xplain the functionality of each line of code in the provided C# scripts- using System.Collections; using System.Collections.Generic; using UnityEngine; public class Movement2D : MonoBehaviour { public float moveSpeed = 3f; public float jumpForce = 5f; public bool isGrounded; private bool doubleJump; private bool canDash = true; private bool isDashing; private float dashingPower = 24f; private float dashingTime = .2f; private float dashingCooldown = 1f; private bool isWallJumping; private float wallJumpingDirection; private float wallJumpingTime; private float wallJumpingCounter; private float wallJumpingDuration = .04f; private Vector2 wallJumpingPower = new Vector2(8f, 16f); [SerializeField] private Rigidbody2D rb; [SerializeField] private TrailRenderer tr; [SerializeField] private Transform wallCheck; [SerializeField] private LayerMask wallLayer; private SpriteRenderer sprite; public void Start() { sprite = gameObject.GetComponent(); } private void Update() { if (isDashing) { return; } WallJump(); Jump(); Vector3 movement = new Vector3(Input.GetAxis("Horizontal"), 0f, 0f); transform.position += movement * Time.deltaTime * moveSpeed; if (Input.GetAxisRaw("Horizontal") < 0) { sprite.flipX = true; } else if (Input.GetAxisRaw("Horizontal") > 0) { sprite.flipX = false; } if (Input.GetKeyDown(KeyCode.LeftShift) && canDash) { StartCoroutine(Dash()); } if (!isWallJumping) { rb.velocity = new Vector2(Input.GetAxisRaw("Horizontal") * moveSpeed, rb.velocity.y); } } void Jump() { if (Input.GetButtonDown("Jump") && isGrounded) { gameObject.GetComponent().velocity = (new Vector2(0f, jumpForce)); doubleJump = true; } else if (Input.GetButtonDown("Jump") && doubleJump) { gameObject.GetComponent().velocity = (new Vector2(0f, jumpForce)); doubleJump = false; } } private IEnumerator Dash() { canDash = false; isDashing = true; float orignialGravity = rb.gravityScale; rb.gravityScale = 0f; rb.velocity = new Vector3(Input.GetAxis("Horizontal") * dashingPower, 0f); tr.emitting = true; yield return new WaitForSeconds(dashingTime); tr.emitting = false; rb.gravityScale = orignialGravity; isDashing = false; yield return new WaitForSeconds(dashingCooldown); canDash = true; } private void WallJump() { if (Input.GetButtonDown("Jump") && wallJumpingCounter > 0f) { isWallJumping = true; rb.velocity = new Vector2(wallJumpingDirection * wallJumpingPower.x, wallJumpingPower.y); wallJumpingCounter = 0f; if (Input.GetAxisRaw("Horizontal") != wallJumpingDirection) { sprite.flipX = !sprite.flipX; } Invoke(nameof(StopWallJumping), wallJumpingDuration); } } private void StopWallJumping() { isWallJumping = false; } } using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.Video; [RequireComponent(typeof(VideoPlayer))] [RequireComponent(typeof(AudioSource))] public class PlayVideo : MonoBehaviour { public VideoClip videoClip; private VideoPlayer videoPlayer; private AudioSource audioSource; void Start() { videoPlayer = GetComponent(); audioSource = GetComponent(); videoPlayer.playOnAwake = false; audioSource.playOnAwake = false; videoPlayer.source = VideoSource.VideoClip; videoPlayer.clip = videoClip; videoPlayer.audioOutputMode = VideoAudioOutputMode.AudioSource; videoPlayer.SetTargetAudioSource(0, audioSource); videoPlayer.renderMode = VideoRenderMode.MaterialOverride; videoPlayer.targetMaterialRenderer = GetComponent(); videoPlayer.targetMaterialProperty = "_MainTex"; } void Update() { if (Input.GetButtonDown("Jump")) PlayPause(); } private void PlayPause() { if (videoPlayer.isPlaying) videoPlayer.Pause(); else videoPlayer.Play(); } } using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.SceneManagement; public class NewBehaviourScript : MonoBehaviour { // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { } public void LoadOnClick (int sceneIndex) { SceneManager.LoadScene(sceneIndex); }
Explain the functionality of each line of code in the provided C# scripts-
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Movement2D : MonoBehaviour
{
public float moveSpeed = 3f;
public float jumpForce = 5f;
public bool isGrounded;
private bool doubleJump;
private bool canDash = true;
private bool isDashing;
private float dashingPower = 24f;
private float dashingTime = .2f;
private float dashingCooldown = 1f;
private bool isWallJumping;
