• If you do not have a copy constructor function for Date , add it to the class definition, which is in the following form o Date (const Date &src) • Modify the constructor functions of the Date class (including the copy constructor function mentioned above) to add a line of code to print The constructor function is executed on the screen. • Write two functions that accept an Date object as parameter void f(Date &d); void g(Date d); • For both f and g, call the member function print . Please observe the execution results of f and g, and answer the following two questions: • What is the difference when you run function f and g ? • What is the reason for the difference?
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Based on this code, can you add what is in the picture to it?
#include <iostream>
#include <stdlib.h>
#include <string>
using namespace std;
// Declare the class
class Date
{
// Declare the private members of class
private:
int day_of_month, month, year;
// Declare the public members of the class.
public:
Date()
{
// initialize the variables.
day_of_month = 1;
month = 1;
year = 2001;
}
Date(int d, int m, int y)
{
// Initialized the values.
day_of_month = d;
month = m;
year = y;
}
// Definition of the function.
void printNumerical()
{
cout << month << "/" << day_of_month << "/" << year << endl;
}
// Definition of the function.
void printMonthFirst()
{
cout << getMonth(month) << " " << day_of_month << ", " << year << endl;
}
// Definition of the function
void printDateFirst()
{
cout << day_of_month << " " << getMonth(month) << " " << year << endl;
}
// Definition of the function
string getMonth(int month) {
if (month == 1) {
return "January";
}
else if (month == 2) {
return "February";
}
else if (month == 3) {
return "March";
}
else if (month == 4) {
return "April";
}
else if (month == 5) {
return "May";
}
else if (month == 6) {
return "June";
}
else if (month == 7) {
return "July";
}
else if (month == 8) {
return "August";
}
else if (month == 9) {
return "September";
}
else if (month == 10) {
return "October";
}
else if (month == 11) {
return "November";
}
else if (month == 12) {
return "December";
}
return 0;
}
};
// Declare the main method.
int main()
{
int day_of_month, month, year;
// Prompt the user to enter the date of month.
cout << "Enter the day of month: ";
cin >> day_of_month;
// Prompt the user to enter the month.
cout << "Enter the month: ";
cin >> month;
// Prompt the user to enter the year.
cout << "Enter the year: ";
cin >> year;
cout << endl;
// Check whether the condition are valid or not.
if (day_of_month < 1 || day_of_month > 31 || month < 1 || month > 12 || year<0)
{
// Create the object of class.
Date d1;
// Call to the functions
d1.printNumerical();
d1.printMonthFirst();
d1.printDateFirst();
}
else
{
// Create the object of class.
Date d2(day_of_month, month, year);
// Call to the functions
d2.printNumerical();
d2.printMonthFirst();
d2.printDateFirst();
}
return 0;
}
.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F72b83cc2-495e-4bc5-a028-f79dc88fc308%2F287f43ad-e88e-4747-bfdb-8ad67046edfd%2Fsebp25c_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

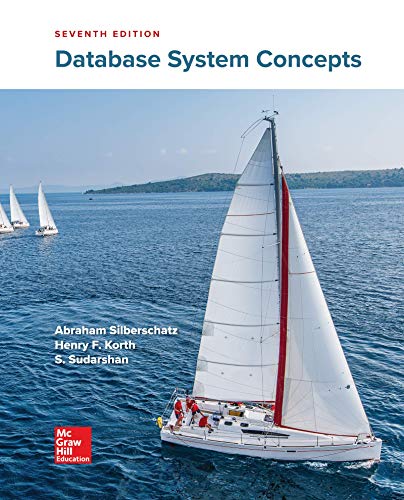
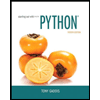
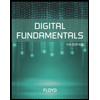
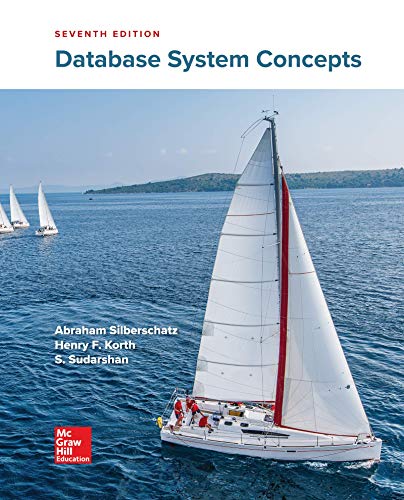
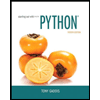
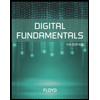
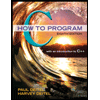
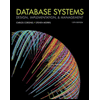
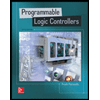