Complete the TODO by finding the locations of the data samples for each iris flower type. Hint: Use the code for how we found all the setosa_locs as an example! Using iris_df, find the location of all the data sample locations for the versicolor class which has a label of 1. To do so, use NumPy's np.where() function. Using iris_df, find the location of all the data sample locations for the virginica class which has a label of 2. To do so, use NumPy's np.where() function. setosa_locs = np.where(iris_df['class'] == 0)[0] # TODO 2.1 versicolor_locs = # TODO 2.2 virginica_locs = todo_check([ (type(versicolor_locs) is np.ndarray, "'versicolor_locs' is not type np.ndarray. Make sure you indexed np.where() currently!"), (type(virginica_locs) is np.ndarray, "'virginica_locs' is not type np.ndarray. Make sure you indexed np.where() currently!"), (versicolor_locs[0] == 50, "'versicolor_locs' has an incorrect value"), (virginica_locs[0] == 100, "'virginica_locs' has an incorrect value"), ])
TODO 2
Complete the TODO by finding the locations of the data samples for each iris flower type.
Hint: Use the code for how we found all the setosa_locs as an example!
-
Using iris_df, find the location of all the data sample locations for the versicolor class which has a label of 1. To do so, use NumPy's np.where() function.
-
Using iris_df, find the location of all the data sample locations for the virginica class which has a label of 2. To do so, use NumPy's np.where() function.
setosa_locs = np.where(iris_df['class'] == 0)[0]
# TODO 2.1
versicolor_locs =
# TODO 2.2
virginica_locs =
todo_check([
(type(versicolor_locs) is np.ndarray, "'versicolor_locs' is not type np.ndarray. Make sure you indexed np.where() currently!"),
(type(virginica_locs) is np.ndarray, "'virginica_locs' is not type np.ndarray. Make sure you indexed np.where() currently!"),
(versicolor_locs[0] == 50, "'versicolor_locs' has an incorrect value"),
(virginica_locs[0] == 100, "'virginica_locs' has an incorrect value"),
])

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

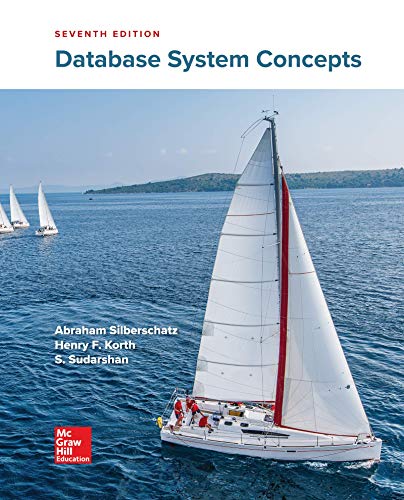
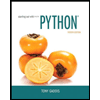
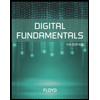
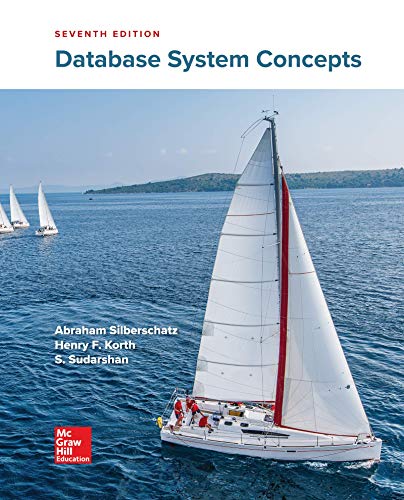
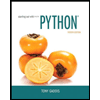
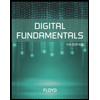
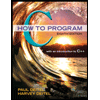
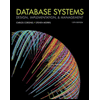
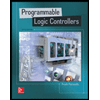