( I need the output to be: Enter the number of vertices and edges: Enter edges in the format (from to weight): Enter the source vertex: Shortest paths from source vertex 2: Vertex 0: 4 Vertex 1: 3 Vertex 2: 0 the output i keep getting from the code below is Enter the number of vertices and edges: Enter edges in the format (from to weight): Enter the source vertex: Shortest paths from source vertex 2: Vertex 0: No path Vertex 1: No path Vertex 2: 0) #include <iostream> #include <vector> #include <queue> #include <climits> using namespace std; vector<int> dijkstra(int V, vector<vector<pair<int, int>>> adj, int S, vector<int>& dist) { dist.resize(V, INT_MAX); // dist[i] will hold the shortest distance from S to i vector<int> prev(V, -1); // prev[i] will hold the penultimate vertex in the shortest path from S to i // Priority queue to store vertices that are being processed priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq; // Initialize source vertex distance as 0 and push it to the priority queue dist[S] = 0; // Adjusted: Set distance from source to itself as 0 pq.push({0, S}); // Dijkstra's algorithm while (!pq.empty()) { int u = pq.top().second; pq.pop(); // Visit each neighbor of u for (auto& edge : adj[u]) { int v = edge.first; // neighbor vertex int weight = edge.second; // weight of the edge from u to v // If a shorter path is found, update distance and penultimate vertex if (dist[u] + weight < dist[v]) { dist[v] = dist[u] + weight; prev[v] = u; pq.push({dist[v], v}); } } } return prev; // Return the penultimate vertex array } int main() { int v, e, source; cout << "Enter the number of vertices and edges: "; cin >> v >> e; // Initialize the graph with n vertices vector<vector<pair<int, int>>> adj(v); // Read edges and weights cout << "Enter edges in the format (from to weight):" << endl; for (int i = 0; i < e; ++i) { int from, to, weight; cin >> from >> to >> weight; adj[from].push_back({to, weight}); } cout << "Enter the source vertex: "; cin >> source; // Find shortest paths from source to all vertices vector<int> dist; vector<int> prev_vertices = dijkstra(v, adj, source, dist); // Print shortest paths cout << "Shortest paths from source vertex " << source << ":\n"; for (int i = 0; i < v; ++i) { cout << "Vertex " << i << ": "; if (dist[i] == INT_MAX) { cout << "No path\n"; } else { cout << dist[i] << endl; } } return 0; }
( I need the output to be: Enter the number of vertices and edges: Enter edges in the format (from to weight): Enter the source vertex: Shortest paths from source vertex 2: Vertex 0: 4 Vertex 1: 3 Vertex 2: 0 the output i keep getting from the code below is Enter the number of vertices and edges: Enter edges in the format (from to weight): Enter the source vertex: Shortest paths from source vertex 2: Vertex 0: No path Vertex 1: No path Vertex 2: 0) #include <iostream> #include <vector> #include <queue> #include <climits> using namespace std; vector<int> dijkstra(int V, vector<vector<pair<int, int>>> adj, int S, vector<int>& dist) { dist.resize(V, INT_MAX); // dist[i] will hold the shortest distance from S to i vector<int> prev(V, -1); // prev[i] will hold the penultimate vertex in the shortest path from S to i // Priority queue to store vertices that are being processed priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq; // Initialize source vertex distance as 0 and push it to the priority queue dist[S] = 0; // Adjusted: Set distance from source to itself as 0 pq.push({0, S}); // Dijkstra's algorithm while (!pq.empty()) { int u = pq.top().second; pq.pop(); // Visit each neighbor of u for (auto& edge : adj[u]) { int v = edge.first; // neighbor vertex int weight = edge.second; // weight of the edge from u to v // If a shorter path is found, update distance and penultimate vertex if (dist[u] + weight < dist[v]) { dist[v] = dist[u] + weight; prev[v] = u; pq.push({dist[v], v}); } } } return prev; // Return the penultimate vertex array } int main() { int v, e, source; cout << "Enter the number of vertices and edges: "; cin >> v >> e; // Initialize the graph with n vertices vector<vector<pair<int, int>>> adj(v); // Read edges and weights cout << "Enter edges in the format (from to weight):" << endl; for (int i = 0; i < e; ++i) { int from, to, weight; cin >> from >> to >> weight; adj[from].push_back({to, weight}); } cout << "Enter the source vertex: "; cin >> source; // Find shortest paths from source to all vertices vector<int> dist; vector<int> prev_vertices = dijkstra(v, adj, source, dist); // Print shortest paths cout << "Shortest paths from source vertex " << source << ":\n"; for (int i = 0; i < v; ++i) { cout << "Vertex " << i << ": "; if (dist[i] == INT_MAX) { cout << "No path\n"; } else { cout << dist[i] << endl; } } return 0; }
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section6.4: A Case Study: Rectangular To Polar Coordinate Conversion
Problem 9E: (Numerical) Write a program that tests the effectiveness of the rand() library function. Start by...
