#include <ctime>#include <cstdlib>#include<iostream> using namespace std; char choice;void printMenu();int getSelect(int&);bool isUnique(int[], int, int);void printArray(int[], int); int drawing[] = { 56,34,1,8,12 }; int main(){ int sel; printMenu(); getSelect(sel); return 0;} void printMenu(){ cout << " Welcome to the Lottery\t\n"; cout << "---------------------------------\n"; cout << " 1. Quick Pick\n"; cout << " 2. Pick Numbers\n"; cout << " 3. Exit\n";} void selection1_output(){ int r[5]; for (int i = 0; i < 5; i++) { r[i] = 1 + (rand() % 69); } cout << "Players Ticket : "; for (int i = 0; i < 5; i++) { cout << r[i] << " "; } cout << "\nDrawings : "; for (int i = 0; i < 5; i++) { cout << drawing[i] << " "; } int same = 0; for (int i = 0; i < 5; i++) { for (int j = 0; j < 5; j++) { if (r[j] == drawing[i]) same++; } } if (same == 5) cout << "\nYou Won the grand prize\n"; else cout << "\nBetter luck next time!\n"; } void selection2_output(int ticket[]){ cout << "Drawings : "; for (int i = 0; i < 5; i++) { cout << drawing[i] << " "; } int same = 0; for (int i = 0; i < 5; i++) { for (int j = 0; j < 5; j++) { if (ticket[j] == drawing[i]) same++; } } if (same == 5) cout << "\nYou Won the grand prize\n"; else cout << "\nBetter luck next time!\n"; } int getSelect(int& sel){ int count = 0; int ticket[5]; cout << " Selection: "; cin >> sel; while (true) { while (sel <= 0 || sel > 3) { cout << " Please your selction must be between 1 and 3. Try again.\n"; return 0; } while (sel == 1) { selection1_output(); break; } while (sel == 2) { while (count < 5) { cout << "Enter a unique number (1 - 69): "; cin >> ticket[count]; while (ticket[count] < 1 || ticket[count] > 69 || !isUnique(ticket, ticket[count], count)) { cout << "Try again.\n"; cout << "Enter a unique number (1 - 69): "; cin >> ticket[count]; break; } count++; cout << endl; } isUnique(ticket, ticket[count], count); cout << "Player Ticket: ", printArray(ticket, count); selection2_output(ticket); break; } while (sel == 3) { cout << "Program ending..." << endl; return 0; } return sel; }}bool isUnique(int ticket[], int n, int count){ for (int i = 0; i < count; i++) { if (ticket[i] == n) { return false; } } return true;}void printArray(int ticket[], int count){ for (int i = 0; i < count; i++) { cout << ticket[i] << " "; } cout << endl;} where do i insert a while loop to have the player keep going
#include <ctime>
#include <cstdlib>
#include<iostream>
using namespace std;
char choice;
void printMenu();
int getSelect(int&);
bool isUnique(int[], int, int);
void printArray(int[], int);
int drawing[] = { 56,34,1,8,12 };
int main()
{
int sel;
printMenu();
getSelect(sel);
return 0;
}
void printMenu()
{
cout << " Welcome to the Lottery\t\n";
cout << "---------------------------------\n";
cout << " 1. Quick Pick\n";
cout << " 2. Pick Numbers\n";
cout << " 3. Exit\n";
}
void selection1_output()
{
int r[5];
for (int i = 0; i < 5; i++)
{
r[i] = 1 + (rand() % 69);
}
cout << "Players Ticket : ";
for (int i = 0; i < 5; i++)
{
cout << r[i] << " ";
}
cout << "\nDrawings : ";
for (int i = 0; i < 5; i++)
{
cout << drawing[i] << " ";
}
int same = 0;
for (int i = 0; i < 5; i++)
{
for (int j = 0; j < 5; j++)
{
if (r[j] == drawing[i])
same++;
}
}
if (same == 5)
cout << "\nYou Won the grand prize\n";
else
cout << "\nBetter luck next time!\n";
}
void selection2_output(int ticket[])
{
cout << "Drawings : ";
for (int i = 0; i < 5; i++)
{
cout << drawing[i] << " ";
}
int same = 0;
for (int i = 0; i < 5; i++)
{
for (int j = 0; j < 5; j++)
{
if (ticket[j] == drawing[i])
same++;
}
}
if (same == 5)
cout << "\nYou Won the grand prize\n";
else
cout << "\nBetter luck next time!\n";
}
int getSelect(int& sel)
{
int count = 0;
int ticket[5];
cout << " Selection: ";
cin >> sel;
while (true)
{
while (sel <= 0 || sel > 3)
{
cout << " Please your selction must be between 1 and 3. Try again.\n";
return 0;
}
while (sel == 1)
{
selection1_output();
break;
}
while (sel == 2)
{
while (count < 5)
{
cout << "Enter a unique number (1 - 69): ";
cin >> ticket[count];
while (ticket[count] < 1 || ticket[count] > 69 || !isUnique(ticket, ticket[count], count))
{
cout << "Try again.\n";
cout << "Enter a unique number (1 - 69): ";
cin >> ticket[count];
break;
}
count++;
cout << endl;
}
isUnique(ticket, ticket[count], count);
cout << "Player Ticket: ", printArray(ticket, count);
selection2_output(ticket);
break;
}
while (sel == 3)
{
cout << "Program ending..." << endl;
return 0;
}
return sel;
}
}
bool isUnique(int ticket[], int n, int count)
{
for (int i = 0; i < count; i++)
{
if (ticket[i] == n)
{
return false;
}
}
return true;
}
void printArray(int ticket[], int count)
{
for (int i = 0; i < count; i++)
{
cout << ticket[i] << " ";
}
cout << endl;
}
where do i insert a while loop to have the player keep going

Step by step
Solved in 2 steps

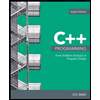
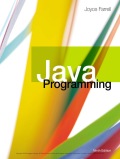
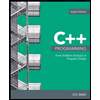
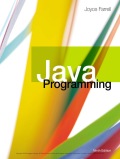
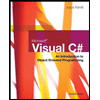
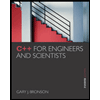
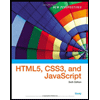