the last node
}
// if there is atleast one node in the list
// add it to the end of the list and update the tail
else
{
last->next = newNode;
last = last->next;
}
// update the size
Size++;
return true;
}
// method to access the front element of the queue
bool front(entrytype &value)
{
// if queue is empty : return the underflow error
if (first == nullptr)
{
return false;
}
// else set the value as first element's value
value = first->nodedata;
return true;
}
// method to delete the first element of the queue
bool pop()
{
// if queue is empty : return underflow error code
if (first == nullptr)
{
return false;
}
// else remove the first element of the queue
node *toPop = first; // access the first element
// move first to next node
first = first->next;
// if first became null : it means there was a single node in the list
// so make last null as well.
if (!first)
last = nullptr;
delete toPop; // delete the first node
// update the Size
Size--;
return true; // return true if operation was successful
}
// method to get the current size of the queue
int size()
{
return Size;
}
// method to check if given element exists in the queue
bool find(entrytype target)
{
for (node *curr = first; curr != nullptr; curr = curr->next)
{
if (curr->nodedata == target) // if value is found return true
return true;
}
return false; // return false
}
};
int main()
{
queue *q = new queue; // create a new queue
// add the even elements to the queue
for (entrytype x = 8; x <= 398; x += 2)
{
q->append(x);
}
entrytype frontEl; // to store the front of the queue
// get the front of the queue
q->front(frontEl);
cout << "Front of the queue: " << frontEl << endl;
// remove the first two elements of the queue
cout << "Popped 2 elements from the queue\n";
q->pop();
q->pop();
// check if 8 exists in the queue
cout << "Does 8 exists in the queue? " << q->find(8) << endl;
// check if 200 exists in the queue
cout << "Does 200 exists in the queue? " << q->find(200) << endl;
// report the size of the queue
cout << "Current size of the queue: " << q->size() << endl;
// remove 10 elements from the queue
for (int i = 0; i < 10; i++)
q->pop();
cout << "Popped 10 elements from the queue\n";
// get the new size of the queue
cout << "Updated size of the queue: " << q->size() << endl;
// get the front of the queue
q->front(frontEl);
cout << "Front of the queue: " << frontEl << endl;
return 0;
}
Step by step
Solved in 3 steps with 2 images

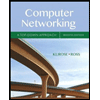
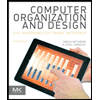
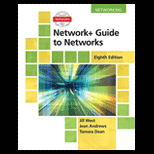
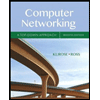
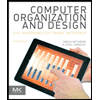
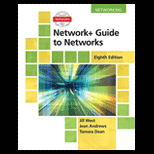
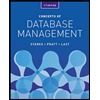
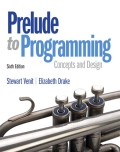
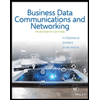