Explain the functionality of each line of code in the following C# script- using System.Collections; using System.Collections.Generic; using UnityEngine; public class Movement2D : MonoBehaviour { public float moveSpeed = 3f; public float jumpForce = 5f; public bool isGrounded; private bool doubleJump; private bool canDash = true; private bool isDashing; private float dashingPower = 24f; private float dashingTime = .2f; private float dashingCooldown = 1f; private bool isWallJumping; private float wallJumpingDirection; private float wallJumpingTime; private float wallJumpingCounter; private float wallJumpingDuration = .04f; private Vector2 wallJumpingPower = new Vector2(8f, 16f); [SerializeField] private Rigidbody2D rb; [SerializeField] private TrailRenderer tr; [SerializeField] private Transform wallCheck; [SerializeField] private LayerMask wallLayer; private SpriteRenderer sprite; public void Start() { sprite = gameObject.GetComponent(); } private void Update() { if (isDashing) { return; } WallJump(); Jump(); Vector3 movement = new Vector3(Input.GetAxis("Horizontal"), 0f, 0f); transform.position += movement * Time.deltaTime * moveSpeed; if (Input.GetAxisRaw("Horizontal") < 0) { sprite.flipX = true; } else if (Input.GetAxisRaw("Horizontal") > 0) { sprite.flipX = false; } if (Input.GetKeyDown(KeyCode.LeftShift) && canDash) { StartCoroutine(Dash()); } if (!isWallJumping) { rb.velocity = new Vector2(Input.GetAxisRaw("Horizontal") * moveSpeed, rb.velocity.y); } } void Jump() { if (Input.GetButtonDown("Jump") && isGrounded) { gameObject.GetComponent().velocity = (new Vector2(0f, jumpForce)); doubleJump = true; } else if (Input.GetButtonDown("Jump") && doubleJump) { gameObject.GetComponent().velocity = (new Vector2(0f, jumpForce)); doubleJump = false; } } private IEnumerator Dash() { canDash = false; isDashing = true; float orignialGravity = rb.gravityScale; rb.gravityScale = 0f; rb.velocity = new Vector3(Input.GetAxis("Horizontal") * dashingPower, 0f); tr.emitting = true; yield return new WaitForSeconds(dashingTime); tr.emitting = false; rb.gravityScale = orignialGravity; isDashing = false; yield return new WaitForSeconds(dashingCooldown); canDash = true; } private void WallJump() { if (Input.GetButtonDown("Jump") && wallJumpingCounter > 0f) { isWallJumping = true; rb.velocity = new Vector2(wallJumpingDirection * wallJumpingPower.x, wallJumpingPower.y); wallJumpingCounter = 0f; if (Input.GetAxisRaw("Horizontal") != wallJumpingDirection) { sprite.flipX = !sprite.flipX; } Invoke(nameof(StopWallJumping), wallJumpingDuration); } } private void StopWallJumping() { isWallJumping = false; } }
Explain the functionality of each line of code in the following C# script-
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Movement2D : MonoBehaviour
{
public float moveSpeed = 3f;
public float jumpForce = 5f;
public bool isGrounded;
private bool doubleJump;
private bool canDash = true;
private bool isDashing;
private float dashingPower = 24f;
private float dashingTime = .2f;
private float dashingCooldown = 1f;
private bool isWallJumping;
