import java.io.File; import java.io.FileReader; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Scanner; public class Client { Scanner sc = new Scanner(System.in); ArrayList students = new ArrayList(); ArrayList courses = new ArrayList(); // Method to read the file contents and stores it in // instance arrays void readStudents() { // Scanner class object declared Scanner readStuF = null; // try block begins try { // Opens the file for reading readStuF = new Scanner(new File("student.txt")); // Loops till end of the file to read records while(readStuF.hasNextLine()) { String stu = readStuF.nextLine(); String []eachStu = stu.split(" "); students.add(new Student(eachStu[0], eachStu[1], eachStu[2], Long.parseLong(eachStu[3]), Integer.parseInt(eachStu[4]), Integer.parseInt(eachStu[5]), Integer.parseInt(eachStu[6]))); }// End of while loop }// End of try block // Catch block to handle file not found exception catch(FileNotFoundException fe) { System.out.println("\n ERROR: Unable to open the file for reading."); }// End of catch block // Close the file readStuF.close(); }// End of method int searchStudentID(long studentID) { for(int c = 0; c < students.size(); c++) if(studentID == students.get(c).getId()) return c; return -1; } void searchStudentName(String name) { int found = -1; for(int c = 0; c < courses.size(); c++) { if(name.equalsIgnoreCase(courses.get(c).getLastName())) { System.out.println(courses.get(c)); found = c; } } if(found == -1) System.out.println("\n ERROR: No student found on name: " + name); } void add() { // Scanner class object declared Scanner readCourseF = null; Scanner readResearchF = null; // try block begins try { // Opens the file for reading readCourseF = new Scanner(new File("CourseworkStudent.txt")); readResearchF = new Scanner(new File("ResearchStudent.txt")); // Loops till end of the file to read records while(readCourseF.hasNextLine() || readResearchF.hasNextLine()) { String course = readCourseF.nextLine(); String []eachCour = course.split(" "); int position = searchStudentID(Long.parseLong(eachCour[0])); if(position != -1) courses.add(new CourseWorkStudent( students.get(position).getTitle(), students.get(position).getFirstName(), students.get(position).getLastName(), students.get(position).getId(), students.get(position).getDay(), students.get(position).getMonth(), students.get(position).getYear(), Float.parseFloat(eachCour[1]), Float.parseFloat(eachCour[2]), Float.parseFloat(eachCour[3]), Float.parseFloat(eachCour[4]))); String reserch = readResearchF.nextLine(); String []eachReserch = reserch.split(" "); position = searchStudentID(Long.parseLong(eachReserch[0])); if(position != -1) courses.add(new ResearchStudent(students.get(position).getTitle(), students.get(position).getFirstName(), students.get(position).getLastName(), students.get(position).getId(), students.get(position).getDay(), students.get(position).getMonth(), students.get(position).getYear(), Float.parseFloat(eachReserch[1]), Float.parseFloat(eachReserch[2]))); }// End of while loop calculateGrade(); }// End of try block // Catch block to handle file not found exception catch(FileNotFoundException fe) { System.out.println("\n ERROR: Unable to open the file for reading."); }// End of catch block // Close the file readCourseF.close(); readResearchF.close(); } I did according to this code which was provided by an expert here and I got the error in the image attached. what went wrong?
