. Write code to read in from the console, a set of numbers, each separated by a newline (each number is on its own line). The user will terminate the input with the sentinel value -666. a) Write the code to read in the values and store them into a data structure. Also, create a menu that will have 4 options. Selecting “a” should cause the computer to read input from the user, selecting “d” will cause the program to quit.: a. Enter/Re-enter Data b. Sort c. List All d. Quit b) Write a function that will output the current contents of the data structure to the console window and call that function from the menu (as appropriate) c) Write a function, InsertionSort, to sort the data entered by the user using the following algorithm, given in pseudo-code: for i ← 1 to length(A) - 1 j ← i while j > 0 and A[j-1] > A[j] swap A[j] and A[j-1] j ← j - 1 end while end for Link the sorting algorithm to your menu. Make yourself a little array of values.
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Please help with this code and make sure to create in c++. Make sure it is 100% correct and works on Visual studios. Thanks.
Write the code as described in the questions below. Submit your code electronically in the box at the bottom. You may only use C++.
1. Write code to read in from the console, a set of numbers, each separated by a newline (each number is on its own line). The user will terminate the input with the sentinel value -666.
a) Write the code to read in the values and store them into a data structure. Also, create a menu that will have 4 options. Selecting “a” should cause the computer to read input from the user, selecting “d” will cause the program to quit.:
a. Enter/Re-enter Data
b. Sort
c. List All
d. Quit
b) Write a function that will output the current contents of the data structure to the console window and call that function from the menu (as appropriate)
c) Write a function, InsertionSort, to sort the data entered by the user using the following
for i ← 1 to length(A) - 1
j ← i
while j > 0 and A[j-1] > A[j]
swap A[j] and A[j-1]
j ← j - 1
end while
end for
Link the sorting algorithm to your menu.
Make yourself a little array of values.

Step by step
Solved in 4 steps with 3 images

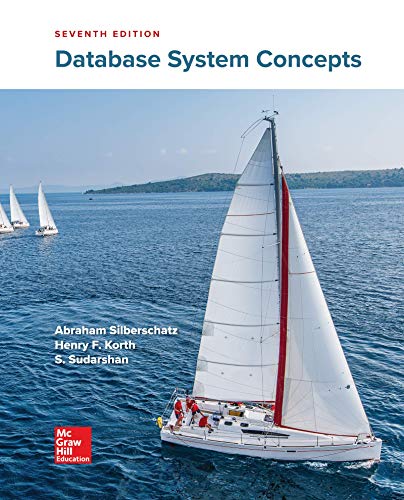
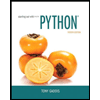
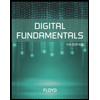
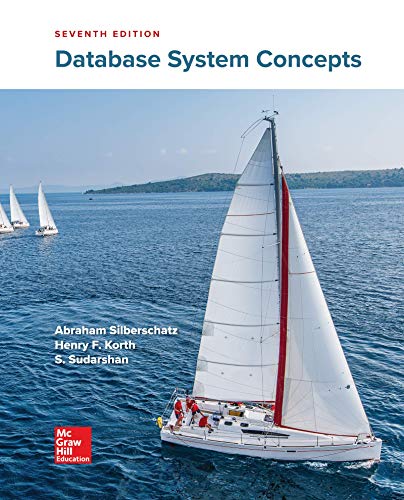
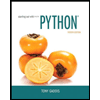
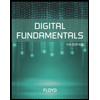
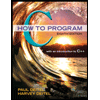
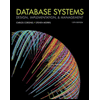
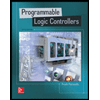