Chapter 5. PC #2. Retail Price Calculator (page 312) Write a program that asks the user to enter an item’s wholesale cost and its markup percentage. It should then display the item’s retail price. For example: • If an item’s wholesale cost is 5.00 and its markup percentage is 100 percent, then the item’s retail price is 10.00. • If an item’s wholesale cost is 5.00 and its markup percentage is 50 percent, then the item’s retail price is 7.50. The program should have a method named calculateRetail that receives the wholesale cost and the markup percentage as arguments, and returns the retail price of the item. Class name: RetailPriceCalculator Here is a working code but please fix it so it will in Hypergrade which as all the test casses. I DO NOT NEED THANK YOU IN THE PROGRAM. IT HAS TO PASS ALL THE TEST CASSES PLEASE. THANK YOU!!!!!: import java.util.Scanner; public class RetailPriceCalculator { public static double calculateRetail(double wholesale, double percentage) { double retailPrice = wholesale + wholesale * (percentage / 100); return retailPrice; } public static void main(String[] args) { Scanner sc = new Scanner(System.in); double wholesale = 0; double percentage = 0; // To check if it is the wholesale or percentage input error // n checks if it is a first-time input error or multiple time error int n = 0; boolean wholeSale = true; boolean percent = true; boolean condition = true; while (condition) { while (wholeSale) { if (n == 0) { System.out.println("Please enter the wholesale cost or -1 to exit: "); } else { System.out.println("Please enter the wholesale cost again or -1 to exit: "); n = 0; } wholesale = sc.nextDouble(); if (wholesale == -1) { condition = false; // Exit the loop break; } else if (wholesale < 0) { System.out.println("Wholesale cost cannot be a negative value."); n = 1; // Set the flag to indicate a second input error continue; // Continue to prompt for input } wholeSale = false; // Valid input received, exit the inner loop } if (condition == false) { break; // Exit the outer loop } while (percent) { if (n == 0) { System.out.println("Please enter the markup percentage or -1 to exit: "); } else { System.out.println("Please enter the markup again or -1 to exit: "); n = 0; } percentage = sc.nextDouble(); if (percentage == -1) { condition = false; // Exit the loop break; } else if (percentage < -100) { System.out.println("Markup cannot be less than -100%."); n = 1; // Set the flag to indicate a second input error continue; // Continue to prompt for input } percent = false; // Valid input received, exit the inner loop } if (condition == false) { break; // Exit the outer loop } wholeSale = true; // Reset the flag for the next input percent = true; // Reset the flag for the next input double retailPrice = calculateRetail(wholesale, percentage); System.out.printf("The retail price is: %.2f\n", retailPrice); } } } Test Case 1 Please enter the wholesale cost or -1 exit:\n 10ENTER Please enter the markup percentage or -1 exit:\n -1ENTER Test Case 2 Please enter the wholesale cost or -1 exit:\n 100ENTER Please enter the markup percentage or -1 exit:\n 100ENTER The retail price is: 200.00\n Please enter the wholesale cost or -1 exit:\n -1ENTER Test Case 3 Please enter the wholesale cost or -1 exit:\n 10ENTER Please enter the markup percentage or -1 exit:\n 50ENTER The retail price is: 15.00\n Please enter the wholesale cost or -1 exit:\n 10ENTER Please enter the markup percentage or -1 exit:\n 100ENTER The retail price is: 20.00\n Please enter the wholesale cost or -1 exit:\n 10ENTER Please enter the markup percentage or -1 exit:\n -100ENTER The retail price is: 0.00\n Please enter the wholesale cost or -1 exit:\n -1ENTER Test Case 4 Please enter the wholesale cost or -1 exit:\n -200ENTER Wholesale cost cannot be a negative value.\n Please enter the wholesale cost again or -1 exit:\n 10ENTER Please enter the markup percentage or -1 exit:\n -200ENTER Markup cannot be less than -100%.\n Please enter the markup again or -1 exit:\n 50ENTER The retail price is: 15.00\n Please enter the wholesale cost or -1 exit:\n -1ENTER Test Case 5 Please enter the wholesale cost or -1 exit:\n -1ENTER
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Here is a working code but please fix it so it will in Hypergrade which as all the test casses. I DO NOT NEED THANK YOU IN THE PROGRAM. IT HAS TO PASS ALL THE TEST CASSES PLEASE. THANK YOU!!!!!:
import java.util.Scanner;
public class RetailPriceCalculator {
public static double calculateRetail(double wholesale, double percentage) {
double retailPrice = wholesale + wholesale * (percentage / 100);
return retailPrice;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double wholesale = 0;
double percentage = 0;
// To check if it is the wholesale or percentage input error
// n checks if it is a first-time input error or multiple time error
int n = 0;
boolean wholeSale = true;
boolean percent = true;
boolean condition = true;
while (condition) {
while (wholeSale) {
if (n == 0) {
System.out.println("Please enter the wholesale cost or -1 to exit: ");
} else {
System.out.println("Please enter the wholesale cost again or -1 to exit: ");
n = 0;
}
wholesale = sc.nextDouble();
if (wholesale == -1) {
condition = false; // Exit the loop
break;
} else if (wholesale < 0) {
System.out.println("Wholesale cost cannot be a negative value.");
n = 1; // Set the flag to indicate a second input error
continue; // Continue to prompt for input
}
wholeSale = false; // Valid input received, exit the inner loop
}
if (condition == false) {
break; // Exit the outer loop
}
while (percent) {
if (n == 0) {
System.out.println("Please enter the markup percentage or -1 to exit: ");
} else {
System.out.println("Please enter the markup again or -1 to exit: ");
n = 0;
}
percentage = sc.nextDouble();
if (percentage == -1) {
condition = false; // Exit the loop
break;
} else if (percentage < -100) {
System.out.println("Markup cannot be less than -100%.");
n = 1; // Set the flag to indicate a second input error
continue; // Continue to prompt for input
}
percent = false; // Valid input received, exit the inner loop
}
if (condition == false) {
break; // Exit the outer loop
}
wholeSale = true; // Reset the flag for the next input
percent = true; // Reset the flag for the next input
double retailPrice = calculateRetail(wholesale, percentage);
System.out.printf("The retail price is: %.2f\n", retailPrice);
}
}
}
Test Case 1
10ENTER
Please enter the markup percentage or -1 exit:\n
-1ENTER
Test Case 2
100ENTER
Please enter the markup percentage or -1 exit:\n
100ENTER
The retail price is: 200.00\n
Please enter the wholesale cost or -1 exit:\n
-1ENTER
Test Case 3
10ENTER
Please enter the markup percentage or -1 exit:\n
50ENTER
The retail price is: 15.00\n
Please enter the wholesale cost or -1 exit:\n
10ENTER
Please enter the markup percentage or -1 exit:\n
100ENTER
The retail price is: 20.00\n
Please enter the wholesale cost or -1 exit:\n
10ENTER
Please enter the markup percentage or -1 exit:\n
-100ENTER
The retail price is: 0.00\n
Please enter the wholesale cost or -1 exit:\n
-1ENTER
Test Case 4
-200ENTER
Wholesale cost cannot be a negative value.\n
Please enter the wholesale cost again or -1 exit:\n
10ENTER
Please enter the markup percentage or -1 exit:\n
-200ENTER
Markup cannot be less than -100%.\n
Please enter the markup again or -1 exit:\n
50ENTER
The retail price is: 15.00\n
Please enter the wholesale cost or -1 exit:\n
-1ENTER
Test Case 5
-1ENTER

Below is the java code for the class RetailPriceCalculator:
1import java.util.Scanner;
2
3public class RetailPriceCalculator {
4 public static double calculateRetail(double wholesale, double percentage) {
5 double retailPrice = wholesale + wholesale * (percentage / 100);
6 return retailPrice;
7 }
8
9 public static void main(String[] args) {
10 Scanner sc = new Scanner(System.in);
11 double wholesale = 0;
12 double percentage = 0;
13 // To check if it is the first or second input error
14 int n = 0;
15 boolean wholeSale = true;
16 boolean percent = true;
17 boolean condition = true;
18
19 while (condition) {
20 while (wholeSale) {
21 if (n == 0) {
22 System.out.println("Please enter the wholesale cost or -1 to exit: ");
23 } else {
24 System.out.println("Please enter the wholesale cost again or -1 to exit: ");
25 n = 0;
26 }
27 wholesale = sc.nextDouble();
28
29 if (wholesale == -1) {
30 condition = false; // Exit the loop
31 break;
32 } else if (wholesale < 0) {
33 System.out.println("Wholesale cost cannot be a negative value.");
34 n = 1; // Set the flag to indicate a second input error
35 continue; // Continue to prompt for input
36 }
37 wholeSale = false; // Valid input received, exit the inner loop
38 }
39 if (condition == false) {
40 break; // Exit the outer loop
41 }
42
43 while (percent) {
44 if (n == 0) {
45 System.out.println("Please enter the markup percentage or -1 to exit: ");
46 } else {
47 System.out.println("Please enter the markup again or -1 to exit: ");
48 n = 0;
49 }
50
51 percentage = sc.nextDouble();
52
53 if (percentage == -1) {
54 condition = false; // Exit the loop
55 break;
56 } else if (percentage < -100) {
57 System.out.println("Markup cannot be less than -100%.");
58 n = 1; // Set the flag to indicate a second input error
59 continue; // Continue to prompt for input
60 }
61 percent = false; // Valid input received, exit the inner loop
62 }
63 if (condition == false) {
64 break; // Exit the outer loop
65 }
66 wholeSale = true; // Reset the flag for the next input
67 percent = true; // Reset the flag for the next input
68 double retailPrice = calculateRetail(wholesale, percentage);
69 System.out.printf("The retail price is: %.2f\n", retailPrice);
70 }
71 }
72}
73
Step by step
Solved in 3 steps with 5 images

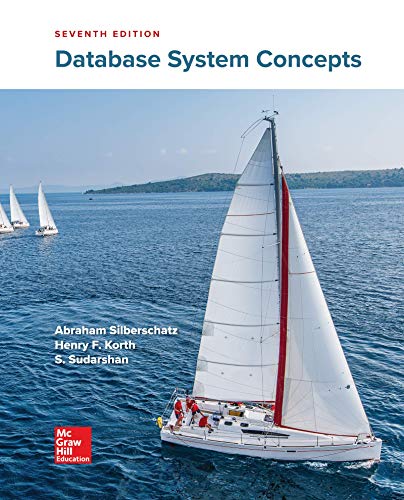
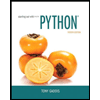
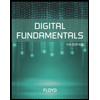
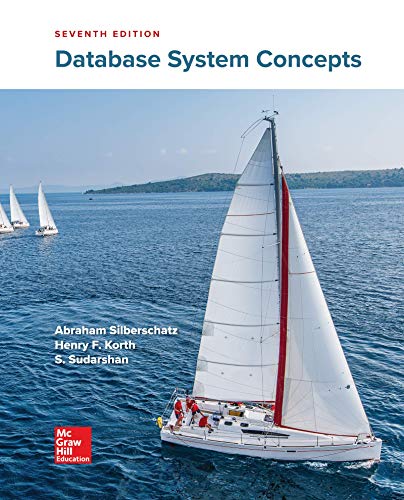
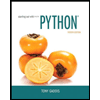
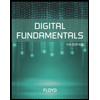
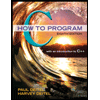
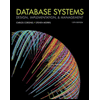
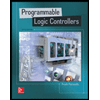