JAVA PROGRAM Chapter 5. PC #2. Retail Price Calculator (page 312) Write a program that asks the user to enter an item’s wholesale cost and its markup percentage. It should then display the item’s retail price. For example: • If an item’s wholesale cost is 5.00 and its markup percentage is 100 percent, then the item’s retail price is 10.00. • If an item’s wholesale cost is 5.00 and its markup percentage is 50 percent, then the item’s retail price is 7.50. The program should have a method named calculateRetail that receives the wholesale cost and the markup percentage as arguments, and returns the retail price of the item. Class name: RetailPriceCalculator PLEASE MODIFY THIS JAVA PROGRAM SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES ALL TEST CASSES PLEASE. RIGHT NOW IT SAYS 0 OUT 5 PASSED. THE PROGRAM IS NOT WORKING IN HYPERGRADE FIX IT SO IT WILL WORK IN HYPERGRADE. THANK YOU. import java.util.Scanner; public class Main { public static double calculateRetail(double wholesale, double percentage) { if (percentage < -100) { throw new IllegalArgumentException("Markup cannot be less than -100%."); } return wholesale + wholesale * (percentage / 100); } public static void main(String[] args) { Scanner sc = new Scanner(System.in); double wholesale; double percentage; do { System.out.println("Please enter the wholesale cost: "); wholesale = sc.nextDouble(); } while (wholesale < 0); do { System.out.println("Please enter the markup percentage: "); percentage = sc.nextDouble(); } while (percentage < -100); double retailPrice = calculateRetail(wholesale, percentage); System.out.printf("The retail price is: %.2f\n", retailPrice); } } Test Case 1 Please enter the wholesale cost or -1 exit:\n 10ENTER Please enter the markup percentage or -1 exit:\n -1ENTER Test Case 2 Please enter the wholesale cost or -1 exit:\n 100ENTER Please enter the markup percentage or -1 exit:\n 100ENTER The retail price is: 200.00\n Please enter the wholesale cost or -1 exit:\n -1ENTER Test Case 3 Please enter the wholesale cost or -1 exit:\n 10ENTER Please enter the markup percentage or -1 exit:\n 50ENTER The retail price is: 15.00\n Please enter the wholesale cost or -1 exit:\n 10ENTER Please enter the markup percentage or -1 exit:\n 100ENTER The retail price is: 20.00\n Please enter the wholesale cost or -1 exit:\n 10ENTER Please enter the markup percentage or -1 exit:\n -100ENTER The retail price is: 0.00\n Please enter the wholesale cost or -1 exit:\n -1ENTER Test Case 4 Please enter the wholesale cost or -1 exit:\n -200ENTER Wholesale cost cannot be a negative value.\n Please enter the wholesale cost again or -1 exit:\n 10ENTER Please enter the markup percentage or -1 exit:\n -200ENTER Markup cannot be less than -100%.\n Please enter the markup again or -1 exit:\n 50ENTER The retail price is: 15.00\n Please enter the wholesale cost or -1 exit:\n -1ENTER Test Case 5 Please enter the wholesale cost or -1 exit:\n -1ENTER
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
JAVA
PLEASE MODIFY THIS JAVA PROGRAM SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES ALL TEST CASSES PLEASE. RIGHT NOW IT SAYS 0 OUT 5 PASSED. THE PROGRAM IS NOT WORKING IN HYPERGRADE FIX IT SO IT WILL WORK IN HYPERGRADE. THANK YOU.
import java.util.Scanner;
public class Main {
public static double calculateRetail(double wholesale, double percentage) {
if (percentage < -100) {
throw new IllegalArgumentException("Markup cannot be less than -100%.");
}
return wholesale + wholesale * (percentage / 100);
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double wholesale;
double percentage;
do {
System.out.println("Please enter the wholesale cost: ");
wholesale = sc.nextDouble();
} while (wholesale < 0);
do {
System.out.println("Please enter the markup percentage: ");
percentage = sc.nextDouble();
} while (percentage < -100);
double retailPrice = calculateRetail(wholesale, percentage);
System.out.printf("The retail price is: %.2f\n", retailPrice);
}
}
Test Case 1
10ENTER
Please enter the markup percentage or -1 exit:\n
-1ENTER
Test Case 2
100ENTER
Please enter the markup percentage or -1 exit:\n
100ENTER
The retail price is: 200.00\n
Please enter the wholesale cost or -1 exit:\n
-1ENTER
Test Case 3
10ENTER
Please enter the markup percentage or -1 exit:\n
50ENTER
The retail price is: 15.00\n
Please enter the wholesale cost or -1 exit:\n
10ENTER
Please enter the markup percentage or -1 exit:\n
100ENTER
The retail price is: 20.00\n
Please enter the wholesale cost or -1 exit:\n
10ENTER
Please enter the markup percentage or -1 exit:\n
-100ENTER
The retail price is: 0.00\n
Please enter the wholesale cost or -1 exit:\n
-1ENTER
Test Case 4
-200ENTER
Wholesale cost cannot be a negative value.\n
Please enter the wholesale cost again or -1 exit:\n
10ENTER
Please enter the markup percentage or -1 exit:\n
-200ENTER
Markup cannot be less than -100%.\n
Please enter the markup again or -1 exit:\n
50ENTER
The retail price is: 15.00\n
Please enter the wholesale cost or -1 exit:\n
-1ENTER
Test Case 5
-1ENTER

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

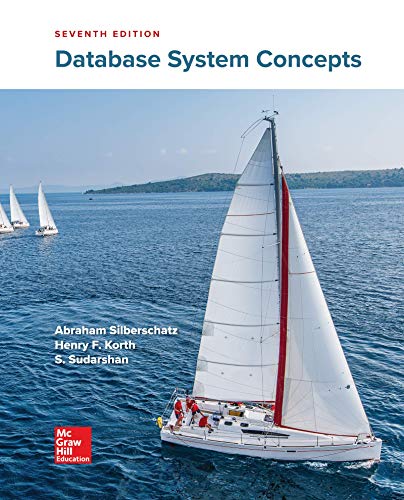
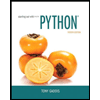
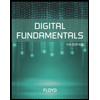
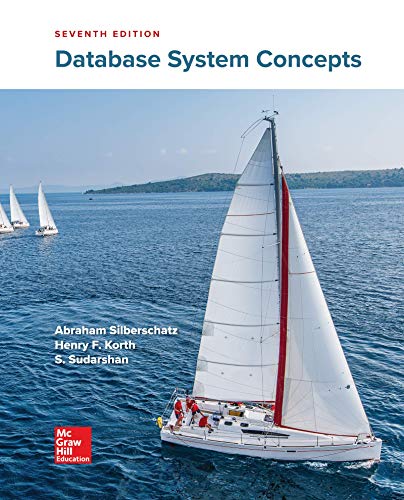
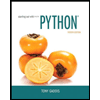
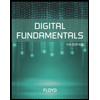
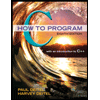
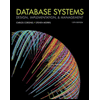
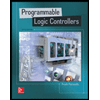