in java 1) create a class Cat... Create class Cat Add properties What characteristics do they have? Name, age, color, type (domestic / feral), etc. What color(s) might they have? White, cream, fawn, Cinnamon, Chocolate, Red, Lilac, Blue, Black, Lavender, etc. Add methods eat(), play(), etc. Constructors? A class provides a special type of methods, known as constructors, which are invoked to construct objects from the class Create 2 different constructor with different initialization parameters How to get the number of cats? Add a static variable and a static method Test Cat class by creating 2 Cat instances 2) create an Abstract class AbstractCat with the same properties and methods as Cat... Create class AbstractCat. How to secure data? To protect it from direct access and manipulation from outside of the class we need to make our instance data fields private. To let clients still manipulate data under our control we can provide them public getters and setters. create getters, setters, constructors and toString() Test AbstractCat class
Create class Cat
Add properties
What characteristics do they have?
Name, age, color, type (domestic / feral), etc.
What color(s) might they have?
White, cream, fawn, Cinnamon, Chocolate, Red, Lilac, Blue, Black,
Lavender, etc.
Add methods
eat(), play(), etc.
Constructors?
A class provides a special type of methods, known as constructors, which are
invoked to construct objects from the class
Create 2 different constructor with different initialization parameters
How to get the number of cats?
Add a static variable and a static method
Test Cat class by creating 2 Cat instances
properties and methods as Cat...
Create class AbstractCat.
How to secure data?
To protect it from direct access and manipulation from outside of the class
we need to make our instance data fields private.
To let clients still manipulate data under our control we can provide them
public getters and setters.
create getters, setters, constructors and toString()
Test AbstractCat class

Algorithm for Cat class:
----------------------------------------
1. Define a class named Cat.
2. Declare private instance variables: name, age, color, and type.
3. Declare a private static variable numberOfCats to keep track of the number of Cat objects.
4. Create constructors:
a. Constructor with name, age, color, and type as parameters.
- Initialize instance variables with the provided values.
- Increment numberOfCats by 1.
b. Constructor with name and age as parameters.
- Call the first constructor with default values for color and type.
5. Define methods:
a. eat():
- Display a message indicating that the cat is eating.
b. play():
- Display a message indicating that the cat is playing.
c. Static method getNumberOfCats():
- Return the value of numberOfCats.
d. Getters and setters for instance variables.
e. Override the toString() method to represent Cat objects as strings.
6. In the main method:
a. Create two Cat objects with different constructors.
b. Display the Cat objects.
c. Call the eat() and play() methods on Cat objects.
d. Display the number of cats using getNumberOfCats().
Algorithm for AbstractCat class:
----------------------------------------
1. Define an abstract class named AbstractCat.
2. Declare private instance variables: name, age, color, and type.
3. Create constructors:
a. Constructor with name, age, color, and type as parameters.
- Initialize instance variables with the provided values.
b. Constructor with name and age as parameters.
- Call the first constructor with default values for color and type.
4. Declare abstract methods:
a. eat(): To be implemented by subclasses.
b. play(): To be implemented by subclasses.
5. Define methods:
a. Getters and setters for instance variables.
b. Override the toString() method to represent AbstractCat objects as strings.
6. In the main method:
- Since AbstractCat is an abstract class, no instances of it are created directly in the main method.
- Subclasses of AbstractCat should be created to provide implementations for eat() and play() methods.
Step by step
Solved in 4 steps with 5 images

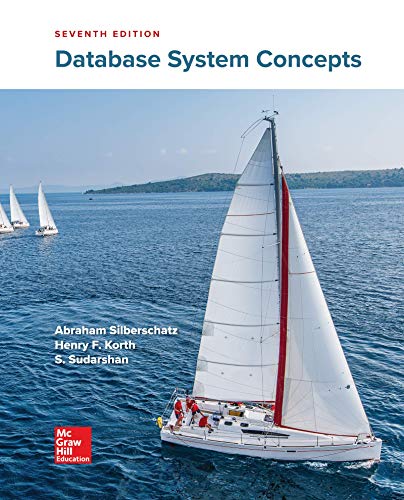
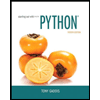
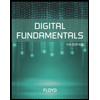
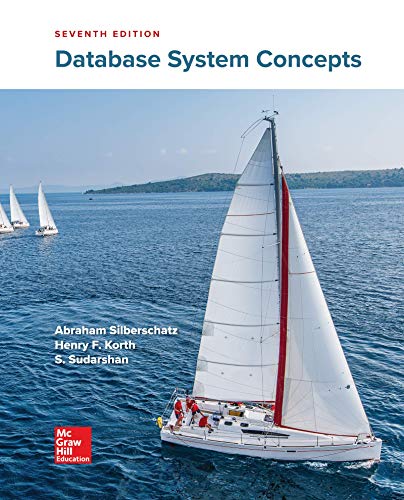
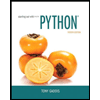
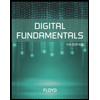
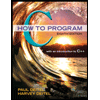
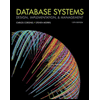
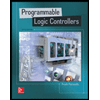