MUST BE DONE IN C#!!! Create a class called StudentGrades. This class should be designed based off the following UML Diagram: class StudentGrades -double list grades_list + +AddGrade(double): void +RemoveGrade(double): bool +GetClassAverage(): double +GetHighestGrade(): double +GetLowestGrade(): double Method Descriptions: a. - creates an empty array for the grades_list array. b. AddGrade – Adds the passed in grade to the list. Note that any number that is NOT 0 – 100 should be rejected. c. RemoveGrade – Removes the first occurrence of the passed in grade from the list. If the grade is not found, the method should return false. d. GetClassAverage() – Average the grades in the list and return the average. e. GetHighestGrade - Find and return the highest grade in the list. f. GetLowestGrade – Find and return the lowest grade in the list. In main, create a StudentGrades object to test the listed methods. Add 10 grades randomly and call each method to test your class.
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
MUST BE DONE IN C#!!!
Create a class called StudentGrades. This class should be designed based off the following UML Diagram:
class StudentGrades
-double list grades_list
+<constructor>
+AddGrade(double): void
+RemoveGrade(double): bool
+GetClassAverage(): double
+GetHighestGrade(): double
+GetLowestGrade(): double
Method Descriptions:
a. <constructor> - creates an empty array for the grades_list array.
b. AddGrade – Adds the passed in grade to the list. Note that any number that is NOT 0 – 100 should be rejected.
c. RemoveGrade – Removes the first occurrence of the passed in grade from the list. If the grade is not found, the method should return false.
d. GetClassAverage() – Average the grades in the list and return the average.
e. GetHighestGrade - Find and return the highest grade in the list.
f. GetLowestGrade – Find and return the lowest grade in the list.
In main, create a StudentGrades object to test the listed methods. Add 10 grades randomly and call each method to test your class.

Algorithm:
1. Create a StudentGrades class with a List of double grades_list as a property, and define a constructor.
2. Create a method named AddGrade that takes a double grade as a parameter.
3. In AddGrade, check if the grade is between 0 and 100.
4. If the grade is between 0 and 100, add it to the grades_list and print a message indicating that the grade was added.
5. If the grade is not between 0 and 100, print a message indicating that the grade is invalid and cannot be added.
6. Create a method named RemoveGrade that takes a double grade as a parameter.
7. In RemoveGrade, check if the grade is in the grades_list.
8. If the grade is in the grades_list, remove it and print a message indicating that the grade was removed.
9. If the grade is not in the grades_list, print a message indicating that the grade was not found in the list.
10. Create a method named GetClassAverage that calculates the average of all grades in the grades_list and returns the result as a double.
11. Create a method named GetHighestGrade that finds the highest grade in the grades_list and returns the result as a double.
12. Create a method named GetLowestGrade that finds the lowest grade in the grades_list and returns the result as a double.
13. In Main, create a StudentGrades object and add 10 grades to the grades_list.
14. Calculate the class average, highest grade, and lowest grade with the methods from the StudentGrades object.
15. Remove the grade 75.7 from the grades_list with the RemoveGrade method.
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

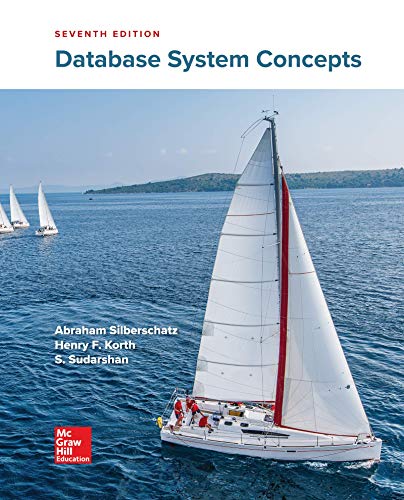
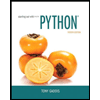
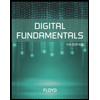
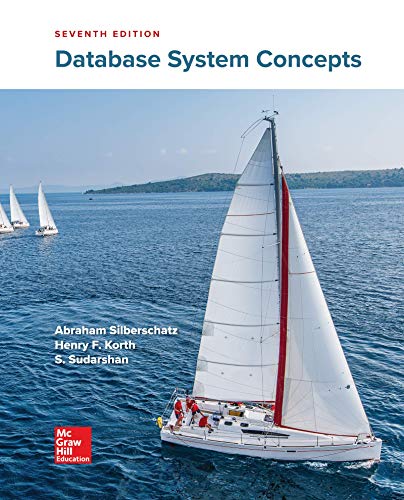
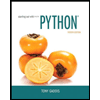
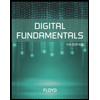
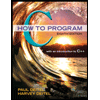
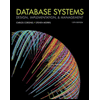
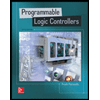