In Checkpoint B, the game will be playable as a two-player game, albeit the competition will be a computer player who makes moves randomly. Implement these functions from the template following the description (specification) in their docstring: shuffle() replace_deck() deal_initial_hands() play_hand() should be extended to support 'random' mode The main function to drive the game has been provided. In 'interactive' mode, play_hand() should behave the same way as in Part A In 'random' mode, the computer selects actions randomly. Compared to 'interactive' mode: Every call to input() for the player should be replaced with a random choice Extra printed feedback for the player should be omitted For testing, we have hard-coded the seed 26 for the random source Hint: you can use random.randint(x, y) to select a number between x and y, inclusive. In particular, "flipping a coin" would be accomplished via random.randint(0, 1) Sample input/output When the inputs are: yes 1 no yes 9 The expected behavior is (inputs are shown in-line) -------------------------------------------------- Deck: [3, 2] Discard pile: [7] Your current rack is: [9, 5, 1] Computer rack is: [4, 8, 6] The top discard card is 7 Do you want it? (yes or no): yes Enter the card in your rack to discard: 1 Your new rack is: [9, 5, 7] Computer rack is: [4, 8, 1] -------------------------------------------------- Deck: [3, 2] Discard pile: [6] Your current rack is: [9, 5, 7] Computer rack is: [4, 8, 1] The top discard card is 6 Do you want it? (yes or no): no The card from the deck is 2 Do you want it? (yes or no): yes Enter the number of the card you want to kick out: 9 Your new rack is: [2, 5, 7]
In Checkpoint B, the game will be playable as a two-player game, albeit the competition will be a computer player who makes moves randomly.
Implement these functions from the template following the description (specification) in their docstring:
- shuffle()
- replace_deck()
- deal_initial_hands()
- play_hand() should be extended to support 'random' mode
The main function to drive the game has been provided.
- In 'interactive' mode, play_hand() should behave the same way as in Part A
- In 'random' mode, the computer selects actions randomly. Compared to 'interactive' mode:
- Every call to input() for the player should be replaced with a random choice
- Extra printed feedback for the player should be omitted
- For testing, we have hard-coded the seed 26 for the random source
Hint: you can use random.randint(x, y) to select a number between x and y, inclusive. In particular, "flipping a coin" would be accomplished via random.randint(0, 1)
Sample input/output
When the inputs are:
yes
1
no
yes
9
The expected behavior is (inputs are shown in-line)
--------------------------------------------------
Deck: [3, 2]
Discard pile: [7]
Your current rack is: [9, 5, 1]
Computer rack is: [4, 8, 6]
The top discard card is 7
Do you want it? (yes or no):
yes Enter the card in your rack to discard:
1 Your new rack is: [9, 5, 7]
Computer rack is: [4, 8, 1]
--------------------------------------------------
Deck: [3, 2]
Discard pile: [6]
Your current rack is: [9, 5, 7]
Computer rack is: [4, 8, 1]
The top discard card is 6
Do you want it? (yes or no): no
The card from the deck is 2
Do you want it? (yes or no): yes
Enter the number of the card you want to kick out: 9
Your new rack is: [2, 5, 7]
HUMAN WINS! with hand of [2, 5, 7]
![1 def shuffle (card_pile):
2
3
4
5
6
7
8
9
15
16
10
11
12 def replace_deck(discard):
13
14
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
17
18
19
20
21
22
23
24 def deal initial_hands (deck, rack_size):
25
"""Deals inital hands to two players.
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
26 Cards are dealt one at a time, alternating.Player 2 gets the first card.
27
28
29
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
80
01
02
93
04
"""Shuffles the pile of cards.
05
Parameters:
card_pile (list): draw deck or discard pile
06
37
Returns: None
98
39
pass
10
11
"""Shuffle the discard pile and use it as the new draw deck.
