I need help in converting the following Java code related to BST to a pseudocode. public static class Node { //instance variable of Node class public String data; public Node left; public Node right; //constructor public Node(String data) { this.data = data; this.left = null; this.right = null; } } public void deleteANode(Node node) { deleteNode(this.root, node); } private Node deleteNode(Node root, Node node) { // check for node initially if (root == null) { return null; } else if (node.data.length() < root.data.length()) { // process the left sub tree root.left = deleteNode(root.left, node); } else if (node.data.length() > root.data.length()) { // process the right sub tree root.right = deleteNode(root.right, node); } else if(root.data==node.data){ // case 3: 2 child if (root.left != null && root.right != null) { String lmax = findMaxData(root.left); root.data = lmax; root.left = deleteNode(root.left, new Node(lmax)); return root; } //case 2: one child // case i-> has only left child else if (root.left != null) { return root.left; } // case ii-> has only right child else if (root.right != null) { return root.right; } //case 1:- no child else { return null; } } return root; } // inorder successor of given node public String findMaxData(Node root) { if (root.right != null) { return findMaxData(root.right); } else { return root.data; } }
I need help in converting the following Java code related to BST to a pseudocode.
public static class Node {
//instance variable of Node class
public String data;
public Node left;
public Node right;
//constructor
public Node(String data) {
this.data = data;
this.left = null;
this.right = null;
}
}
public void deleteANode(Node node) {
deleteNode(this.root, node);
}
private Node deleteNode(Node root, Node node) {
// check for node initially
if (root == null) {
return null;
} else if (node.data.length() < root.data.length()) {
// process the left sub tree
root.left = deleteNode(root.left, node);
} else if (node.data.length() > root.data.length()) {
// process the right sub tree
root.right = deleteNode(root.right, node);
} else if(root.data==node.data){
// case 3: 2 child
if (root.left != null && root.right != null) {
String lmax = findMaxData(root.left);
root.data = lmax;
root.left = deleteNode(root.left, new Node(lmax));
return root;
}
//case 2: one child
// case i-> has only left child
else if (root.left != null) {
return root.left;
}
// case ii-> has only right child
else if (root.right != null) {
return root.right;
}
//case 1:- no child
else {
return null;
}
}
return root;
}
// inorder successor of given node
public String findMaxData(Node root) {
if (root.right != null) {
return findMaxData(root.right);
} else {
return root.data;
}
}

Step by step
Solved in 2 steps

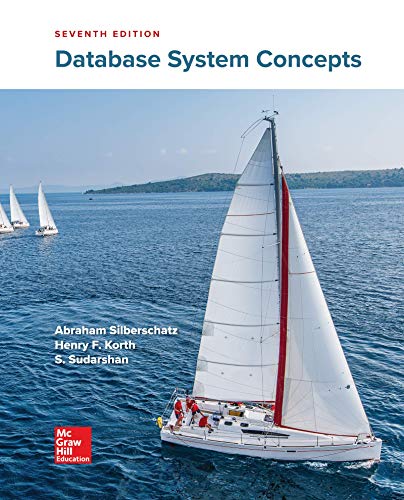
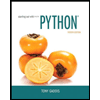
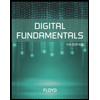
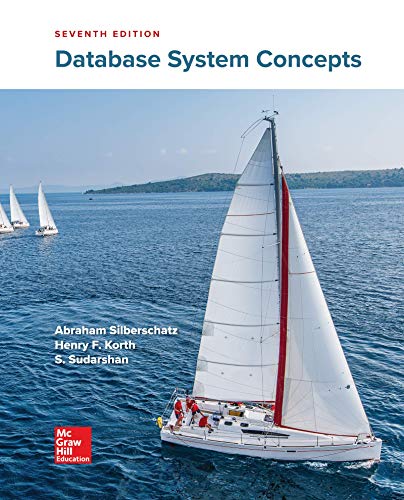
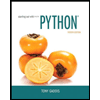
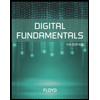
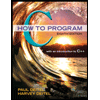
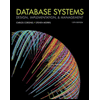
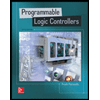