In Python 3, write a program with classes to keep track of phones. Here are the requirements: Create a new class called Phone. The Phone class should have a class attribute for the owner's name. Since all phones have a manufacturer and a model number, your class should take the manufacturer and model number from the constructor and assign them to instance attributes. Your Phone constructor should be able to take the parameters. Override the print method for Phone to output a human-friendly version of the information. Your code should prompt the user for an owner name and then start prompting for the manufacturers and models for all phones for that user until the user enters a 'q' or 'Q'. After the entry print out a list of all the phones.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
In Python 3, write a program with classes to keep track of phones. Here are the requirements: Create a new class called Phone. The Phone class should have a class attribute for the owner's name. Since all phones have a manufacturer and a model number, your class should take the manufacturer and model number from the constructor and assign them to instance attributes. Your Phone constructor should be able to take the parameters. Override the print method for Phone to output a human-friendly version of the information. Your code should prompt the user for an owner name and then start prompting for the manufacturers and models for all phones for that user until the user enters a 'q' or 'Q'. After the entry print out a list of all the phones.

Steps:
- Create class Phone:
- Define __init__(self, *args)
- Define _str_(self)
- Test class Phone
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

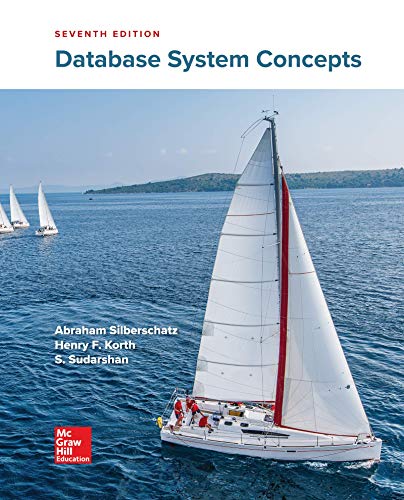
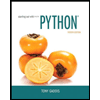
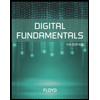
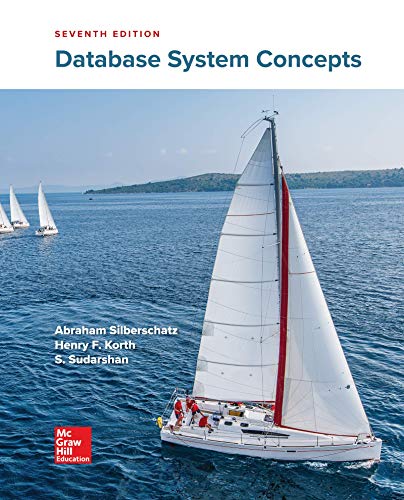
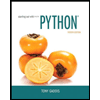
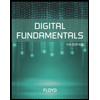
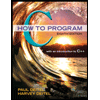
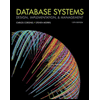
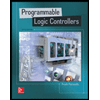