#include #include #include using namespace std; struct Player { string firstName; string lastName; int jerseyNumber; int rating; }; // Prompt menu options to user void displayMenu() { cout << "MENU\n"; cout << "a - Add player\n"; cout << "d - Remove player\n"; cout << "u - Update player rating\n"; cout << "o - Output roster\n"; cout << "q - Quit\n"; cout << "\nChoose an option: \n"; } // Add new player to the roster void addPlayer(vector& roster) { Player newPlayer; cout << "\nEnter the new player's data\n"; cout << "first name:\n"; cin >> newPlayer.firstName; cout << "last name:\n"; cin >> newPlayer.lastName; cout << "jersey number:\n"; cin >> newPlayer.jerseyNumber; cout << "rating:\n\n"; cin >> newPlayer.rating; roster.push_back(newPlayer); } // Remove a player from the roster based on their jersey number void removePlayer(vector& roster) { int jerseyNumber; cout << "\nEnter a jersey number: "; cin >> jerseyNumber; auto iter = find_if(roster.begin(), roster.end(), [jerseyNumber](const Player& p) { return p.jerseyNumber == jerseyNumber; }); if (iter == roster.end()) { cout << "Player not found.\n"; } else { roster.erase(iter); cout << "Player removed from roster.\n"; } } // Update a player's rating based on their jersey number void updatePlayerRating(vector& roster) { int jerseyNumber; cout << "\nEnter a jersey number: "<> jerseyNumber; auto iter = find_if(roster.begin(), roster.end(), [jerseyNumber](const Player& p) { return p.jerseyNumber == jerseyNumber; }); if (iter == roster.end()) { cout << "Player not found.\n"; } else { int newRating; cout << "Enter a new rating for player: "; cin >> newRating; iter->rating = newRating; cout << "Player rating updated.\n"; } } // Output the roster void outputRoster(const vector& roster) { cout << "\nROSTER\n"; for (size_t i = 0; i < roster.size(); i++) { cout << "Player " << i + 1 << " -- " << roster[i].firstName << " " << roster[i].lastName << ", jersey number " << roster[i].jerseyNumber << ", rating " << roster[i].rating << endl; } cout << endl; } int main() { vector roster; char option; do { displayMenu(); cin >> option; switch (option) { case 'a': addPlayer(roster); break; case 'd': removePlayer(roster); break; case 'u': updatePlayerRating(roster); break; case 'o': outputRoster(roster); break; case 'q': break; default: cout << "Invalid option. Please try again.\n"; } } while (option != 'q'); return 0; } SO I need to put a blank space under the second Choose an option(the last line)
#include <iostream>
#include <
#include <algorithm>
using namespace std;
struct Player {
string firstName;
string lastName;
int jerseyNumber;
int rating;
};
// Prompt menu options to user
void displayMenu() {
cout << "MENU\n";
cout << "a - Add player\n";
cout << "d - Remove player\n";
cout << "u - Update player rating\n";
cout << "o - Output roster\n";
cout << "q - Quit\n";
cout << "\nChoose an option: \n";
}
// Add new player to the roster
void addPlayer(vector<Player>& roster) {
Player newPlayer;
cout << "\nEnter the new player's data\n";
cout << "first name:\n";
cin >> newPlayer.firstName;
cout << "last name:\n";
cin >> newPlayer.lastName;
cout << "jersey number:\n";
cin >> newPlayer.jerseyNumber;
cout << "rating:\n\n";
cin >> newPlayer.rating;
roster.push_back(newPlayer);
}
// Remove a player from the roster based on their jersey number
void removePlayer(vector<Player>& roster) {
int jerseyNumber;
cout << "\nEnter a jersey number: ";
cin >> jerseyNumber;
auto iter = find_if(roster.begin(), roster.end(), [jerseyNumber](const Player& p) { return p.jerseyNumber == jerseyNumber; });
if (iter == roster.end()) {
cout << "Player not found.\n";
}
else {
roster.erase(iter);
cout << "Player removed from roster.\n";
}
}
// Update a player's rating based on their jersey number
void updatePlayerRating(vector<Player>& roster) {
int jerseyNumber;
cout << "\nEnter a jersey number: "<<endl;
cin >> jerseyNumber;
auto iter = find_if(roster.begin(), roster.end(), [jerseyNumber](const Player& p) { return p.jerseyNumber == jerseyNumber; });
if (iter == roster.end()) {
cout << "Player not found.\n";
}
else {
int newRating;
cout << "Enter a new rating for player: ";
cin >> newRating;
iter->rating = newRating;
cout << "Player rating updated.\n";
}
}
// Output the roster
void outputRoster(const vector<Player>& roster) {
cout << "\nROSTER\n";
for (size_t i = 0; i < roster.size(); i++) {
cout << "Player " << i + 1 << " -- " << roster[i].firstName << " " << roster[i].lastName << ", jersey number " << roster[i].jerseyNumber << ", rating " << roster[i].rating << endl;
}
cout << endl;
}
int main() {
vector<Player> roster;
char option;
do {
displayMenu();
cin >> option;
switch (option) {
case 'a':
addPlayer(roster);
break;
case 'd':
removePlayer(roster);
break;
case 'u':
updatePlayerRating(roster);
break;
case 'o':
outputRoster(roster);
break;
case 'q':
break;
default:
cout << "Invalid option. Please try again.\n";
}
} while (option != 'q');
return 0;
}
SO I need to put a blank space under the second Choose an option(the last line)



Step by step
Solved in 4 steps with 5 images

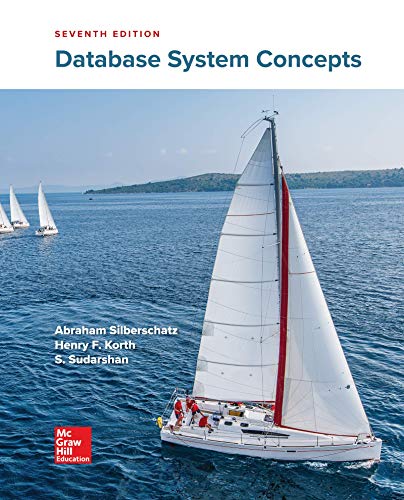
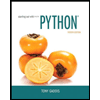
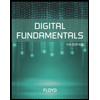
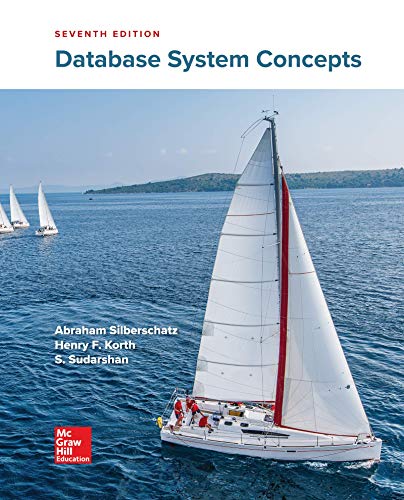
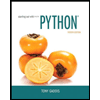
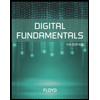
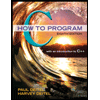
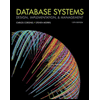
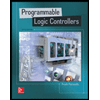