import java.io.File;
import java.io.FileReader;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Scanner;
public class Client
{
Scanner sc = new Scanner(System.in);
ArrayList <Student> students = new ArrayList<Student>();
ArrayList <Student> courses = new ArrayList<Student>();
// Method to read the file contents and stores it in
// instance arrays
void readStudents()
{
// Scanner class object declared
Scanner readStuF = null;
// try block begins
try
{
// Opens the file for reading
readStuF = new Scanner(new File("student.txt"));
// Loops till end of the file to read records
while(readStuF.hasNextLine())
{
String stu = readStuF.nextLine();
String []eachStu = stu.split(" ");
students.add(new Student(eachStu[0], eachStu[1],
eachStu[2], Long.parseLong(eachStu[3]),
Integer.parseInt(eachStu[4]), Integer.parseInt(eachStu[5]),
Integer.parseInt(eachStu[6])));
}// End of while loop
}// End of try block
// Catch block to handle file not found exception
catch(FileNotFoundException fe)
{
System.out.println("\n ERROR: Unable to open the file for reading.");
}// End of catch block
// Close the file
readStuF.close();
}// End of method
int searchStudentID(long studentID)
{
for(int c = 0; c < students.size(); c++)
if(studentID == students.get(c).getId())
return c;
return -1;
}
void searchStudentName(String name)
{
int found = -1;
for(int c = 0; c < courses.size(); c++)
{
if(name.equalsIgnoreCase(courses.get(c).getLastName()))
{
System.out.println(courses.get(c));
found = c;
}
}
if(found == -1)
System.out.println("\n ERROR: No student found on name: " + name);
}
void add()
{
// Scanner class object declared
Scanner readCourseF = null;
Scanner readResearchF = null;
// try block begins
try
{
// Opens the file for reading
readCourseF = new Scanner(new File("CourseworkStudent.txt"));
readResearchF = new Scanner(new File("ResearchStudent.txt"));
// Loops till end of the file to read records
while(readCourseF.hasNextLine() || readResearchF.hasNextLine())
{
String course = readCourseF.nextLine();
String []eachCour = course.split(" ");
int position = searchStudentID(Long.parseLong(eachCour[0]));
if(position != -1)
courses.add(new CourseWorkStudent(
students.get(position).getTitle(),
students.get(position).getFirstName(),
students.get(position).getLastName(),
students.get(position).getId(),
students.get(position).getDay(),
students.get(position).getMonth(),
students.get(position).getYear(),
Float.parseFloat(eachCour[1]),
Float.parseFloat(eachCour[2]),
Float.parseFloat(eachCour[3]),
Float.parseFloat(eachCour[4])));
String reserch = readResearchF.nextLine();
String []eachReserch = reserch.split(" ");
position = searchStudentID(Long.parseLong(eachReserch[0]));
if(position != -1)
courses.add(new ResearchStudent(students.get(position).getTitle(),
students.get(position).getFirstName(),
students.get(position).getLastName(),
students.get(position).getId(),
students.get(position).getDay(),
students.get(position).getMonth(),
students.get(position).getYear(),
Float.parseFloat(eachReserch[1]),
Float.parseFloat(eachReserch[2])));
}// End of while loop
calculateGrade();
}// End of try block
// Catch block to handle file not found exception
catch(FileNotFoundException fe)
{
System.out.println("\n ERROR: Unable to open the file for reading.");
}// End of catch block
// Close the file
readCourseF.close();
readResearchF.close();
}
I did according to this code which was provided by an expert here and I got the error in the image attached. what went wrong?
![e Console X
<terminated> Client (1) [Java Application] C:\Program Files\Java\jdk-16.0.1\bin\javaw.exe (30 Jul 2021, 12:09:14 am – 12:09:15 am)
Exception in thread "main"
ERROR: Unable to open the file for reading.
java.lang. NullPointerException: Cannot invoke "java.util.Scanner.close()" because "readStuf" is null
at Client.readStudents (Client.java:47)
at Client.main(Client.java:281)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbceba861-5172-42f7-bf19-945c9a97d1a1%2F5e7a464e-4271-410e-9b94-74881d9d7747%2F3uu0z7n_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

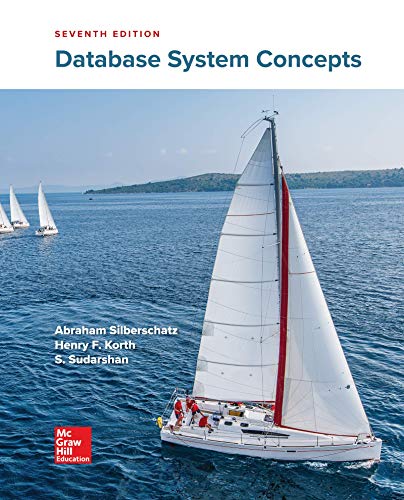
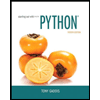
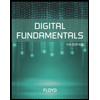
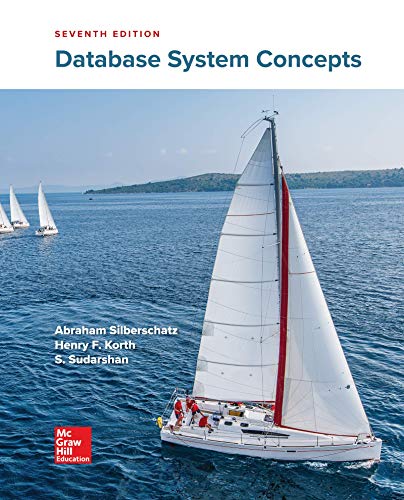
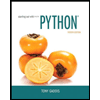
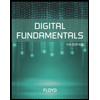
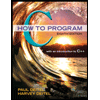
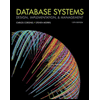
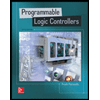