private float wallJumpingDirection;
private float wallJumpingTime;
private float wallJumpingCounter;
private float wallJumpingDuration = .04f;
private Vector2 wallJumpingPower = new Vector2(8f, 16f);
[SerializeField] private Rigidbody2D rb;
[SerializeField] private TrailRenderer tr;
[SerializeField] private Transform wallCheck;
[SerializeField] private LayerMask wallLayer;
private SpriteRenderer sprite;
public void Start()
{
sprite = gameObject.GetComponent<SpriteRenderer>();
}
private void Update()
{
if (isDashing)
{
return;
}
WallJump();
Jump();
Vector3 movement = new Vector3(Input.GetAxis("Horizontal"), 0f, 0f);
transform.position += movement * Time.deltaTime * moveSpeed;
if (Input.GetAxisRaw("Horizontal") < 0)
{
sprite.flipX = true;
}
else if (Input.GetAxisRaw("Horizontal") > 0)
{
sprite.flipX = false;
}
if (Input.GetKeyDown(KeyCode.LeftShift) && canDash)
{
StartCoroutine(Dash());
}
if (!isWallJumping)
{
rb.velocity = new Vector2(Input.GetAxisRaw("Horizontal") * moveSpeed, rb.velocity.y);
}
}
void Jump()
{
if (Input.GetButtonDown("Jump") && isGrounded)
{
gameObject.GetComponent<Rigidbody2D>().velocity = (new Vector2(0f, jumpForce));
doubleJump = true;
}
else if (Input.GetButtonDown("Jump") && doubleJump)
{
gameObject.GetComponent<Rigidbody2D>().velocity = (new Vector2(0f, jumpForce));
doubleJump = false;
}
}
private IEnumerator Dash()
{
canDash = false;
isDashing = true;
float orignialGravity = rb.gravityScale;
rb.gravityScale = 0f;
rb.velocity = new Vector3(Input.GetAxis("Horizontal") * dashingPower, 0f);
tr.emitting = true;
yield return new WaitForSeconds(dashingTime);
tr.emitting = false;
rb.gravityScale = orignialGravity;
isDashing = false;
yield return new WaitForSeconds(dashingCooldown);
canDash = true;
}
private void WallJump()
{
if (Input.GetButtonDown("Jump") && wallJumpingCounter > 0f)
{
isWallJumping = true;
rb.velocity = new Vector2(wallJumpingDirection * wallJumpingPower.x, wallJumpingPower.y);
wallJumpingCounter = 0f;
if (Input.GetAxisRaw("Horizontal") != wallJumpingDirection)
{
sprite.flipX = !sprite.flipX;
}
Invoke(nameof(StopWallJumping), wallJumpingDuration);
}
}
private void StopWallJumping()
{
isWallJumping = false;
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Video;
[RequireComponent(typeof(VideoPlayer))]
[RequireComponent(typeof(AudioSource))]
public class PlayVideo : MonoBehaviour
{
public VideoClip videoClip;
private VideoPlayer videoPlayer;
private AudioSource audioSource;
void Start()
{
videoPlayer = GetComponent<VideoPlayer>();
audioSource = GetComponent<AudioSource>();
videoPlayer.playOnAwake = false;
audioSource.playOnAwake = false;
videoPlayer.source = VideoSource.VideoClip;
videoPlayer.clip = videoClip;
videoPlayer.audioOutputMode = VideoAudioOutputMode.AudioSource;
videoPlayer.SetTargetAudioSource(0, audioSource);
videoPlayer.renderMode = VideoRenderMode.MaterialOverride;
videoPlayer.targetMaterialRenderer = GetComponent<Renderer>();
videoPlayer.targetMaterialProperty = "_MainTex";
}
void Update()
{
if (Input.GetButtonDown("Jump"))
PlayPause();
}
private void PlayPause()
{
if (videoPlayer.isPlaying)
videoPlayer.Pause();
else
videoPlayer.Play();
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class NewBehaviourScript : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
public void LoadOnClick (int sceneIndex)
{
SceneManager.LoadScene(sceneIndex);
}
}

The first script can be defined in such a way that it controls 2D character motion, such as leaping, dashing, and wall jumping, even as dealing with consumer input and managing movement parameters.
The second script can be defined in such a way that it lets in for video playback manipulation in unity, such as playing and pausing a certain video clip using the VideoPlayer component.
The third script can be defined in such a way that it offers a simple functionality to load a brand new scene in unity based on a specified scene index.
Step by step
Solved in 5 steps

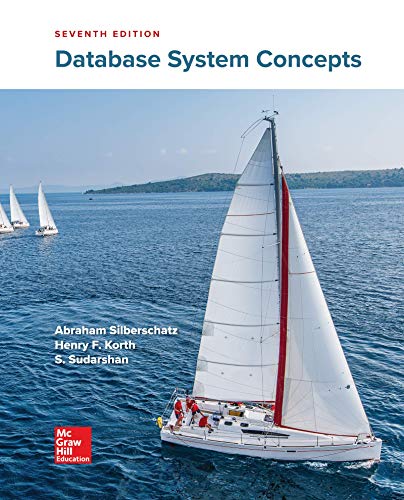
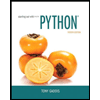
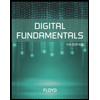
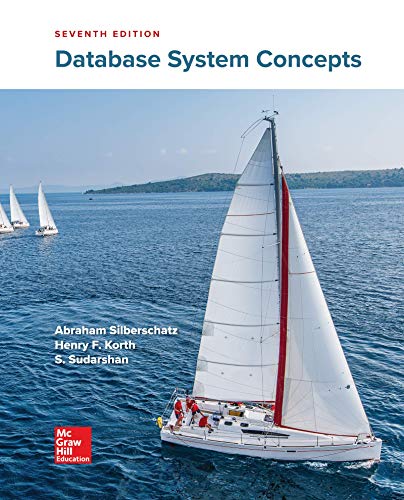
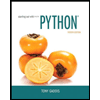
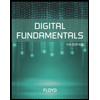
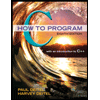
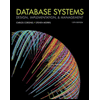
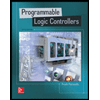