Parameters:
discard (list): the current discard pile
Returns:
two lists: the new draw deck and the new discard pile
pass
55
56
57
58
59
60 if __name__ == '___main___':
61
62
63
64
65
66
67
68
Parameters:
deck (list): the draw deck
rack_size (int): the number cards to deal to each player
Returns:
two lists: the racks for Player 1 and Player 2.
pass
def play_hand(rack, deck, discard, mode='interactive'):
"""Plays a single hand of Racko. See input/output in documentation.
Parameters:
rack (list): the player's hand
deck (list): the deck used to draw new cards
discard (list): the deck used for the discard pile
Returns: None
if mode
'interactive':
# COPY FROM PART A
pass
elif mode == 'random':
# adapt the interactive player,
# but make all choices randomly and remove print statements
pass
"""Plays a two-player game of Racko with a human and computer player'
random.seed (26)
num_cards = 9 # 9, 12, 60
rack_size = 3 # 3, 4, 10
# A draw deck
deck = list (range (1, num_cards+1))
shuffle(deck)
#deal initial hands
human_hand, computer_hand = deal_initial_hands (deck, rack_size)
# An empty discard pile
discard = []
# To start, take the top of the deck and put it on the discard
discard_card = get_top_card (deck)
add_card_to_discard (discard_card, discard)
while not check_racko(human_hand) and not check_racko(computer_hand)
print('-'*50)
print('Deck:', deck)
print('Discard pile:', discard)
print('Your current rack is:', human_hand)
print('Computer rack is:', computer_hand)
play_hand (human_hand, deck, discard, mode='interactive')
print('Your new rack is:', human_hand)
if len(deck) == 0:
print("\nHuman: Deck is empty. Shuffling discard pile.")
deck, discard = replace_deck (discard)
elif check_racko(human_hand):
break
play_hand (computer_hand, deck, discard, mode="random')
print('Computer rack is:', computer_hand)
if len(deck) == 0:
print("\nComputer: Deck is empty. Shuffling discard pile."]
deck, discard replace_deck (discard)
else:
if check_racko (human_hand):
print("HUMAN WINS! with hand of", human_hand)
print("COMPUTER WINS! with hand of", computer_hand)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4d8acdf6-9c5e-42f8-ad34-e57535beff59%2Fefac9a2b-46e5-4d03-ba2a-398c32f12d0b%2F8unsp3m_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

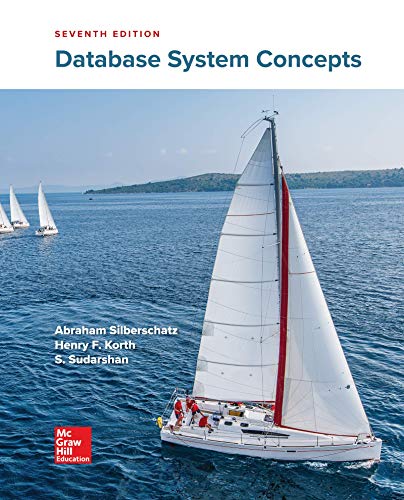
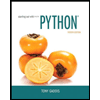
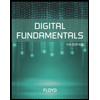
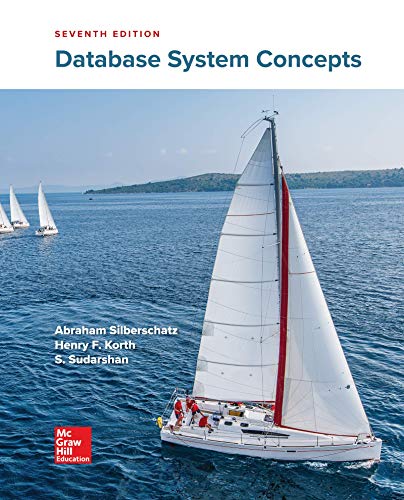
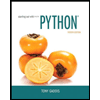
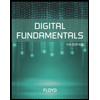
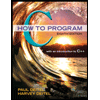
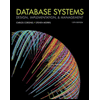
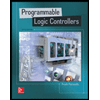