Investigate! Directions: Type each of the following statements into your main method. Test them one-by-one, commenting each one out when you're done with it. For each one, (i) write the output or write "compiler error"; (i) explain why you got the output you did, or why you got an error. System.out.println (mary.getSalary()): System.out.println (jill.getLanguage ()) ; System.out.println (mary.tostring ()): System.out.println (bob.tostring ()); Worker tl - bob; Worker t2 - mary: System.out.printin (t1.getSalary (); System.out.println (t2.getLanguage (); Programmer jane - new Programmer ("Jane", "Python", 47000) ; Worker temp - jane; temp.raisesalary (30000); System.out.println (temp.getsalary ()):



public class Worker {
private String name;
private double salary;
public Worker(String aName, double sal) {
name = aName;
salary = sal;
}
public String getName() {
return name;
}
public double getSalary() {
return salary;
}
public void work() {
System.out.println("Employee working");
}
public void raiseSalary(double byAmount) {
salary += byAmount;
}
public String toString() {
return name + " has a yearly salary: " + salary;
}
}
public class Programmer extends Worker {
private String programmingLanguage;
public Programmer(String aName, String lang, double sal) {
super(aName, sal);
programmingLanguage = lang;
}
public String getLanguage() {
return programmingLanguage;
}
public void work() {
System.out.println("Programmer working");
}
public String toString() {
return "Programmer: " + super.toString() + " and programs in " + programmingLanguage;
}
}
public class Manager extends Worker {
public Manager(String aName, double sal) {
super(aName, sal);
}
public void work() {
System.out.println("Manager working");
}
public String toString() {
return "Manager: " + super.toString();
}
}
public class Main {
public static void main(String[] args) {
Worker bob = new Worker("Bob", 15000);
Programmer mary = new Programmer("Mary", "Java", 70000);
Manager jill = new Manager("Jill", 56000);
/*
* Note : before compiling this we need to comment jill.getLanguage() and
* t2.getLanguage() as there is a compiler time error and the reason is stated
* below
*
* Output: 70000.0 this is because Mary is an object of class Programmer which
* is a subclass of Worker Worker class has a method getSalary which Programmer
* class can use because of inheritance value 70000 was set in the field salary
* at the time of object creation through constructor using super we passed
* 70000 but the output is 70000.0 because the data type is double.
*/
System.out.println(mary.getSalary()); // returns 700000
/*
* Output: compiler error
*
* There is a compile time error because jill is an object of class Manager
* which does not have the method getLanguage. It is present inside class
* Programmer.
*/
// System.out.println(jill.getLanguage());
/*
* Output: Programmer: Mary has a yearly salary: 70000.0 and programs in Java
*
* First toString() method of Programmer class is called which created a string
* starting with 'Programmer: ' and ending with ' and programs in Java' it calls
* the toString() method of its super class Worker which adds the string 'Mary
* has a yearly salary: 70000.0' in between the earlier two strings.
*/
System.out.println(mary.toString());
/*
* Output: Bob has a yearly salary: 15000.0
*
* toString() method of Worker class is called which created a string 'Bob has a
* yearly salary: 15000.0'.
*/
System.out.println(bob.toString());
Worker t1 = bob;
Worker t2 = mary;
/*
* Output: 15000.0
*
* t1 and bob are pointing to the same address therefore they point to the same
* object which has the name 'Bob' and salary '15000'
*/
System.out.println(t1.getSalary());
/*
* Output: compiler error
*
* There is a compile time error because although t2 is same as mary which is a
* programmer t2 is a variable of class Worker and Worker class does not have a
* method getLanguage(). to make the below line work we can write
* System.out.println(((Programmer) t2).getLanguage());
*/
// System.out.println((t2.getLanguage());
Programmer jane = new Programmer("Jane", "Python", 47000);
Worker temp = jane;
temp.raiseSalary(30000);
/*
* Output: 77000.0
*
* an Object of class Programmer is created which has salary 47000
* temp points to this same object
* using method raiseSalary() from class Programmer salary increased from 47000 to 77000
* temp using getSalary() method from the parent class Worker prints the salary.
*/
System.out.println(temp.getSalary());
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

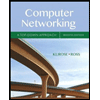
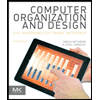
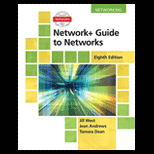
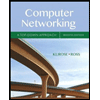
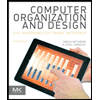
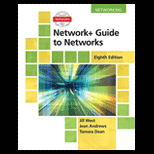
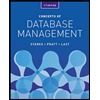
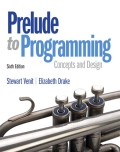
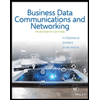