I want to show the result better (I mean the one in the red box only). as in attached Thank you. >>>>>>>>>>>>>>>>>>>>>>>>>>>> using System; public class Rectangle { double width, length, coorx, coory; public Rectangle() { width = 1; length = 1; coorx = 0; coory = 0; } public void move(double x, double y) { coorx += x; coory += y; } public double getArea() { return width * length; } public double getPerimeter() { return 2 * (width + length); } public override string ToString() { return "Rectangle with width: " + width.ToString() + ", length: " + length.ToString() + " with its center at : (" + coorx.ToString() + ", " + coory.ToString() + ")\n"; } public static void Main() { Rectangle[] arr = new Rectangle[2]; arr[0] = new Rectangle(); arr[1] = new Rectangle(); Console.WriteLine(arr[0]); Console.WriteLine(arr[1]); arr[0].move(1, 1); Console.WriteLine(arr[0]); Console.WriteLine(arr[0].getArea()); Console.WriteLine(arr[0].getPerimeter()); Console.WriteLine(arr[1].getArea()); Console.WriteLine(arr[1].getPerimeter()); } }
I have this code (C#):
I want to show the result better (I mean the one in the red box only).
as in attached
Thank you.
>>>>>>>>>>>>>>>>>>>>>>>>>>>>
using System;
public class Rectangle
{
double width, length, coorx, coory;
public Rectangle()
{
width = 1;
length = 1;
coorx = 0;
coory = 0;
}
public void move(double x, double y)
{
coorx += x;
coory += y;
}
public double getArea()
{
return width * length;
}
public double getPerimeter()
{
return 2 * (width + length);
}
public override string ToString()
{
return "Rectangle with width: " + width.ToString() + ", length: " + length.ToString() + " with its center at : (" + coorx.ToString() + ", " + coory.ToString() + ")\n";
}
public static void Main()
{
Rectangle[] arr = new Rectangle[2];
arr[0] = new Rectangle();
arr[1] = new Rectangle();
Console.WriteLine(arr[0]);
Console.WriteLine(arr[1]);
arr[0].move(1, 1);
Console.WriteLine(arr[0]);
Console.WriteLine(arr[0].getArea());
Console.WriteLine(arr[0].getPerimeter());
Console.WriteLine(arr[1].getArea());
Console.WriteLine(arr[1].getPerimeter());
}
}
>>>>>>>>>>>>>>>>>>>>>>>>>>>>


Step by step
Solved in 3 steps with 1 images

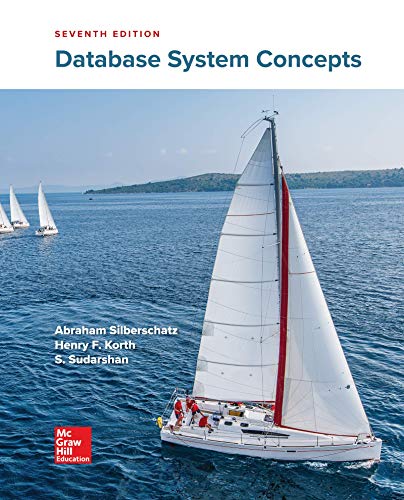
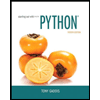
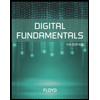
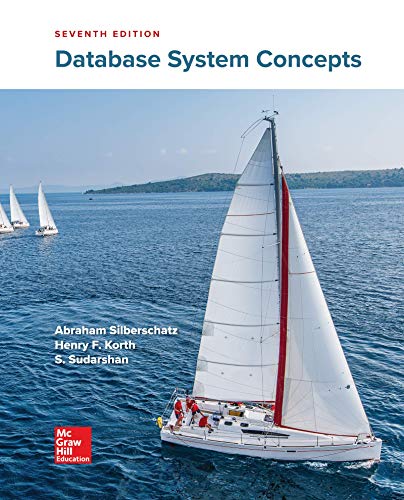
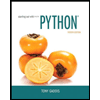
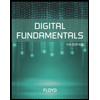
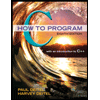
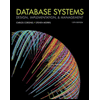
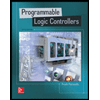