Related questions
Question
( I need the output to be: Enter the number of vertices and edges: Enter edges in the format (from to weight): Enter the source vertex: Shortest paths from source vertex 2: Vertex 0: 4 Vertex 1: 3 Vertex 2: 0 the output i keep getting from the code below is Enter the number of vertices and edges: Enter edges in the format (from to weight): Enter the source vertex: Shortest paths from source vertex 2: Vertex 0: No path Vertex 1: No path Vertex 2: 0)
#include <iostream>
#include <vector >
#include <queue>
#include <climits>
using namespace std;
vector<int> dijkstra(int V, vector<vector<pair<int, int>>> adj, int S, vector<int>& dist) {
dist.resize(V, INT_MAX); // dist[i] will hold the shortest distance from S to i
vector<int> prev(V, -1); // prev[i] will hold the penultimate vertex in the shortest path from S to i
// Priority queue to store vertices that are being processed
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq;
// Initialize source vertex distance as 0 and push it to the priority queue
dist[S] = 0; // Adjusted: Set distance from source to itself as 0
pq.push({0, S});
// Dijkstra's algorithm
while (!pq.empty()) {
int u = pq.top().second;
pq.pop();
// Visit each neighbor of u
for (auto& edge : adj[u]) {
int v = edge.first; // neighbor vertex
int weight = edge.second; // weight of the edge from u to v
// If a shorter path is found, update distance and penultimate vertex
if (dist[u] + weight < dist[v]) {
dist[v] = dist[u] + weight;
prev[v] = u;
pq.push({dist[v], v});
}
}
}
return prev; // Return the penultimate vertex array
}
int main() {
int v, e, source;
cout << "Enter the number of vertices and edges: ";
cin >> v >> e;
// Initialize the graph with n vertices
vector<vector<pair<int, int>>> adj(v);
// Read edges and weights
cout << "Enter edges in the format (from to weight):" << endl;
for (int i = 0; i < e; ++i) {
int from, to, weight;
cin >> from >> to >> weight;
adj[from].push_back({to, weight});
}
cout << "Enter the source vertex: ";
cin >> source;
// Find shortest paths from source to all vertices
vector<int> dist;
vector<int> prev_vertices = dijkstra(v, adj, source, dist);
// Print shortest paths
cout << "Shortest paths from source vertex " << source << ":\n";
for (int i = 0; i < v; ++i) {
cout << "Vertex " << i << ": ";
if (dist[i] == INT_MAX) {
cout << "No path\n";
} else {
cout << dist[i] << endl;
}
}
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
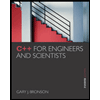
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
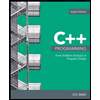
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
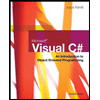
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
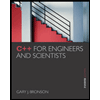
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
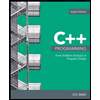
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
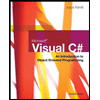
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
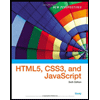
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L
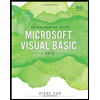
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning