My issue: I've written comments in the code as indicated with "????" of instructions in the problem that I don't know how to code in order to execute. My code: import java.util.Scanner; public class Hailstone { /* Type your code here. */public int hailstone(int num) { int i=1; while(num != 1) { //as the instruction says in the 3rd bullet point "Continue until num is 1" if(num % 2 == 0){ //divisible by an even number num = num/2; //1st bullet point expression assignment } else { //if odd number, then this num = num*3+1; //2nd bullet point expression assignment } i++; //if the number is not 1, it continues //???? I want to add an if statement that stops at 10 digits as specify in the instructions, but don't know how to } System.out.print(num + "\t"); /*???? I'm assuming it would print out the 10 digits, if not I do not how to do so or would it create an infinite loop? }
My issue:
I've written comments in the code as indicated with "????" of instructions in the problem that I don't know how to code in order to execute.
My code:
import java.util.Scanner;
public class Hailstone {
/* Type your code here. */public int hailstone(int num) {
int i=1;
while(num != 1) { //as the instruction says in the 3rd bullet point "Continue until num is 1"
if(num % 2 == 0){ //divisible by an even number
num = num/2; //1st bullet point expression assignment
}
else { //if odd number, then this
num = num*3+1; //2nd bullet point expression assignment
}
i++; //if the number is not 1, it continues
//???? I want to add an if statement that stops at 10 digits as specify in the instructions, but don't know how to
}
System.out.print(num + "\t"); /*???? I'm assuming it would print out the 10 digits, if not I do not how to do so
or would it create an infinite loop?
}
/*my code ends here*/
//code below generated by lab/computer begins here
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
Hailstone labObject = new Hailstone();
int num;
num = scnr.nextInt();
labObject.hailstone(num); // Call hailstone() to print out the hailstone sequence.
}
}


Step by step
Solved in 4 steps with 3 images

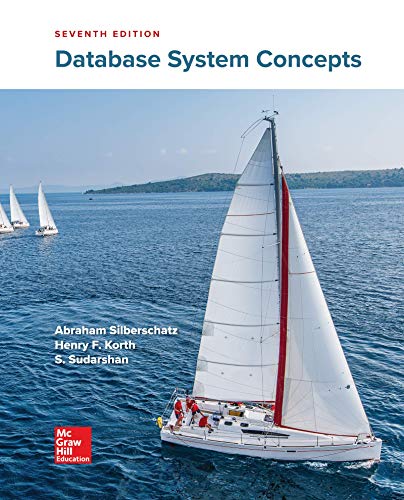
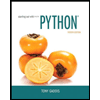
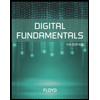
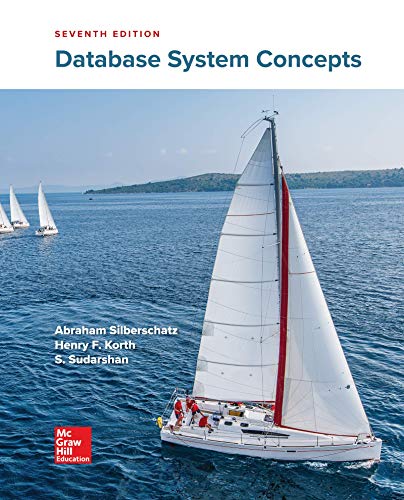
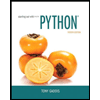
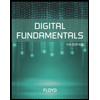
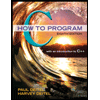
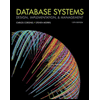
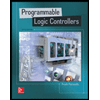