I have the following code in JAVA: package com.mycompany.test; /** * * @author */ import java.util.ArrayList; import java.util.Scanner; public class Test { static ArrayList animales = new ArrayList<>(); /** * * @param args */ static void imprimirAnimales() { for (Animal animal : animales) { System.out.println("Nombre: " + animal.getNombre() + ", Especie: " + animal.getEspecie() + ", Edad: " + animal.getEdad()); } } public static void main(String[] args) { Scanner scanner = new Scanner(System.in); int opcion = 0; while (opcion != 5) { System.out.println("¿Qué acción desea realizar?"); System.out.println("1. Añadir animal"); System.out.println("2. Modificar animal"); System.out.println("3. Eliminar animal"); System.out.println("4. Imprimir lista de animales"); System.out.println("5. Salir"); opcion = scanner.nextInt(); switch (opcion) { case 1: agregarAnimal(scanner); break; case 2: modificarAnimal(scanner); break; case 3: eliminarAnimal(scanner); break; case 4: imprimirAnimales(); break; case 5: break; default: System.out.println("Opción inválida"); break; } } } static void agregarAnimal(Scanner scanner) { System.out.println("Ingrese el nombre del animal:"); String nombre = scanner.next(); System.out.println("Ingrese la especie del animal:"); String especie = scanner.next(); System.out.println("Ingrese la edad del animal:"); int edad = scanner.nextInt(); Animal animal = new Animal(nombre, especie, edad); animales.add(animal); System.out.println("Animal agregado correctamente."); } static void modificarAnimal(Scanner scanner) { System.out.println("Ingrese el índice del animal a modificar:"); int indice = scanner.nextInt(); if (indice >= 0 && indice < animales.size()) { Animal animal = animales.get(indice); System.out.println("Ingrese el nuevo nombre del animal:"); String nombre = scanner.next(); System.out.println("Ingrese la nueva especie del animal:"); String especie = scanner.next(); System.out.println("Ingrese la nueva edad del animal:"); String edad = scanner.next(); animal.setNombre(nombre); animal.setEspecie(especie); animal.setEdad(Integer.parseInt(edad)); System.out.println("Animal modificado correctamente."); } else { System.out.println("Índice inválido."); } } static void eliminarAnimal(Scanner scanner) { System.out.println("Ingrese el índice del animal a eliminar:"); int indice = scanner.nextInt(); if (indice >= 0 && indice < animales.size()) { animales.remove(indice); System.out.println("Animal eliminado correctamente."); } else { System.out.println("Índice inválido."); } } } class Animal { private String nombre; private String especie; private int edad; public Animal(String nombre, String especie, int edad) { this.nombre = nombre; this.especie = especie; this.edad = edad; } public String getNombre() { return nombre; } public void setNombre(String nombre) { this.nombre = nombre; } public String getEspecie() { return especie; } public void setEspecie(String especie){ this.especie = especie; } public int getEdad() { return edad; } public void setEdad(int edad) { this.edad = edad; } } The code runs perfectly to ask the user if he wants to add, remove or modify an animal. However, I want this code to be called from another class that is located in the same package. How can I do it? The new class must contain an output when it is executed that asks the user if he wants to enter animals, consult data or consult cash counting. If the option is 1, then the above code located in another class is executed
I have the following code in JAVA:
package com.mycompany.test;
/**
*
* @author
*/
import java.util.ArrayList;
import java.util.Scanner;
public class Test {
static ArrayList<Animal> animales = new ArrayList<>();
