i need documentation this code using System;using System.Collections.Generic;using System.ComponentModel;using System.Data;using System.Drawing;using System.Linq;using System.Text;using System.Threading.Tasks;using System.Windows.Forms; namespace WindowsFormsApp3{ public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { // Get the dimensions of the PictureBox int width = pictureBox1.Width; int height = pictureBox1.Height; // Create a new Bitmap object with the same dimensions as the PictureBox Bitmap bmp = new Bitmap(width, height); // Create a Graphics object from the Bitmap to draw on it using (Graphics g = Graphics.FromImage(bmp)) { // Clear the Bitmap to white background g.Clear(Color.White); // Create a Pen to draw the sine wave, black color and 2 pixels wide Pen pen = new Pen(Color.Black, 2); // Scale factor for the sine wave amplitude int scale = 20; // Step for the x-values, calculated to fit several cycles in the PictureBox double step = (Math.PI * 2 / width) * 4; // Iterate through each x-coordinate in the width of the PictureBox for (int x = 0; x < width; x++) { // Calculate the corresponding y-coordinate using the sine function int y = (int)(height / 2.0 + scale * Math.Sin(x * step)); // Set the pixel at (x, y) to the color of the pen bmp.SetPixel(x, y, pen.Color); } } // Set the Bitmap as the image of the PictureBox to display it pictureBox1.Image = bmp; } private void button2_Click(object sender, EventArgs e) { // Clear the PictureBox by setting its Image property to null pictureBox1.Image = null; } } }
i need documentation this code
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp3
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
// Get the dimensions of the PictureBox
int width = pictureBox1.Width;
int height = pictureBox1.Height;
// Create a new Bitmap object with the same dimensions as the PictureBox
Bitmap bmp = new Bitmap(width, height);
// Create a Graphics object from the Bitmap to draw on it
using (Graphics g = Graphics.FromImage(bmp))
{
// Clear the Bitmap to white background
g.Clear(Color.White);
// Create a Pen to draw the sine wave, black color and 2 pixels wide
Pen pen = new Pen(Color.Black, 2);
// Scale factor for the sine wave amplitude
int scale = 20;
// Step for the x-values, calculated to fit several cycles in the PictureBox
double step = (Math.PI * 2 / width) * 4;
// Iterate through each x-coordinate in the width of the PictureBox
for (int x = 0; x < width; x++)
{
// Calculate the corresponding y-coordinate using the sine function
int y = (int)(height / 2.0 + scale * Math.Sin(x * step));
// Set the pixel at (x, y) to the color of the pen
bmp.SetPixel(x, y, pen.Color);
}
}
// Set the Bitmap as the image of the PictureBox to display it
pictureBox1.Image = bmp;
}
private void button2_Click(object sender, EventArgs e)
{
// Clear the PictureBox by setting its Image property to null
pictureBox1.Image = null;
}
}
}
Unlock instant AI solutions
Tap the button
to generate a solution
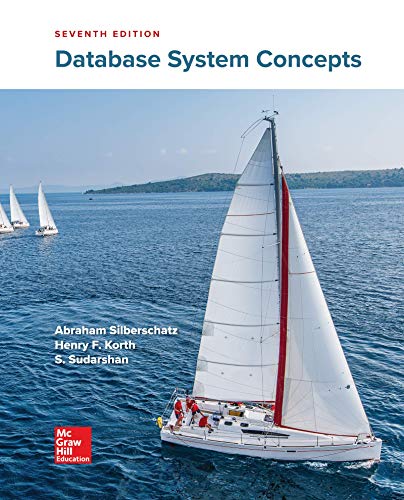
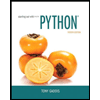
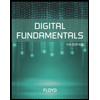
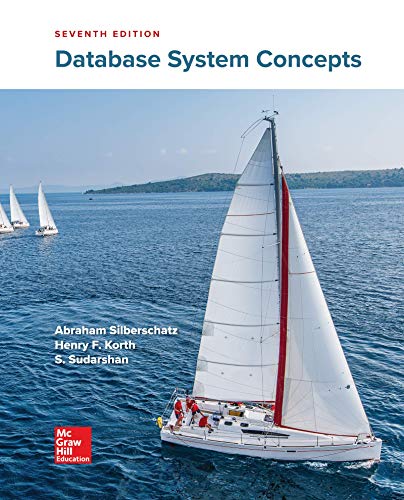
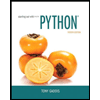
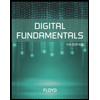
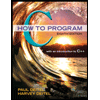
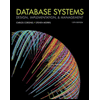
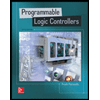