private float wallJumpingDirection;
private float wallJumpingTime;
private float wallJumpingCounter;
private float wallJumpingDuration = .04f;
private Vector2 wallJumpingPower = new Vector2(8f, 16f);
[SerializeField] private Rigidbody2D rb;
[SerializeField] private TrailRenderer tr;
[SerializeField] private Transform wallCheck;
[SerializeField] private LayerMask wallLayer;
private SpriteRenderer sprite;
public void Start()
{
sprite = gameObject.GetComponent<SpriteRenderer>();
}
private void Update()
{
if (isDashing)
{
return;
}
WallJump();
Jump();
Vector3 movement = new Vector3(Input.GetAxis("Horizontal"), 0f, 0f);
transform.position += movement * Time.deltaTime * moveSpeed;
if (Input.GetAxisRaw("Horizontal") < 0)
{
sprite.flipX = true;
}
else if (Input.GetAxisRaw("Horizontal") > 0)
{
sprite.flipX = false;
}
if (Input.GetKeyDown(KeyCode.LeftShift) && canDash)
{
StartCoroutine(Dash());
}
if (!isWallJumping)
{
rb.velocity = new Vector2(Input.GetAxisRaw("Horizontal") * moveSpeed, rb.velocity.y);
}
}
void Jump()
{
if (Input.GetButtonDown("Jump") && isGrounded)
{
gameObject.GetComponent<Rigidbody2D>().velocity = (new Vector2(0f, jumpForce));
doubleJump = true;
}
else if (Input.GetButtonDown("Jump") && doubleJump)
{
gameObject.GetComponent<Rigidbody2D>().velocity = (new Vector2(0f, jumpForce));
doubleJump = false;
}
}
private IEnumerator Dash()
{
canDash = false;
isDashing = true;
float orignialGravity = rb.gravityScale;
rb.gravityScale = 0f;
rb.velocity = new Vector3(Input.GetAxis("Horizontal") * dashingPower, 0f);
tr.emitting = true;
yield return new WaitForSeconds(dashingTime);
tr.emitting = false;
rb.gravityScale = orignialGravity;
isDashing = false;
yield return new WaitForSeconds(dashingCooldown);
canDash = true;
}
private void WallJump()
{
if (Input.GetButtonDown("Jump") && wallJumpingCounter > 0f)
{
isWallJumping = true;
rb.velocity = new Vector2(wallJumpingDirection * wallJumpingPower.x, wallJumpingPower.y);
wallJumpingCounter = 0f;
if (Input.GetAxisRaw("Horizontal") != wallJumpingDirection)
{
sprite.flipX = !sprite.flipX;
}
Invoke(nameof(StopWallJumping), wallJumpingDuration);
}
}
private void StopWallJumping()
{
isWallJumping = false;
}
}

It is possible to design, produce, and deploy interactive experiences across a variety of platforms using Unity, a strong and well-liked game creation platform. It is renowned for its adaptability, supporting simulations, virtual reality, augmented reality, and 3D and 2D game production. For developers and artists, Unity's user-friendly interface and wide selection of assets make it available, and its powerful scripting features enable the creation of unique game logic.
Step by step
Solved in 3 steps

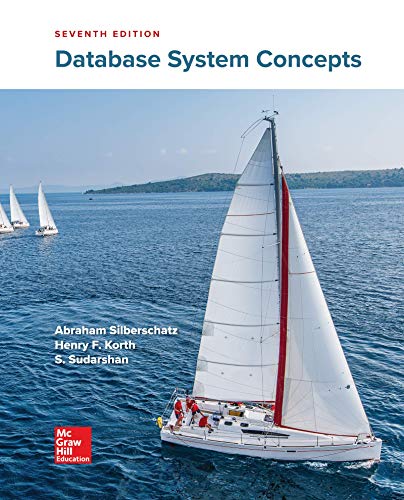
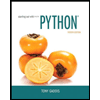
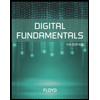
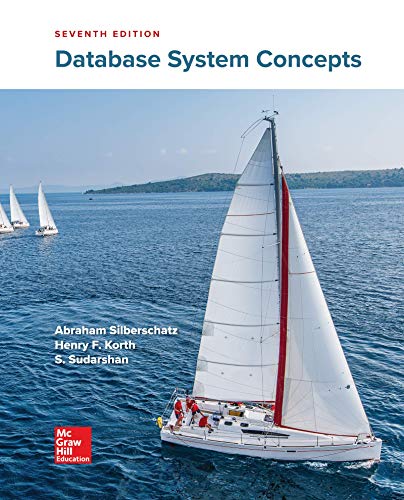
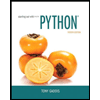
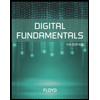
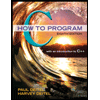
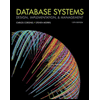
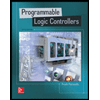