/**
*
* @param args
*/
static void imprimirAnimales() {
for (Animal animal : animales) {
System.out.println("Nombre: " + animal.getNombre() + ", Especie: " + animal.getEspecie() + ", Edad: " + animal.getEdad());
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int opcion = 0;
while (opcion != 5) {
System.out.println("¿Qué acción desea realizar?");
System.out.println("1. Añadir animal");
System.out.println("2. Modificar animal");
System.out.println("3. Eliminar animal");
System.out.println("4. Imprimir lista de animales");
System.out.println("5. Salir");
opcion = scanner.nextInt();
switch (opcion) {
case 1:
agregarAnimal(scanner);
break;
case 2:
modificarAnimal(scanner);
break;
case 3:
eliminarAnimal(scanner);
break;
case 4:
imprimirAnimales();
break;
case 5:
break;
default:
System.out.println("Opción inválida");
break;
}
}
}
static void agregarAnimal(Scanner scanner) {
System.out.println("Ingrese el nombre del animal:");
String nombre = scanner.next();
System.out.println("Ingrese la especie del animal:");
String especie = scanner.next();
System.out.println("Ingrese la edad del animal:");
int edad = scanner.nextInt();
Animal animal = new Animal(nombre, especie, edad);
animales.add(animal);
System.out.println("Animal agregado correctamente.");
}
static void modificarAnimal(Scanner scanner) {
System.out.println("Ingrese el índice del animal a modificar:");
int indice = scanner.nextInt();
if (indice >= 0 && indice < animales.size()) {
Animal animal = animales.get(indice);
System.out.println("Ingrese el nuevo nombre del animal:");
String nombre = scanner.next();
System.out.println("Ingrese la nueva especie del animal:");
String especie = scanner.next();
System.out.println("Ingrese la nueva edad del animal:");
String edad = scanner.next();
animal.setNombre(nombre);
animal.setEspecie(especie);
animal.setEdad(Integer.parseInt(edad));
System.out.println("Animal modificado correctamente.");
} else {
System.out.println("Índice inválido.");
}
}
static void eliminarAnimal(Scanner scanner) {
System.out.println("Ingrese el índice del animal a eliminar:");
int indice = scanner.nextInt();
if (indice >= 0 && indice < animales.size()) {
animales.remove(indice);
System.out.println("Animal eliminado correctamente.");
} else {
System.out.println("Índice inválido.");
}
}
}
class Animal {
private String nombre;
private String especie;
private int edad;
public Animal(String nombre, String especie, int edad) {
this.nombre = nombre;
this.especie = especie;
this.edad = edad;
}
public String getNombre() {
return nombre;
}
public void setNombre(String nombre) {
this.nombre = nombre;
}
public String getEspecie() {
return especie;
}
public void setEspecie(String especie){
this.especie = especie;
}
public int getEdad() {
return edad;
}
public void setEdad(int edad) {
this.edad = edad;
}
}
The code runs perfectly to ask the user if he wants to add, remove or modify an animal.
However, I want this code to be called from another class that is located in the same package. How can I do it? The new class must contain an output when it is executed that asks the user if he wants to enter animals, consult data or consult cash counting. If the option is 1, then the above code located in another class is executed

Step by step
Solved in 3 steps with 3 images

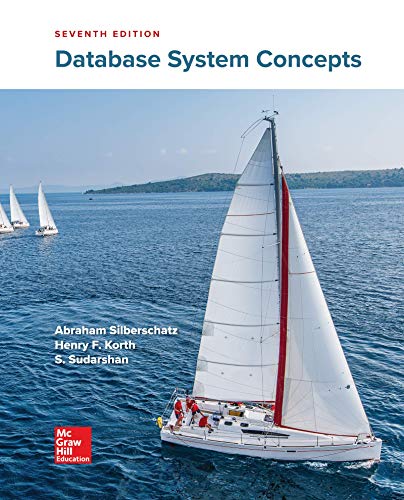
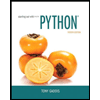
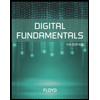
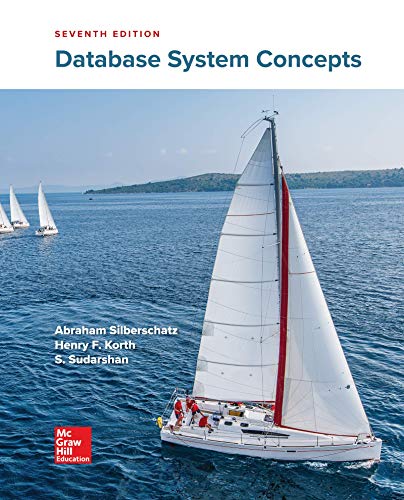
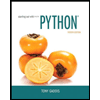
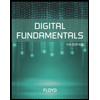
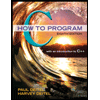
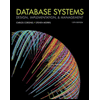
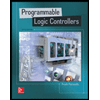