My code is giving me trouble with running FXML. when i click the radio button metric nothing gets printed out when i click the button. i am using scene builder to do this. code is in java. here is the code BMI BUTTON CODE import javafx.beans.value.ChangeListener; import javafx.beans.value.ObservableValue; import javafx.event.ActionEvent; import javafx.fxml.FXML; import javafx.scene.control.Label; import javafx.scene.control.TextField; import javafx.scene.control.Button; import javafx.scene.control.RadioButton; import java.text.DecimalFormat;
My code is giving me trouble with running FXML. when i click the radio button metric nothing gets printed out when i click the button. i am using scene builder to do this. code is in java.
here is the code
BMI BUTTON CODE
import javafx.beans.value.ChangeListener;
import javafx.beans.value.ObservableValue;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.control.Button;
import javafx.scene.control.RadioButton;
import java.text.DecimalFormat;
public class BMICalculateButton
{
@FXML
private Button BMIButton;
@FXML
private TextField weightTextField;
@FXML
private TextField heightTextField;
@FXML
private TextField BMICalculationTextField;
@FXML
private RadioButton EnglishRadioButton;
@FXML
private RadioButton MetricRadioButton;
@FXML
void handleButtonAction(ActionEvent event) {
try {
// To do task: get the input and add to result label
String input1 = weightTextField.getText();
String input2 = heightTextField.getText();
DecimalFormat format = new DecimalFormat(".00");
double weight = Integer.parseInt(input1);
double height = Integer.parseInt(input2);
double BMI;
if(EnglishRadioButton.isSelected())
{
BMI = (weight * 703 )/ (height * height);
}
else if(MetricRadioButton.isSelected())
{
BMI = (weight)/ (height * height);
BMICalculationTextField.setText(String.valueOf(format.format(BMI)));
}
else
{
BMICalculationTextField.setText(String.valueOf(format.format(BMI)));
}
}
catch (NumberFormatException ex) {
weightTextField.setText("");
heightTextField.setText("");
}
}
// called by FXMLLoader to initialize the controller
public void initialize() {
// listener for changes to number1TextField's text
weightTextField.textProperty().addListener(
new ChangeListener<String>() {
@Override
public void changed(ObservableValue<? extends String> ov,
String oldValue, String newValue) {
BMICalculationTextField.setText("");
}
}
);
// listener for changes to number2TextField's text
heightTextField.textProperty().addListener(
new ChangeListener<String>() {
@Override
public void changed(ObservableValue<? extends String> ov,
String oldValue, String newValue) {
BMICalculationTextField.setText("");
}
}
);
}
}
LAUNCHER CODE
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class BMICalculator extends Application {
@Override
public void start(Stage stage) throws Exception {
Parent root =
FXMLLoader.load(getClass().getResource("BMICalculator.fxml"));
Scene scene = new Scene(root); // attach scene graph to scene
stage.setTitle("BMICalculateButton"); // displayed in window's title bar
stage.setScene(scene); // attach scene to stage
stage.show(); // display the stage
}
public static void main(String[] args) {
// create a Addition object and call its start method
launch(args);
}
}

Step by step
Solved in 2 steps

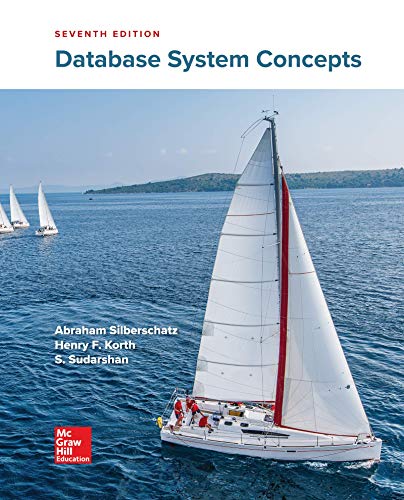
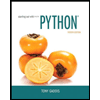
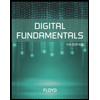
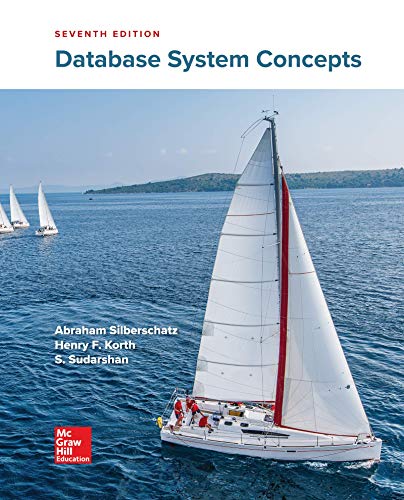
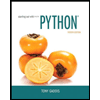
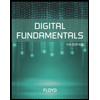
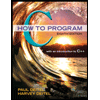
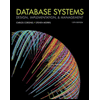
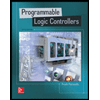